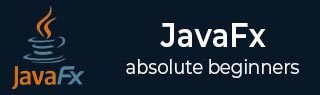
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Environment
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Path Objects
- JavaFX - Path Objects
- JavaFX - LineTo Path Object
- JavaFX - HLineTo Path Object
- JavaFX - VLineTo Path Object
- JavaFX - QuadCurveTo Path Object
- JavaFX - CubicCurveTo Path Object
- JavaFX - ArcTo Path Object
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX - Linear Gradient Pattern
- JavaFX - Radial Gradient Pattern
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX - Color Adjust Effect
- JavaFX - Color input Effect
- JavaFX - Image Input Effect
- JavaFX - Blend Effect
- JavaFX - Bloom Effect
- JavaFX - Glow Effect
- JavaFX - Box Blur Effect
- JavaFX - GaussianBlur Effect
- JavaFX - MotionBlur Effect
- JavaFX - Reflection Effect
- JavaFX - SepiaTone Effect
- JavaFX - Shadow Effect
- JavaFX - DropShadow Effect
- JavaFX - InnerShadow Effect
- JavaFX - Lighting Effect
- JavaFX - Light.Distant Effect
- JavaFX - Light.Spot Effect
- JavaFX - Point.Spot Effect
- JavaFX - DisplacementMap
- JavaFX - PerspectiveTransform
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX - Rotation Transformation
- JavaFX - Scaling Transformation
- JavaFX - Translation Transformation
- JavaFX - Shearing Transformation
- JavaFX Animations
- JavaFX - Animations
- JavaFX - Rotate Transition
- JavaFX - Scale Transition
- JavaFX - Translate Transition
- JavaFX - Fade Transition
- JavaFX - Fill Transition
- JavaFX - Stroke Transition
- JavaFX - Sequential Transition
- JavaFX - Parallel Transition
- JavaFX - Pause Transition
- JavaFX - Path Transition
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX Effects - Box Blur
In general, Blur means becoming unclear, on applying blur effect to a node it is made unclear. Box Blur is a kind of blur effect provided by JavaFX. In this effect, to apply blur to node, a simple box filter is used.
The class named BoxBlur of the package javafx.scene.effect represents the BoxBlur effect, this class contains four properties, which are −
height − This property is of double type representing the vertical size of the effect.
width − This property is of double type representing the horizontal size of the effect.
input − This property is of the type effect and it represents an input to the BoxBlur effect.
iterations − This property is of an integer type representing the number of iterations of the effect, which are to be applied on the node. This is done to improve its quality or smoothness.
Example
Following is an example demonstrating the box blur effect. In here, we are drawing the text “Welcome to Tutorialspoint” filled with DARKSEAGREEN color and applying the Box Blur effect to it.
Save this code in a file with the name BoxBlurEffectExample.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.effect.BoxBlur; import javafx.scene.paint.Color; import javafx.stage.Stage; import javafx.scene.text.Font; import javafx.scene.text.FontWeight; import javafx.scene.text.Text; public class BoxBlurEffectExample extends Application { @Override public void start(Stage stage) { //Creating a Text object Text text = new Text(); //Setting font to the text text.setFont(Font.font(null, FontWeight.BOLD, 40)); //setting the position of the text text.setX(60); text.setY(150); //Setting the text to be added. text.setText("Welcome to Tutorialspoint"); //Setting the color of the text text.setFill(Color.DARKSEAGREEN); //Instantiating the BoxBlur class BoxBlur boxblur = new BoxBlur(); //Setting the width of the box filter boxblur.setWidth(8.0f); //Setting the height of the box filter boxblur.setHeight(3.0f); //Setting the no of iterations boxblur.setIterations(3); //Applying BoxBlur effect to the text text.setEffect(boxblur); //Creating a Group object Group root = new Group(text); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Sample Application"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac BoxBlurEffectExample.java java BoxBlurEffectExample
On executing, the above program generates a JavaFX window as shown below.
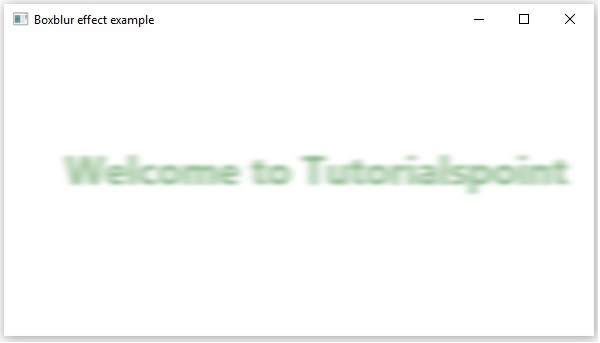
To Continue Learning Please Login