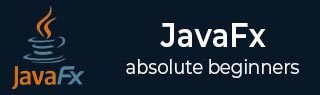
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Button Bar
A ButtonBar is a type of container that arranges buttons in a horizontal layout. The arrangement or positions of these buttons depend on the type of operating system we are working on. Generally, all the buttons placed inside a ButtonBar are uniformly sized. However, it also allows us to customize the size as well as positions of the buttons. A typical button bar looks like the below figure. It contains two buttons namely "Yes" and "No".
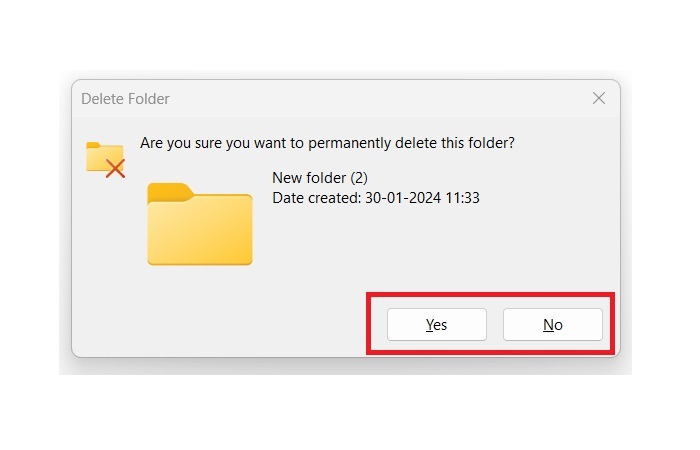
ButtonBar in JavaFX
In JavaFX, the class named ButtonBar represents a button bar. This class belongs to the package javafx.scene.control. We can create a button bar node in JavaFX by instantiating the ButtonBar class.
There are two constructors available for this class, and they are as follows −
ButtonBar() − It is used for creating a button bar with default properties that will be specific to the operating system.
ButtonBar(String buttonOrder) − It will create a button bar with the specified button order.
Steps to create a Button Bar in JavaFX
To create a Button Bar in JavaFX, follow the steps given below.
Step 1: Create two or more Buttons
In JavaFX, the buttons are created by instantiating the class named Button which belongs to a package javafx.scene.control. Instantiate this class as shown below −
//Creating required buttons Button buttonOne = new Button("Back"); Button buttonTwo = new Button("Accept"); Button buttonThree = new Button("Cancel");
Similarly, create the required number of buttons for the project.
Step 2: Instantiate ButtonBar class
Instantiate the ButtonBar class of package javafx.scene.control without passing any parameter value to its constructor and add all the buttons using the getButtons() method.
//Creating a ButtonBar ButtonBar newButtonbar = new ButtonBar(); // Adding buttons to the ButtonBar newButtonbar.getButtons().addAll(buttonOne, buttonTwo, buttonThree);
Step 3: Launching Application
After creating the button bar, follow the given steps below to launch the application properly −
Firstly, instantiate the class named HBox and add the button bar using getChildren() method.
Then, instantiate the class named Scene by passing the HBox object as a parameter value to its constructor. We can also pass dimensions of the application screen as optional parameters to this constructor.
Then, set the title to the stage using the setTitle() method of the Stage class.
Now, a Scene object is added to the stage using the setScene() method of the class named Stage.
Display the contents of the scene using the method named show().
Lastly, the application is launched with the help of the launch() method.
Example
Following is the program which will create a button bar in JavaFX. Save this code in a file with the name JavafxButtonBar.java.
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.ButtonBar; import javafx.scene.control.Button; import javafx.scene.layout.HBox; import javafx.stage.Stage; public class JavafxButtonBar extends Application { @Override public void start(Stage stage) { //Creating required buttons Button buttonOne = new Button("Back"); Button buttonTwo = new Button("Accept"); Button buttonThree = new Button("Cancel"); //Creating a ButtonBar ButtonBar newButtonbar = new ButtonBar(); // Adding buttons to the ButtonBar newButtonbar.getButtons().addAll(buttonOne, buttonTwo, buttonThree); newButtonbar.setPadding(new Insets(10)); HBox box = new HBox(); box.getChildren().addAll(newButtonbar); //Setting the stage Scene scene = new Scene(box, 500, 250); stage.setTitle("ButtonBar in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String args[]) { launch(args); } }
Compile and execute the above Java file using the command prompt with the help of following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxButtonBar.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxButtonBar
Output
On executing, the above program generates a JavaFX window displaying a ButtonBar as shown below.
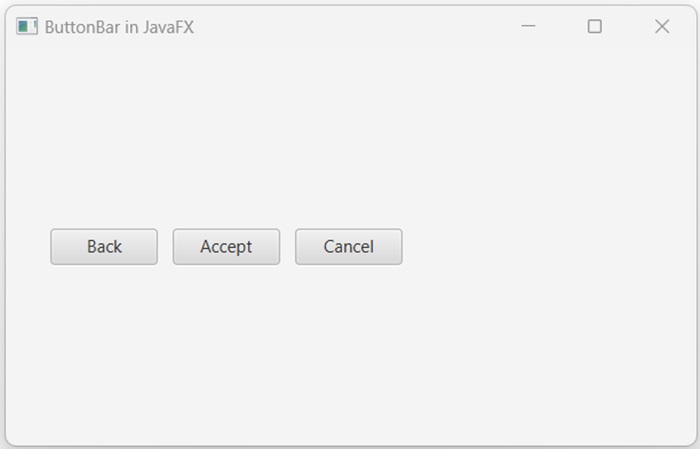
Creating a ButtonBar with customized Button orders
In most cases, the order of the Buttons is determined by the operating system. But, if a custom layout is required, then, the setButtonOrder() method of the ButtonBar class can be used. It takes button order property as a parameter and arranges the buttons accordingly. The button order properties of different OS are BUTTON_ORDER_WINDOWS, BUTTON_ORDER_MAC_OS, and BUTTON_ORDER_LINUX.
Example
In the following JavaFX program, we will create a ButtonBar by setting the button order property of MAC. Save this code in a file with the name JavafxButtonBar.java.
import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.ButtonBar; import javafx.scene.control.ButtonBar.ButtonData; import javafx.scene.control.Button; import javafx.scene.layout.HBox; import javafx.stage.Stage; public class JavafxButtonBar extends Application { @Override public void start(Stage stage) { //Creating required buttons Button buttonTwo = new Button("Yes"); Button buttonOne = new Button("No"); //Creating a ButtonBar ButtonBar newButtonbar = new ButtonBar(); // Setting the order of Buttons newButtonbar.setButtonOrder("BUTTON_ORDER_MAC_OS"); // Adding buttons to the ButtonBar newButtonbar.getButtons().addAll(buttonOne, buttonTwo); newButtonbar.setPadding(new Insets(10)); HBox box = new HBox(); box.getChildren().addAll(newButtonbar); //Setting the stage Scene scene = new Scene(box, 500, 250); stage.setTitle("ButtonBar in JavaFX"); stage.setScene(scene); stage.show(); } public static void main(String args[]) { launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxButtonBar.java java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxButtonBar
Output
When we execute the above code, it will generate the following output.
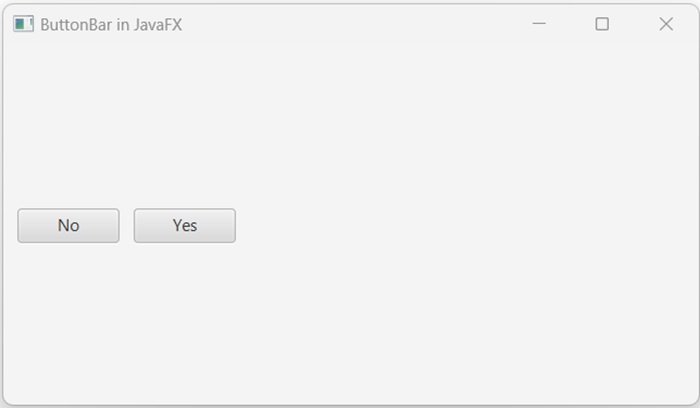