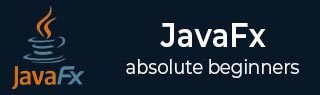
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Textflow Layout
TextFlow Layout in JavaFX
TextFlow is a layout that allows us to set multiple text nodes in a single flow, and adjust their position and alignment according to the font, width, and line spacing of the TextFlow. It can also embed objects, such as images or shapes, that can be inserted in the text content. The class named textFlow of the package javafx.scene.layout represents the text flow. To create a TextFlow, we can use one of its constructors listed below −
TextFlow() − Creates an empty TextFlow.
TextFlow(Node childNodes) − Creates a TextFlow with the given nodes as children.
To customize the appearance and behavior of the TextFlow, this class provides two properties which are as follows −
lineSpacing − This property is of double type and it is used to define the space between the text objects. You can set this property using the method named setLineSpacing().
textAlignment − This property represents the alignment of the text objects in the pane. You can set value to this property using the method setTextAlignment(). To this method you can pass four values: CENTER, JUSTIFY, LEFT, RIGHT.
Example
The following program is an example of the text flow layout. In this, we are creating three text objects with font 15. Save this code in a file with the name TextFlowExample.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.text.Font; import javafx.scene.text.Text; import javafx.scene.text.TextAlignment; import javafx.scene.text.TextFlow; import javafx.stage.Stage; public class TextFlowExample extends Application { @Override public void start(Stage stage) { //Creating text objects Text text1 = new Text("Welcome to Tutorialspoint "); //Setting font to the text text1.setFont(new Font(15)); //Setting color to the text text1.setFill(Color.DARKSLATEBLUE); Text text2 = new Text("We provide free tutorials for readers in various technologies "); //Setting font to the text text2.setFont(new Font(15)); //Setting color to the text text2.setFill(Color.DARKGOLDENROD); Text text3 = new Text("\n Recently we started free video tutorials too "); //Setting font to the text text3.setFont(new Font(15)); //Setting color to the text text3.setFill(Color.DARKGRAY); Text text4 = new Text("We believe in easy learning"); //Setting font to the text text4.setFont(new Font(15)); text4.setFill(Color.MEDIUMVIOLETRED); //Creating the text flow plane TextFlow textFlowPane = new TextFlow(); //Setting the line spacing between the text objects textFlowPane.setTextAlignment(TextAlignment.JUSTIFY); //Setting the width textFlowPane.setPrefSize(600, 300); //Setting the line spacing textFlowPane.setLineSpacing(5.0); //Adding cylinder to the pane textFlowPane.getChildren().addAll(text1, text2, text3, text4); //Creating a scene object Scene scene = new Scene(textFlowPane, 400, 300); //Setting title to the Stage stage.setTitle("Textflow Layout in JavaFX"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls TextFlowExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls TextflowExample
Output
On executing, the above program generates a JavaFX window as shown below.
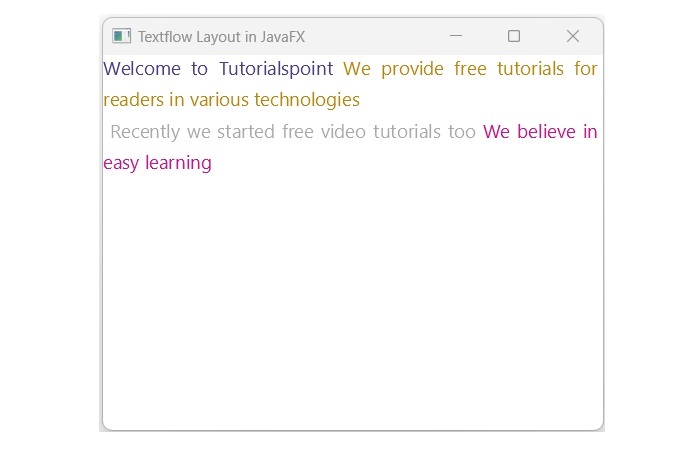
Adding Images to the TextFlow
It is also possible to embed image within the TextFlow. This is achieved by using the Image and ImageView class of the JavaFX. We simply need to instantiate these classes and pass the ImageView object to the constructor of TextFlow class as shown in the below example. Save this code in a Java file with the name JavafxTextflow.java.
Example
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.layout.VBox; import javafx.scene.paint.Color; import javafx.scene.text.Font; import javafx.scene.text.FontPosture; import javafx.scene.text.FontWeight; import javafx.scene.text.Text; import javafx.scene.text.TextAlignment; import javafx.scene.text.TextFlow; import javafx.stage.Stage; public class JavafxTextflow extends Application { @Override public void start(Stage stage) { // Create some text nodes with different styles Text text1 = new Text("Hello"); text1.setFill(Color.RED); text1.setFont(Font.font("Arial", FontWeight.BOLD, 20)); Text text2 = new Text("JavaFX"); text2.setFill(Color.BLUE); text2.setFont(Font.font("Arial", FontPosture.ITALIC, 20)); Text text3 = new Text("\nThis is a "); text3.setFill(Color.BLACK); text3.setFont(Font.font("Arial", 16)); Text text4 = new Text("TextFlow"); text4.setFill(Color.GREEN); text4.setFont(Font.font("Arial", FontWeight.BOLD, FontPosture.ITALIC, 16)); Text text5 = new Text(" example."); text5.setFill(Color.BLACK); text5.setFont(Font.font("Arial", 16)); // Create an image node Image image = new Image("faviconTP.png"); ImageView imageView = new ImageView(image); imageView.setFitWidth(100); imageView.setPreserveRatio(true); // Create a text flow with the text nodes and the image node TextFlow textFlow = new TextFlow(text1, text2, text3, text4, text5, imageView); // Set some properties of the text flow textFlow.setLineSpacing(10); textFlow.setTextAlignment(TextAlignment.CENTER); textFlow.setPrefWidth(300); // Create a scene and a stage Scene scene = new Scene(textFlow, 400, 300); stage.setTitle("TextFlow Example"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved java file from the command prompt, use the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTextflow.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTextflow
Output
On executing the above Java code, it will generate a JavaFX window as shown below.
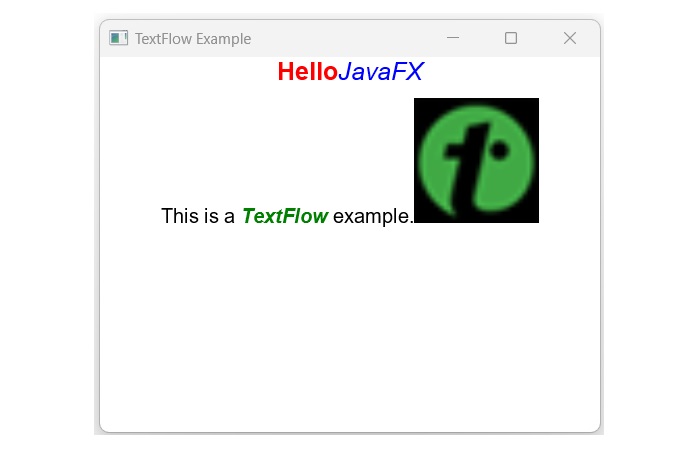