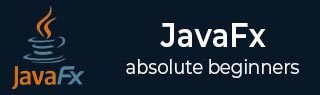
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX Animations
- JavaFX - Animations
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - HTMLEditor
An HTML editor is a type of text editor where users can create and edit HTML code within the JavaFX application. Some of the popular HTML text editors include Notepad, Sublime Text, Atom, Vscode and so on.
Note − The HTML is a markup language used for developing web applications.
In JavaFX, the HTML editor is represented by a class named HTMLEditor. This class belongs to the package javafx.scene.web. By instantiating this class, we can embed an HTMLEditor node in JavaFX.
The JavaFX HTMLEditor provides the following features −
It supports text indentation and alignment.
We can create bulleted as well as numbered lists.
It allows us to change the background and foreground colors.
It also includes text styling features such as colors, bold, italic and underline.
We can also set the font size and font family.
Embedding an HTMLEditor in JavaFX
As mentioned earlier, we can directly embed an HTML editor within the JavaFX application by instantiating the HTMLEditor class. Similar to other UI controls, it is necessary to add the HTMLEditor instance to the Scene object to make it visible in the JavaFX application.
Example
The following JavaFX program demonstrates how to embed an HTML editor into a JavaFX application. Save this code in a file with the name JavafxHtmlEditor.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.*; import javafx.stage.Stage; import javafx.scene.web.HTMLEditor; public class JavafxHtmlEditor extends Application { @Override public void start(Stage stage) { // Instantiating HTMLEditor class HTMLEditor editorhtml = new HTMLEditor(); // including the HTMLEditor to Scene Scene scene = new Scene(editorhtml, 600, 500); // setting the stage to display editor stage.setScene(scene); stage.setTitle("HTML Editor in JavaFX"); stage.show(); } public static void main(String[] args) { launch(args); } }
To compile and execute the saved Java file from the command prompt, use the following commands −
javac --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.web JavafxHtmlEditor.java java --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.web JavafxHtmlEditor
Output
When we execute the above code, it will generate the following output.
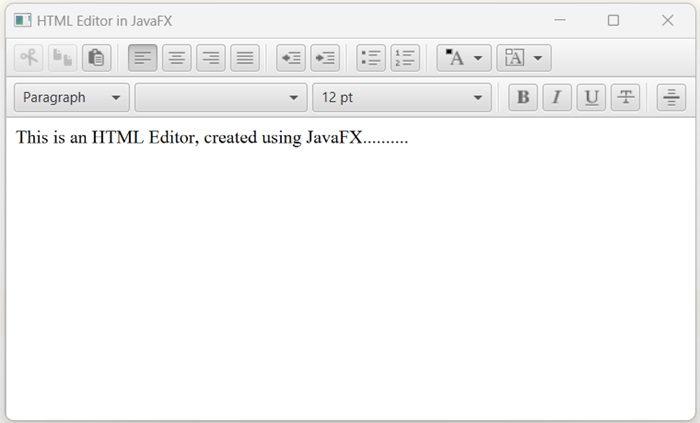
Creating an HtmlEditor in JavaFX with predefined Text
We can also provide predefined text with desired styling through JavaFX code. For this operation, we can use the setHtmlText() method of the HTMLEditor class. This method takes a String as a parameter and displays that content in the editing area when the JavaFX application starts.
Example
Following is the JavaFX program which will create an HTML editor with the predefined text. Save this code in a file with the name HtmlEditorText.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.*; import javafx.stage.Stage; import javafx.scene.web.HTMLEditor; public class HtmlEditorText extends Application { @Override public void start(Stage stage) { // Instantiating HTMLEditor class HTMLEditor editorhtml = new HTMLEditor(); // Setting the content for HTML Editor String text = "<html><body>Lorem ipsum dolor sit " + "amet, consectetur adipiscing elit. Nam tortor felis, pulvinar " + "in scelerisque cursus, pulvinar at ante. Nulla consequat" + "congue lectus in sodales. Nullam eu est a felis ornare.</body></html>"; editorhtml.setHtmlText(text); // including the HTMLEditor to Scene Scene scene = new Scene(editorhtml, 600, 500); // setting the stage to display editor stage.setScene(scene); stage.setTitle("HTML Editor in JavaFX"); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.web HtmlEditorText.java java --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.web HtmlEditorText
Output
When we execute the above code, it will generate the following output.
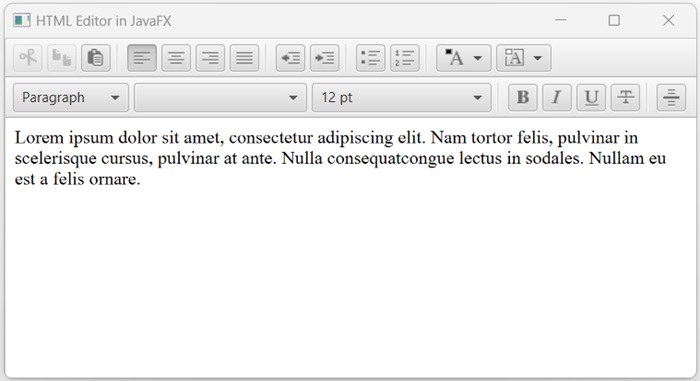
Generating HTML code using HtmlEditor in JavaFX
The HTMLEditor class provides a method named getHtmlText() to retrieve the content of the editing area. This method is called along with the object of HTMLEditor class.
Example
In the following JavaFX program, we will create an HTML editor to create and edit content, a button to obtain respective HTML code and a text area to show obtained code. Save this code in a file with the name HtmlgetText.java.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.*; import javafx.scene.control.*; import javafx.stage.Stage; import javafx.scene.web.HTMLEditor; import javafx.event.ActionEvent; import javafx.geometry.Insets; import javafx.geometry.Pos; public class HtmlgetText extends Application { @Override public void start(Stage stage) { // Instantiating HTMLEditor class HTMLEditor editorhtml = new HTMLEditor(); editorhtml.setPrefHeight(300); // Creating a Text area to show HTML Code TextArea code = new TextArea(); ScrollPane pane = new ScrollPane(); pane.setContent(code); pane.setFitToWidth(true); pane.setPrefHeight(300); // creating button to get code Button button = new Button("Get Code"); button.setOnAction(a -> { code.setText(editorhtml.getHtmlText()); }); // Creating root VBox root = new VBox(); root.setPadding(new Insets(10)); root.setSpacing(5); root.setAlignment(Pos.BOTTOM_LEFT); root.getChildren().addAll(editorhtml, button, pane); // including the HTMLEditor to Scene Scene scene = new Scene(root, 625, 500); // setting the stage to display editor stage.setScene(scene); stage.setTitle("HTML Editor in JavaFX"); stage.show(); } public static void main(String[] args) { launch(args); } }
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.web HtmlgetText.java java --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.web HtmlgetText
Output
On executing, the above program generates a JavaFX window displaying the below output.
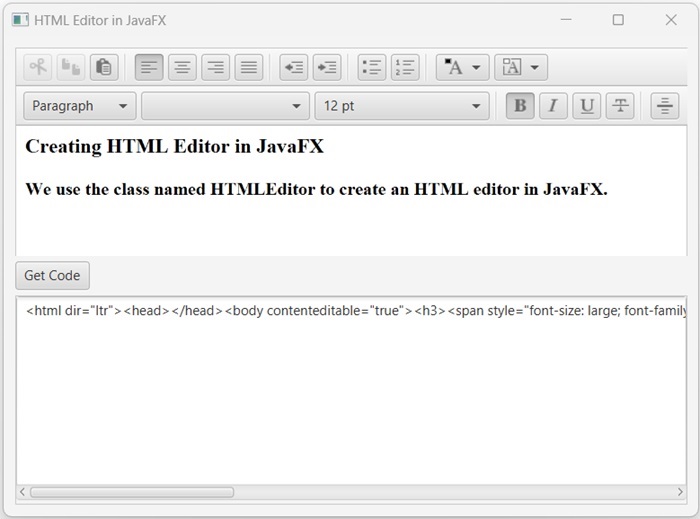