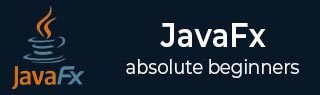
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Stroke Fill Property
JavaFX application supports displaying diverse content like text, 2D shapes, 3D shapes, etc.; and every object has a default setting in order to create a basic application. Hence, while drawing 2D shapes on a JavaFX application, each shape also has some default settings that can be modified when they are set separately. For instance, the default fill colour in a 2D shape is always black.
Various properties are introduced to improve the quality of these shapes; and we have already learned how to change the dimensions and placement of the shape boundaries, in previous chapters. In this chapter, we will learn how to change the default colour black of a specific 2D shape to other colours.
Stroke Fill Property
Stroke Fill property in JavaFX 2D shapes is used to specify the colour which a shape is to be filled with. This property belongs to the javafx.scene.paint package. You can set the fill color of a shape using the method setFill() as follows −
path.setFill(COLOR.BLUE);
By default, the value of the stroke color is BLACK. Following is a diagram of a triangle with different colors.
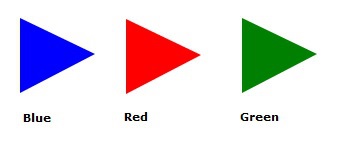
Example
In this example, we will try to create two 2D circles, and fill one of the circle with Yellow colour and another will maintain its default colour. The aim is to observe the difference between both shapes. Save this file with the name StrokeFillExample.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Circle; import javafx.scene.shape.Shape; import javafx.scene.paint.Color; import javafx.stage.Stage; public class StrokeFillExample extends Application { @Override public void start(Stage stage) { //Creating a Circle Circle circle1 = new Circle(200.0f, 150.0f, 50.0f); Circle circle2 = new Circle(100.0f, 150.0f, 50.0f); circle1.setFill(Color.YELLOW); //Creating a Group object Group root = new Group(); root.getChildren().addAll(circle1, circle2); //Creating a scene object Scene scene = new Scene(root, 300, 300); //Setting title to the Stage stage.setTitle("Colouring a Circle"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeFillExample.java java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeFillExample
Output
On executing, the above program generates a JavaFX window displaying two circles, the left one having its default fill while the other one is yellow coloured, as shown below.
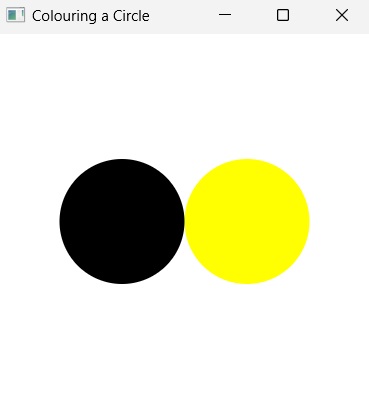
Example
We created two simple 2D shapes in the previous example, but you can also set a fill color to complex shapes created using path element.
In this example, we are trying to fill a complex shape created using line commands of SVG Path. Save this file with the name StrokeFillSVGPath.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.SVGPath; import javafx.stage.Stage; public class StrokeFillSVGPath extends Application { @Override public void start(Stage stage) { //Creating a SVGPath object SVGPath svgPath = new SVGPath(); String path = "M 100 100 H 190 V 190 H 150 L 200 200"; //Setting the SVGPath in the form of string svgPath.setContent(path); // Setting the stroke and fill of the path svgPath.setStroke(Color.BLACK); svgPath.setFill(Color.BLUE); //Creating a Group object Group root = new Group(svgPath); //Creating a scene object Scene scene = new Scene(root, 300, 300); //Setting title to the Stage stage.setTitle("Drawing a Line"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeFillSVGPath.java java --module-path %PATH_TO_FX% --add-modules javafx.controls StrokeFillSVGPath
Output
On executing, the above program generates a JavaFX window displaying two circles, the left one having its default fill while the other one is yellow coloured, as shown below.
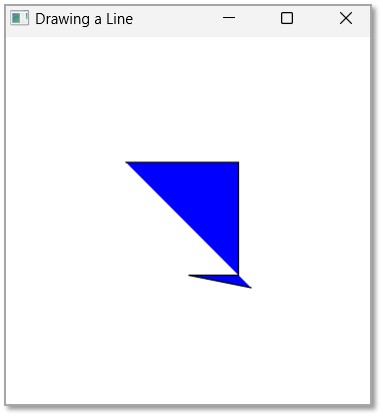