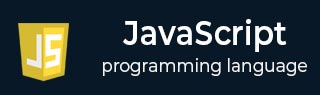
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Yield Operator
JavaScript Yield Operator
The yield operator in JavaScript is used to pause and resume the generator function asynchronously. In JavaScript, generator functions are the special functions that you can pause or resume while executing. The generator functions are defined with the 'function*' syntax. The yield keyword can only be used within the generator function that contains it.
The yield operator pauses the execution of generator function and returns its operand (expression) to the generator's caller.
Syntax
The syntax of the yield operator in JavaScript is as follows −
yield expression;
Paramter
expression − The value to yield from the generator function via the iterator protocol. 'undefined' is yielded, if expression is omitted.
Return value
It returns the optional value passed to the generator's next() method to resume its execution.
Yield Operator in Genrator Function
To understand the yield operator, let's understand fist the working of the generator function.
When a generator function is called, it returns a generator object. When the next() method this generator object is called it resumes the execution of the generator function. When a yield expression is encountered, it pauses the execution and returns the expression after yield keyword to the object's caller (the next() method).
The next() method of the generator object returns an iterator object with two properties – value and done. The value is the actual value of the expression and the done is a boolean value. The done property is true if the execution of the generator function is completely executed, else it if false.
Below is a complete example code of generator function with yield keyword (operator).
function* test() { // function code yield expression; } const genObj = test(); genObj.next();
In the above syntax, 'function*' is used to create a generator function named test, and the yield keyword is used to return 'expression' from the function.
The generator function test is called and assigned the returned generator object to the variable genObj. The next() method resumes the execution of the function and returns iterator object when encounters yield expression.
Let's execute the below JavaScript code snippet –
function* test() { console.log("I'm before yield expression"); yield 20; } const genObj = test(); console.log(genObj.next());
Notice when we call next() method, it display the message in the console first and then display the iterator object.
I'm before yield expression { value: 20, done: false }
Example: Returning a value
In the example below, we have defined the test() generator function. We used the yield operator 3 times in the function to return a number, an array, and a string, respectively.
After that, we used the next() method four times to resume the execution of the function. Whenever the control flow finds the yield operator, it will stop execution and return the value.
In the output, you can observe that it returns the object containing the operand of the yield operator and boolean value.
function* test() { yield 20; yield [1,2,3]; yield "Hello World"; } let res = test(); console.log(res.next()); console.log(res.next()); console.log(res.next()); console.log(res.next());
Output
{ value: 20, done: false } { value: [ 1, 2, 3 ], done: false } { value: 'Hello World', done: false } { value: undefined, done: true }
Example: Returning undefined
When we omit the expression following the yield keyword, it will return undefined.
function* test() { yield; } let res = test(); console.log(res.next()); console.log(res.next());
Output
{ value: undefined, done: false } { value: undefined, done: true }
Example: Passing a value to the next() method
We can also pass a value to the next() method. In the below example, we have passed 30 to the second next() method. It evaluates yield to 30. The variable result is assigned the value of yield which is evaluated as 30.
function* test() { let result = yield 20; console.log("default value paased to next() method " + result); } let res = test(); console.log(res.next()); console.log(res.next(30));
Output
{ value: 20, done: false } default value paased to next() method 30 { value: undefined, done: true }
Example
In the below code, we used the loop, and in each operation, we stop the execution of the function using the yield operator. Afterward, we use the next() method to start the execution of the generator function.
// Generator function function* test() { for (let p = 0; p < 6; p += 2) { yield p; } } let res = test(); console.log(res.next()); console.log(res.next()); console.log(res.next()); console.log(res.next());
Output
{ value: 0, done: false } { value: 2, done: false } { value: 4, done: false } { value: undefined, done: true }
In real-time development, programmers use the 'yield' operator for asynchronous operations, lazy evaluations, task scheduling, iterating through large datasets, creating custom iterators, etc.