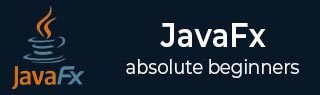
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Stroke Width Property
In the previous chapters, we have learned about various 2D shapes and how they are drawn in a JavaFX application. However, it is necessary to make your application as attractive as possible for better user experience. This also includes enhancing the look and feel of your 2D shapes within your JavaFX application.
JavaFX provides a set of properties for this purpose. They are used to improve the quality of your shapes on the application. In this chapter, we will learn about Stroke Width property in detail.
Stroke Width Property
The Stroke Width Property is used to set thickness of the boundary line of a 2D shape. This property is of the type double and it represents the width of the boundary line of the shape. You can set the stroke width using the method setStrokeWidth() as follows −
Path.setStrokeWidth(3.0)
By default, the value of the stroke with of a shape is 1.0. Following is a diagram of a triangle with different values of stroke width.
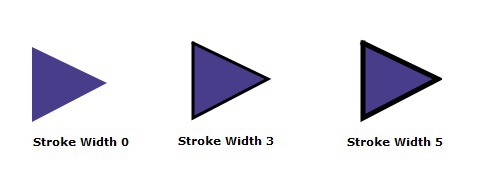
Example
In this example, we will try to create a 2D shape, say a circle, and set a value to its stroke width. Save this file with the name StrokeWidthExample.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Circle; import javafx.scene.shape.Shape; import javafx.scene.paint.Color; import javafx.stage.Stage; public class StrokeWidthExample extends Application { @Override public void start(Stage stage) { //Creating a Circle Circle circle = new Circle(150.0f, 150.0f, 50.0f); circle.setFill(Color.WHITE); circle.setStroke(Color.BLACK); circle.setStrokeWidth(6.0); //Creating a Group object Group root = new Group(circle); //Creating a scene object Scene scene = new Scene(root, 300, 300); //Setting title to the Stage stage.setTitle("Drawing a Circle"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeWidthExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeWidthExample
Output
On executing, the above program generates a JavaFX window displaying a circle with a stroke width as shown below.
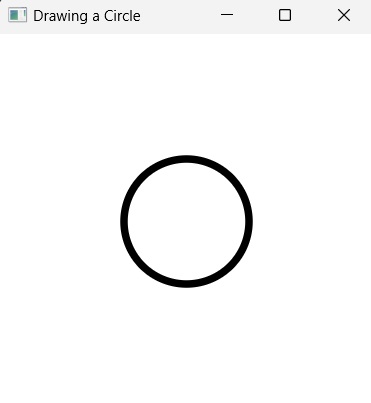
Example
Now, let us try to draw a Triangle shape with relatively larger width and observe the results. Save this file with the name StrokeWidthTriangle.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Polygon; import javafx.scene.shape.Shape; import javafx.scene.paint.Color; import javafx.stage.Stage; public class StrokeWidthTriangle extends Application { @Override public void start(Stage stage) { //Creating a Triangle Polygon triangle = new Polygon(); //Adding coordinates to the polygon triangle.getPoints().addAll(new Double[]{ 150.0, 150.0, 220.0, 175.0, 150.0, 200.0, }); triangle.setFill(Color.WHITE); triangle.setStroke(Color.BLACK); triangle.setStrokeWidth(100.0); //Creating a Group object Group root = new Group(triangle); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Triangle"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeWidthTriangle.java java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeWidthTriangle
Output
On executing, the above program generates a JavaFX window displaying a triangle with a relatively larger stroke width as shown below.
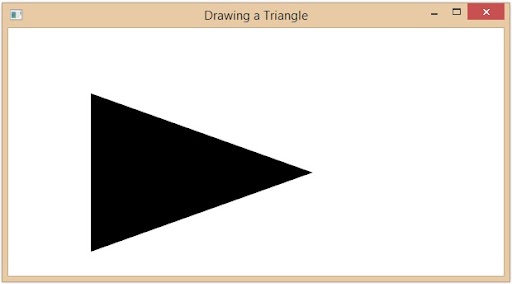