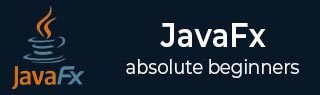
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Animations
In general, animating an object implies creating illusion of its motion by rapid display. In JavaFX, a node can be animated by changing its property over time. JavaFX provides a package named javafx.animation. This package contains classes that are used to animate the nodes. Animation is the base class of all these classes.
Using JavaFX, you can apply animations (transitions) such as Fade Transition, Fill Transition, Rotate Transition, Scale Transition, Stroke Transition, Translate Transition, Path Transition, Sequential Transition, Pause Transition, Parallel Transition, etc.
All these transitions are represented by individual classes in the package javafx.animation.
To apply a particular animation to a node, you have to follow the steps given below −
Create a require node using respective class.
Instantiate the respective transition (animation) class that is to be applied
Set the properties of the transition and
Finally play the transition using the play() method of the Animation class.
In this chapter we are going to discuss examples of basic transitions(Rotation, Scaling, Translation).
Rotate Transition
Following is the program which demonstrates Rotate Transition in JavaFX. Save this code in a file with the name RotateTransitionExample.java.
import javafx.animation.RotateTransition; import javafx.application.Application; import static javafx.application.Application.launch; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Polygon; import javafx.stage.Stage; import javafx.util.Duration; public class RotateTransitionExample extends Application { @Override public void start(Stage stage) { //Creating a hexagon Polygon hexagon = new Polygon(); //Adding coordinates to the hexagon hexagon.getPoints().addAll(new Double[]{ 200.0, 50.0, 400.0, 50.0, 450.0, 150.0, 400.0, 250.0, 200.0, 250.0, 150.0, 150.0, }); //Setting the fill color for the hexagon hexagon.setFill(Color.BLUE); //Creating a rotate transition RotateTransition rotateTransition = new RotateTransition(); //Setting the duration for the transition rotateTransition.setDuration(Duration.millis(1000)); //Setting the node for the transition rotateTransition.setNode(hexagon); //Setting the angle of the rotation rotateTransition.setByAngle(360); //Setting the cycle count for the transition rotateTransition.setCycleCount(50); //Setting auto reverse value to false rotateTransition.setAutoReverse(false); //Playing the animation rotateTransition.play(); //Creating a Group object Group root = new Group(hexagon); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Rotate transition example "); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac RotateTransitionExample.java java RotateTransitionExample
On executing, the above program generates a JavaFX window as shown below.
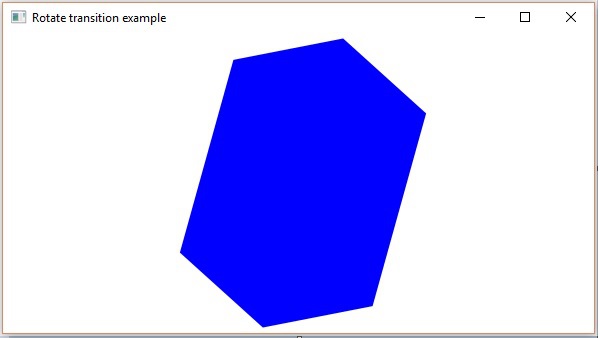
Scale Transition
Following is the program which demonstrates Scale Transition in JavaFX. Save this code in a file with the name ScaleTransitionExample.java.
import javafx.animation.ScaleTransition; import javafx.application.Application; import static javafx.application.Application.launch; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.stage.Stage; import javafx.util.Duration; public class ScaleTransitionExample extends Application { @Override public void start(Stage stage) { //Drawing a Circle Circle circle = new Circle(); //Setting the position of the circle circle.setCenterX(300.0f); circle.setCenterY(135.0f); //Setting the radius of the circle circle.setRadius(50.0f); //Setting the color of the circle circle.setFill(Color.BROWN); //Setting the stroke width of the circle circle.setStrokeWidth(20); //Creating scale Transition ScaleTransition scaleTransition = new ScaleTransition(); //Setting the duration for the transition scaleTransition.setDuration(Duration.millis(1000)); //Setting the node for the transition scaleTransition.setNode(circle); //Setting the dimensions for scaling scaleTransition.setByY(1.5); scaleTransition.setByX(1.5); //Setting the cycle count for the translation scaleTransition.setCycleCount(50); //Setting auto reverse value to true scaleTransition.setAutoReverse(false); //Playing the animation scaleTransition.play(); //Creating a Group object Group root = new Group(circle); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Scale transition example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac ScaleTransitionExample.java java ScaleTransitionExample
On executing, the above program generates a JavaFX window as shown below.
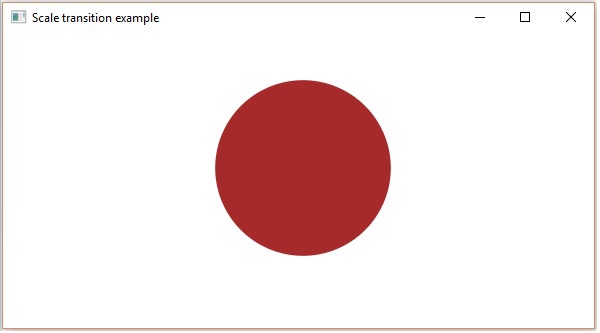
Translate Transition
Following is the program which demonstrates Translate Transition in JavaFX. Save this code in a file with the name TranslateTransitionExample.java.
import javafx.animation.TranslateTransition; import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.stage.Stage; import javafx.util.Duration; public class TranslateTransitionExample extends Application { @Override public void start(Stage stage) { //Drawing a Circle Circle circle = new Circle(); //Setting the position of the circle circle.setCenterX(150.0f); circle.setCenterY(135.0f); //Setting the radius of the circle circle.setRadius(100.0f); //Setting the color of the circle circle.setFill(Color.BROWN); //Setting the stroke width of the circle circle.setStrokeWidth(20); //Creating Translate Transition TranslateTransition translateTransition = new TranslateTransition(); //Setting the duration of the transition translateTransition.setDuration(Duration.millis(1000)); //Setting the node for the transition translateTransition.setNode(circle); //Setting the value of the transition along the x axis. translateTransition.setByX(300); //Setting the cycle count for the transition translateTransition.setCycleCount(50); //Setting auto reverse value to false translateTransition.setAutoReverse(false); //Playing the animation translateTransition.play(); //Creating a Group object Group root = new Group(circle); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Translate transition example"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac TranslateTransitionExample.java java TranslateTransitionExample
On executing, the above program generates a JavaFX window as shown below.

In addition to these, JavaFX provides classes to apply more transitions on nodes. The following are the other kinds of transitions supported by JavaFX.
Transitions that effects the attributes of the nodes Fade, Fill, Stroke
Transition that involve more than one basic transitions Sequential, Parallel, Pause
Transition that translate the object along the specified path Path Transition