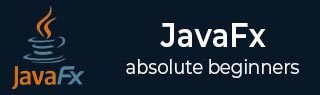
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Cull Face Property
JavaFX also provides various properties for 3D objects. These properties can range from deciding the material of a shape: interior and exterior, rendering the 3D object geometry and culling faces of the 3D shape.
All these properties are offered in order to improvise the look and feel of a 3D object; and check what is suitable for the application and apply them.
In this chapter, let us learn more about the Cull Face Property.
Cull Face Property
In general, culling is the removal of improperly oriented parts of a shape (which are not visible in the view area).
The Cull Face property is of the type CullFace and it represents the Cull Face of a 3D shape. You can set the Cull Face of a shape using the method setCullFace() as shown below −
box.setCullFace(CullFace.NONE);
The stroke type of a shape can be −
None − No culling is performed (CullFace.NONE).
Front − All the front facing polygons are culled. (CullFace.FRONT).
Back − All the back facing polygons are culled. (StrokeType.BACK).
By default, the cull face of a 3-Dimensional shape is Back.
Example
The following program is an example which demonstrates various cull faces of the sphere. Save this code in a file with the name SphereCullFace.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.CullFace; import javafx.stage.Stage; import javafx.scene.shape.Sphere; public class SphereCullFace extends Application { @Override public void start(Stage stage) { //Drawing Sphere1 Sphere sphere1 = new Sphere(); //Setting the radius of the Sphere sphere1.setRadius(50.0); //Setting the position of the sphere sphere1.setTranslateX(100); sphere1.setTranslateY(150); //setting the cull face of the sphere to front sphere1.setCullFace(CullFace.FRONT); //Drawing Sphere2 Sphere sphere2 = new Sphere(); //Setting the radius of the Sphere sphere2.setRadius(50.0); //Setting the position of the sphere sphere2.setTranslateX(300); sphere2.setTranslateY(150); //Setting the cull face of the sphere to back sphere2.setCullFace(CullFace.BACK); //Creating a Group object Group root = new Group(sphere1, sphere2); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Sphere"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls SphereCullFace.java java --module-path %PATH_TO_FX% --add-modules javafx.controls SphereCullFace
Output
On executing, the above program generates a JavaFX window displaying two spheres with cull face values FRONT and BACK respectively as follows −
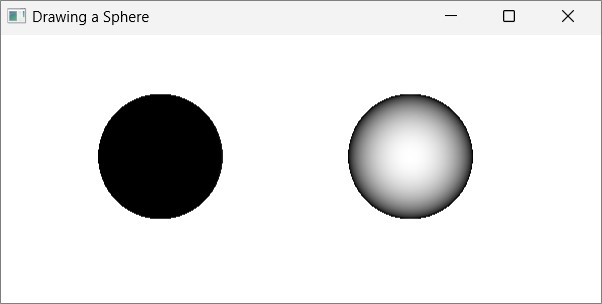
Example
The following program is an example which demonstrates when no cull face property is applied using NONE attribute on a box. Save this code in a file with the name BoxCullFace.java.
import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.shape.CullFace; import javafx.stage.Stage; import javafx.scene.shape.Box; public class BoxCullFace extends Application { @Override public void start(Stage stage) { //Drawing Box1 Box box1 = new Box(); //Setting the dimensions of the Box box1.setWidth(100.0); box1.setHeight(100.0); box1.setDepth(100.0); //Setting the position of the box box1.setTranslateX(100); box1.setTranslateY(150); box1.setTranslateZ(0); //setting the cull face of the box to NONE box1.setCullFace(CullFace.NONE); //Creating a Group object Group root = new Group(box1); //Creating a scene object Scene scene = new Scene(root, 600, 300); //Setting title to the Stage stage.setTitle("Drawing a Box"); //Adding scene to the stage stage.setScene(scene); //Displaying the contents of the stage stage.show(); } public static void main(String args[]){ launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls BoxCullFace.java java --module-path %PATH_TO_FX% --add-modules javafx.controls BoxCullFace
Output
On executing, the above program generates a JavaFX window displaying a box with cull face values NONE respectively as follows −
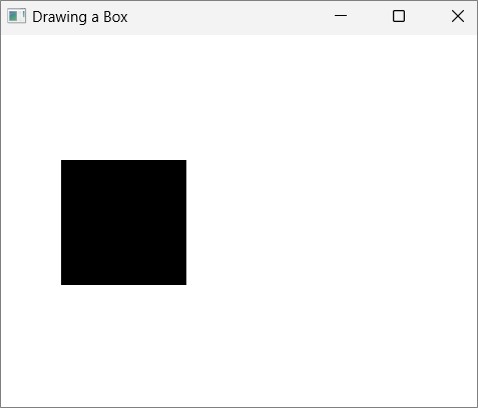