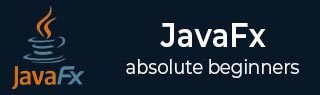
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - SplitPane
The splitpane is a control with two or more slides separated by dividers. Each slide provides a different viewport allowing us to embed multiple components. We can also set the orientation of the splitpane to be horizontal or vertical. Let's have a look at what a typical splitpane looks like −
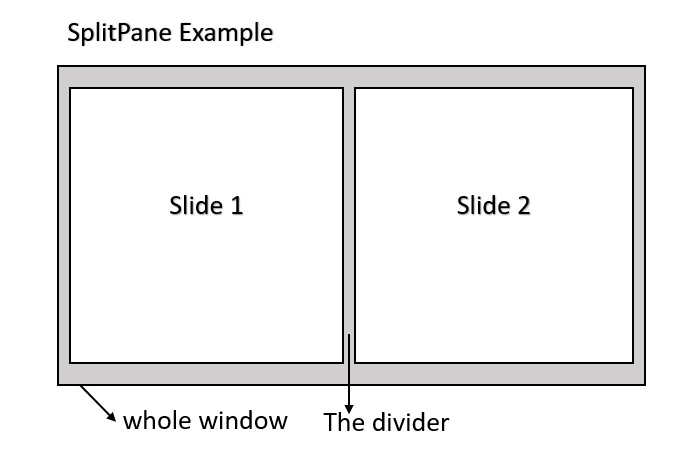
SplitPane in JavaFX
In JavaFX, the class named SplitPane represents a splitpane. To use the feature of spiltpane, we need to create an instance of the SplitPane class and add the components we want to display inside it. These components can be any JavaFX nodes, such as labels, buttons, images, text fields and so on. We can use any of the below constructors to create a splitpane −
SplitPane() − It is the default constructor used to create a splitpane without any predefined nodes.
SplitPane(Node components) − It is the parameterized constructor of SplitPane class which will create a new splitpane with the specified nodes.
Steps to create a splitpane in JavaFX
To create a splitpane in JavaFX, follow the steps given below.
Step 1: Create two or more Nodes
As discussed earlier, a splitpane contains at least two slides. Hence, our first step would be creating two or more nodes to display within these distinct slides. For the sake of this example, we are going to use the labels. In JavaFX, the labels are created by instantiating the class named Label which belongs to a package javafx.scene.control. Create the labels as shown below −
// Creating four labels Label labelOne = new Label("Label 1"); Label labelTwo = new Label("Label 2"); Label labelThree = new Label("Label 3"); Label labelFour = new Label("Label 4");
Similarly, create the desired nodes by instantiating their respective classes.
Step 2: Instantiate the SplitPane class
Instantiate the SplitPane class of package javafx.scene.control without passing any parameter value to its constructor and add all the labels to the splitpane using the getItems() method.
// instantiating the SplitPane class SplitPane splitP = new SplitPane(); // adding the labels to the SplitPane splitP.getItems().addAll(labelOne, labelTwo, labelThree, labelFour);
Step 3: Launching Application
After creating the SplitPane and adding the labels to it, follow the given steps below to launch the application properly −
Firstly, instantiate the class named Scene by passing the SplitPane object as a parameter value to its constructor. Also, pass dimensions of the application screen as optional parameters to this constructor.
Then, set the title to the stage using the setTitle() method of the Stage class.
Now, a Scene object is added to the stage using the setScene() method of the class named Stage.
Display the contents of the scene using the method named show().
Lastly, the application is launched with the help of the launch() method.
Example
Following is the program which will create a splitpane in JavaFX. Save this code in a file with the name NewSplitpane.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.SplitPane; import javafx.stage.Stage; public class NewSplitpane extends Application { @Override public void start(Stage stage) { // Creating four labels Label labelOne = new Label("Label 1"); Label labelTwo = new Label("Label 2"); Label labelThree = new Label("Label 3"); Label labelFour = new Label("Label 4"); // instantiating the SplitPane class SplitPane splitP = new SplitPane(); // adding the labels to the SplitPane splitP.getItems().addAll(labelOne, labelTwo, labelThree, labelFour); // Creating a Scene with the SplitPane as its root node Scene scene = new Scene(splitP, 400, 300); // to set the title stage.setTitle("SplitPane in JavaFX"); // Setting the Scene of the Stage stage.setScene(scene); // Display the Stage stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the above Java file using the command prompt with the help of following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls NewSplitpane.java java --module-path %PATH_TO_FX% --add-modules javafx.controls NewSplitpane
Output
On executing, the above program generates a JavaFX window displaying a SplitPane with four labels and three dividers as shown below.
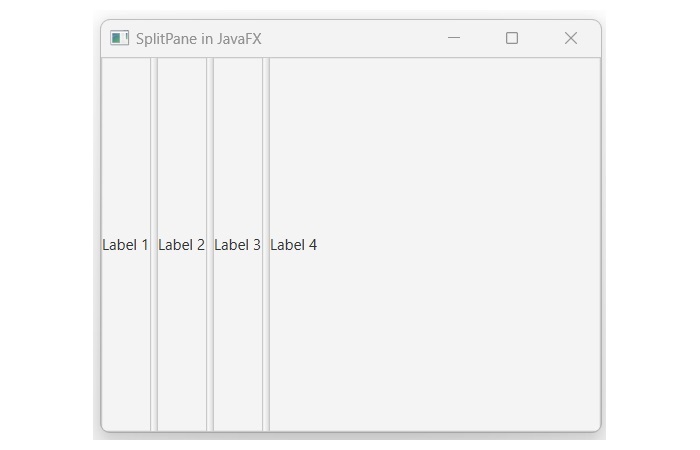
Setting the Orientation of the SplitPane
By default, the SplitPane has a horizontal orientation, meaning that the components are placed next to each other from left to right. We can change the orientation to vertical by calling the setOrientation() method of the SplitPane class and passing an Orientation.VERTICAL argument.
Example
In the following JavaFX program, we will create a vertical SplitPane. Save this code in a file with the name SplitpaneDemo.java.
import javafx.application.Application; import javafx.geometry.Orientation; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.SplitPane; import javafx.stage.Stage; import javafx.scene.layout.VBox; public class SplitpaneDemo extends Application { @Override public void start(Stage stage) { // Create a SplitPane with vertical orientation SplitPane splitP = new SplitPane(); splitP.setOrientation(Orientation.VERTICAL); // vertical box to hold the labels VBox box1 = new VBox(); VBox box2 = new VBox(); // Create two labels and add them to the SplitPane box1.getChildren().add(new Label("This is \nthe \nfirst section")); box2.getChildren().add(new Label("This is \nthe \nsecond section")); splitP.getItems().addAll(box1, box2); // Set the divider position to 50 splitP.setDividerPositions(0.5); // Create a scene and show the stage Scene scene = new Scene(splitP, 400, 300); stage.setTitle("SplitPane in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls SplitpaneDemo.java java --module-path %PATH_TO_FX% --add-modules javafx.controls SplitpaneDemo
Output
When we execute the above code, it will generate the following output.
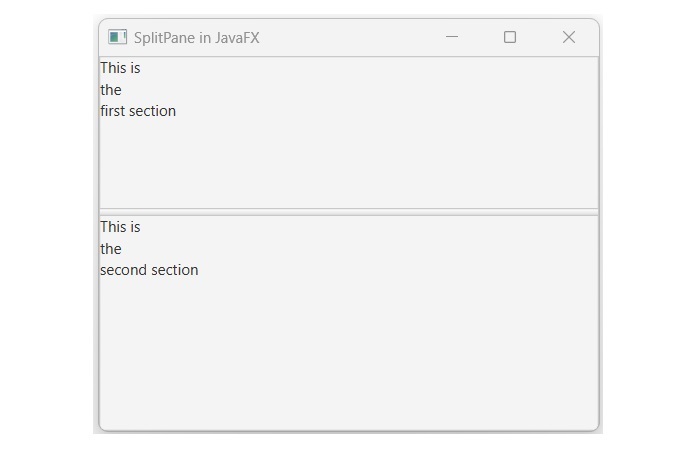