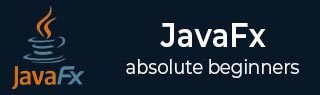
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - TreeView
The TreeView is a graphical user interface component that displays a hierarchical structure of items. It consists of a root node and any number of child nodes. Primarily, the tree view is used for organizing data with hierarchy. It provides a better understanding of data and its relation with other components. In the below figure, we can see a tree view of components.
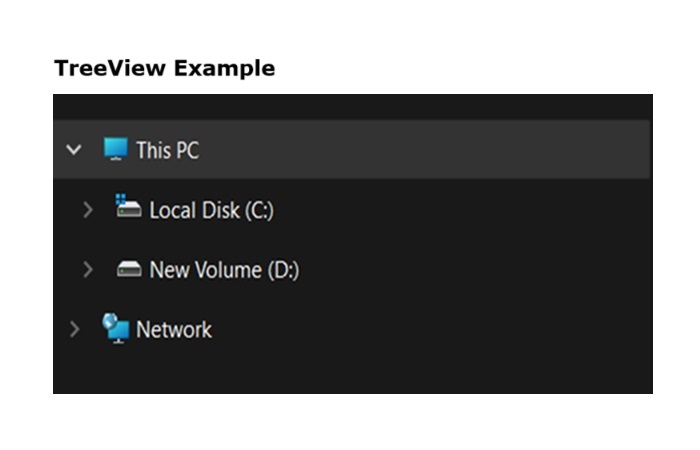
TreeView in JavaFX
In JavaFX, the treeview is represented by a class named TreeView. This class belongs to the package javafx.scene.control. By instantiating this class, we can create a treeview in JavaFX. Constructors of the TreeView class are listed below −
TreeView() − It is the default constructor that constructs an empty treeview.
TreeView(TreeItem rootNode) − It creates a new treeview with the specified root node.
How to create a TreeView in JavaFX?
Follow the steps given below to create a tree view in JavaFX.
Step 1: Create the root node of TreeView
First, create containers for the lists of all items. In this case, root node is the container as it holds all the child nodes. To create the root node, we use the TreeItem class which is the part of javafx.scene.control package. Pass the name of root node to the constructor of this class as a parameter value. −
// Creating the root node TreeItem<String> root = new TreeItem<>("Smartphones");
Step 2: Create the child nodes of TreeView
Again, use the TreeItem class to create child nodes. Once the child nodes are created, use the getChildren() method along with addAll() to add the required items to it.
TreeItem<String> ios = new TreeItem<>("iOS"); ios.getChildren().addAll( new TreeItem<>("iPhone 15 Plus"), new TreeItem<>("iPhone 14") );
Step 3: Add the child nodes to root nodes
To add the child nodes to the root node, pass the name of child nodes as a parameter value to the addAll() method as shown in the following code blocks −
// Add the child nodes to the root node root.getChildren().addAll(android, ios);
Step 4: Instantiate the TreeView class
To create tree view, instantiate the TreeView class and pass the root node to its constructor as a parameter value as shown in the below code −
// Create the TreeView and add the root node TreeView<String> treesV = new TreeView<>(root);
Step 5: Launching Application
Once the tree view is created, follow the given steps below to launch the application properly −
Firstly, instantiate the class named Scene by passing the TreeView object as a parameter value to its constructor. We can also pass the dimensions of the application screen as optional parameters to this constructor.
Then, set the title to the stage using the setTitle() method of the Stage class.
Now, a Scene object is added to the stage using the setScene() method of the class named Stage.
Display the contents of the scene using the method named show().
Lastly, the application is launched with the help of the launch() method.
Example
Following is the program that will create a TreeView using JavaFX. Save this code in a file with the name JavafxTreeview.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.TreeItem; import javafx.scene.control.TreeView; import javafx.stage.Stage; public class JavafxTreeview extends Application { @Override public void start(Stage stage) throws Exception { // Creating the root node TreeItem<String> root = new TreeItem<>("Smartphones"); root.setExpanded(true); // Creating its child nodes TreeItem<String> android = new TreeItem<>("Android"); android.getChildren().addAll( new TreeItem<>("Samsung Galaxy S23 Ultra"), new TreeItem<>("Xiaomi Redmi Note 13 Pro"), new TreeItem<>("OnePlus 11R") ); TreeItem<String> ios = new TreeItem<>("iOS"); ios.getChildren().addAll( new TreeItem<>("iPhone 15 Plus"), new TreeItem<>("iPhone 14") ); // Add the child nodes to the root node root.getChildren().addAll(android, ios); // Create the TreeView and add the root node TreeView<String> treesV = new TreeView<>(root); // Create a scene and add the TreeView Scene scene = new Scene(treesV, 400, 300); stage.setTitle("TreeView in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTreeview.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTreeview
Output
On executing, the above program generates a JavaFX window displaying a TreeView with a list of smartphones as shown below.
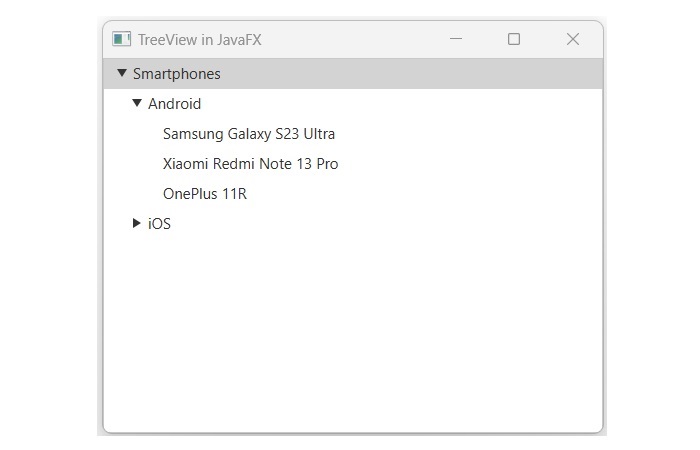
Setting the mouse events for TreeView
If we want to show which items are getting clicked by users, then, we can use setOnMouseClicked() method by passing a lambda expression as shown in the below JavaFX code. Save this code in a file with the name JavafxTreeview.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.TreeItem; import javafx.scene.control.TreeView; import javafx.stage.Stage; public class JavafxTreeview extends Application { @Override public void start(Stage stage) throws Exception { // Creating the root node TreeItem<String> root = new TreeItem<>("Smartphones"); root.setExpanded(true); // Creating its child nodes TreeItem<String> android = new TreeItem<>("Android"); android.getChildren().addAll( new TreeItem<>("Samsung Galaxy S23 Ultra"), new TreeItem<>("Xiaomi Redmi Note 13 Pro"), new TreeItem<>("OnePlus 11R") ); TreeItem<String> ios = new TreeItem<>("iOS"); ios.getChildren().addAll( new TreeItem<>("iPhone 15 Plus"), new TreeItem<>("iPhone 14") ); // Add the child nodes to the root node root.getChildren().addAll(android, ios); // Create the TreeView and add the root node TreeView<String> treesV = new TreeView<>(root); // Handle mouse clicks on the nodes treesV.setOnMouseClicked(event -> { // Get the selected item TreeItem<String> item = treesV.getSelectionModel().getSelectedItem(); if (item != null) { // Display the item text System.out.println(item.getValue()); } }); // Create a scene and add the TreeView Scene scene = new Scene(treesV, 400, 300); stage.setTitle("TreeView in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTreeview.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTreeview
Output
On executing the above code, it will generate the following output. When we click on the items, their name will be displayed on the console.
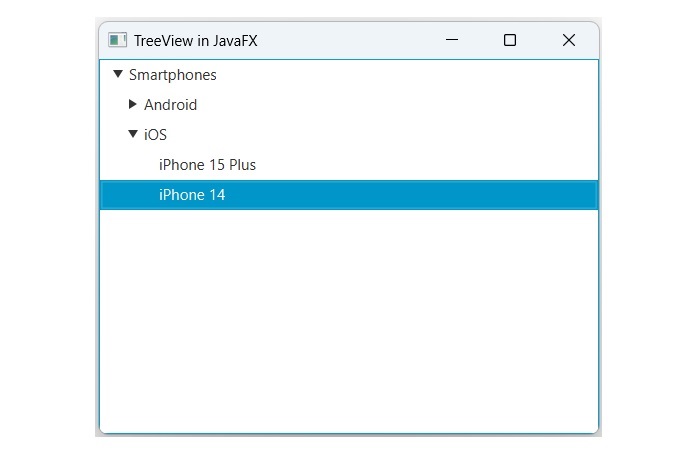