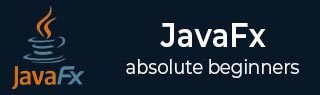
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Drawing Modes Property
You can draw 3D shapes in a JavaFX application using the Shape3D class. This class allows you construct three types of 3D shapes: box, cylinder, sphere as its direct subclasses.
However, according to the nature of a JavaFX application you are trying to create, you can also enhance the look of a 3D shape using the properties the Shape3D class provides. There are three such properties that can be applied on 3D shapes of a JavaFX application. They are listed as follows −
Cull Face Property
Drawing Modes Property
Material Property
In this chapter, we will be learning about the Drawing Modes Property in JavaFX.
Drawing Modes Property
Drawing Modes is the property that belongs to the DrawMode enum type and it represents the type of drawing mode used to draw the current 3D shape. In JavaFX, you can choose two draw modes to draw a 3D shape, which are −
Fill − This mode draws and fills a 2D shape (DrawMode.FILL).
Line − This mode draws a 3D shape using lines (DrawMode.LINE).
By default, the drawing mode of a 3Dimensional shape is fill. But you can still choose the draw mode to draw a 3D shape using the method setDrawMode() as follows −
box.setDrawMode(DrawMode.FILL);
Example
The following program is an example which demonstrates the LINE draw mode on a 3D box. Save this code in a file with the name BoxDrawModeLine.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.Box; import javafx.scene.shape.DrawMode; import javafx.stage.Stage; public class BoxDrawModeLine extends Application { @Override public void start(Stage stage) { //Drawing a Box Box box1 = new Box(); //Setting the properties of the Box box1.setWidth(100.0); box1.setHeight(100.0); box1.setDepth(100.0); //Setting the position of the box box1.setTranslateX(200); box1.setTranslateY(150); box1.setTranslateZ(0); //Setting the drawing mode of the box box1.setDrawMode(DrawMode.LINE); //Creating a Group object Group root = new Group(box1); //Creating a scene object Scene scene = new Scene(root, 300, 300); //Setting title to the Stage stage.setTitle("Drawing a Box"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls BoxDrawModeLine.java java --module-path %PATH_TO_FX% --add-modules javafx.controls BoxDrawModeLine
Output
On executing, the above program generates a JavaFX window displaying a box with draw mode LINE, as follows −
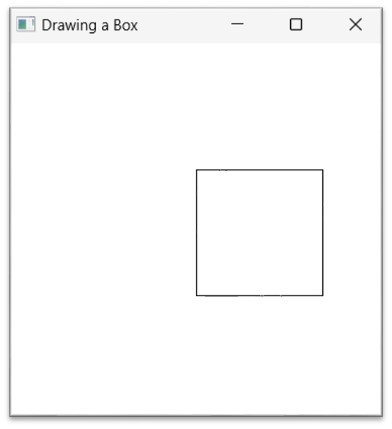
Example
Let us now see another example that shows the usage of FILL draw mode on a 3D shape. To show the difference of these modes properly, we will again apply this mode on a 3D box. Save this code in a file with the name BoxDrawModeFill.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.PerspectiveCamera; import javafx.scene.Scene; import javafx.scene.shape.Box; import javafx.scene.shape.DrawMode; import javafx.stage.Stage; public class BoxDrawModeFill extends Application { @Override public void start(Stage stage) { //Drawing a Box Box box1 = new Box(); //Setting the properties of the Box box1.setWidth(100.0); box1.setHeight(100.0); box1.setDepth(100.0); //Setting the position of the box box1.setTranslateX(200); box1.setTranslateY(150); box1.setTranslateZ(0); //Setting the drawing mode of the box box1.setDrawMode(DrawMode.FILL); //Creating a Group object Group root = new Group(box1); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting camera PerspectiveCamera camera = new PerspectiveCamera(false); camera.setTranslateX(100); camera.setTranslateY(50); camera.setTranslateZ(0); scene.setCamera(camera); //Setting title to the Stage stage.setTitle("Drawing a Box"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls BoxDrawModeFill.java java --module-path %PATH_TO_FX% --add-modules javafx.controls BoxDrawModeFill
Output
On executing, the above program generates a JavaFX window displaying a box with draw mode FILL, as follows −
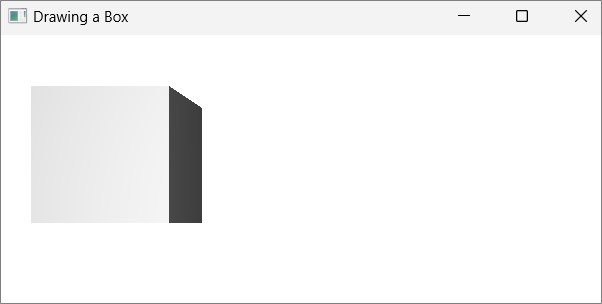