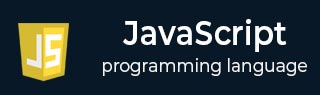
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Geolocation API
Geolocation API
The Geolocation API is a web API that provides a JavaScript interface to access the user's geographical location data. A Geolocation API contains the various methods and properties that you can use to access the user's location on your website.
It detects the location of the user's using the device's GPS. The accuracy of the location depends on the accuracy of the GPS device.
As location compromises the users' privacy, it asks for permission before accessing the location. If users grant permission, websites can access the latitude, longitude, etc.
Sometimes, developers need to get the user's location on the website. For example, if you are creating an Ola, Uber, etc. type applications, you need to know the user's location to pick them up for the ride.
The Geolocation API allows users to share the location with a particular website.
Real-time use cases of the Geolocation API
Here are the real-time use cases of the Geolocation API.
To get the user's location coordinates, show them on the map.
To tag the user's location on the photograph.
To suggest nearby stores, food courts, petrol pumps, etc., to users.
To get the current location for the product or food delivery.
To pick up users for the ride from their current location.
Using the Geolocation API
To use the Geolocation API, you can use the 'navigator' property of the window object. The Navigator object contains the 'geolocation' property, containing the various properties and methods to manipulate the user's location.
Syntax
Follow the syntax below to use the Geolocation API.
var geolocation = window.navigator.geolocation; OR var geolocation = navigator.geolocation;
Here, the geolocation object allows you to access the location coordinates.
Example: Checking the browser support
Using the navigator, you can check whether the user's browser supports the Geolocation.geolocation property.
The code below prints the message accordingly whether the Geolocation is supported.
First, we convert the data into the JSON format. After that, we convert the data into the string and print it on the web page.
<html> <body> <div id = "output"> </div> <script> const output = document.getElementById("output"); if (navigator.geolocation) { output.innerHTML += "Geolocation is supported by your browser." } else { output.innerHTML += "Geolocation is not supported by your browser." } </script> </body> </html>
Output
Geolocation is supported by your browser.
Geolocation Methods
Here are the methods of the Geolocation API.
Method | Description |
---|---|
getCurrentPosition() | It is used to retrieve the current geographic location of the website user. |
watchPosition() | It is used to update the user's live location continuously. |
clearWatch() | To clear the ongoing watch of the user's location using the watchPosition() method. |
The Location Properties
The getCurrentPosition() method executes the callback function you passed as an argument. The callback function takes the object as a parameter. The parametric object contains various properties with information about the user's location.
Here, we have listed all properties of the location object in the table below.
Property | Type | Description |
---|---|---|
coords | objects | It is an object you get as a parameter of the callback function of the getCurrentPosition() method. It contains the below properties. |
coords.latitude | Number | It contains the latitude of the current location in decimal degrees. The value range is [-90.00, +90.00]. |
coords.longitude | Number | It contains the longitude of the current location in decimal degrees. The value range is [-180.00, +180.00]. |
coords.altitude | Number | It is an optional property, and it specifies the altitude estimate in meters above the WGS 84 ellipsoid. |
coords.accuracy | Number | It is also optional and contains the accuracy of the latitude and longitude estimates in meters. |
coords.altitudeAccuracy | Number | [Optional]. It contains the accuracy of the altitude estimate in meters. |
coords.heading | Number | [Optional]. It contains information about the device's current direction of movement in degrees counting clockwise relative to true north. |
coords.speed | Number | [Optional]. It contains the device's current ground speed in meters per second. |
timestamp | date | It contains the information about the time when the location information was retrieved, and the Position object created. |
Getting User's Location
You can use the getCurrentPosition() method to get the user's current location.
Syntax
Users can follow the syntax below to get the user's current position using the getCurrentPosition() method.
navigator.geolocation.getCurrentPosition(successCallback, errorCallback, options);
Parameters
The getCurrentPosition() object takes 3 parameters.
successCallback − This function will be called when the method successfully retrieves the user's location.
errorCallback − This function will be called when the method throws an error while accessing the user's location.
Options − It is an optional parameter. It is an object containing properties like timeout, maxAge, etc.
Permitting the website to access your location
Whenever any website tries to access your location, the browser pops up the permission alert box. If you click the 'allow’, it can fetch your location details. Otherwise, it throws an error.
You can see the permission pop-up in the image below.
Example
In the below code, we use the getCurrentPosition() method to get the user's location. The method calls the getCords() function to get the current location.
In the getCords() function, we print the value of the various properties of the cords object.
First, we convert the data into the JSON format. After that, we convert the data into the string and print it on the web page.
<html> <body> <h3> Location Information </h3> <button onclick = "findLocation()"> Find Location </button> <p id = "output"> </p> <script> const output = document.getElementById("output"); function findLocation() { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(getCords); // } else { output.innerHTML += "Geo Location is not supported by this browser!"; } } // Callback function function getCords(coords) { output.innerHTML += "The current position is: <br>"; output.innerHTML += "Latitude: " + coords.coords.latitude + "<br>"; output.innerHTML += "Longitude: " + coords.coords.longitude + "<br>"; output.innerHTML += "Accuracy: " + coords.coords.accuracy + "<br>"; output.innerHTML += "Altitude: " + coords.coords.altitude + "<br>"; } </script> </body> </html>
Geolocation Errors
The getCurrentPosition() method takes the callback function as a second parameter to handle the error. The callback function can have an error object as a parameter.
In the below cases, an error can occur.
When the user has denied access to the location.
When location information is not available.
When the request for the location is timed out.
It can also generate any random error.
Here is the list of properties of the error object.
Property | Type | Description |
---|---|---|
code | Number | It contains the error code. |
message | String | It contains the error message. |
Here is the list of different error codes.
Code | Constant | Description |
---|---|---|
0 | UNKNOWN_ERROR | When methods of the geolocation object can’t retrieve the location, it returns the code 0 for unknown error. |
1 | PERMISSION_DENIED | When the user has denied the permission to access the location. |
2 | POSITION_UNAVAILABLE | When it can’t find the location of the device. |
3 | TIMEOUT | When the method of the geolocation object can’t find the user’s position. |
Example: Error Handling
We use the getCurrentPosition() method in the findLocation() function in the below code. We have passed the errorCallback() function as a second parameter of the getCurrentPosition() method to handle the errors.
In the errorCallback() function, we use the switch case statement to print the different error messages based on the error code.
When you click the Find Location button, it will show you a pop-up asking permission to access the location. If you click on the 'block’, it will show you an error.
First, we convert the data into the JSON format. After that, we convert the data into the string and print it on the web page.
<html> <body> <div id = "output"> </div> <button onclick = "findLocation()"> Find Location </button> <script> const output = document.getElementById("output"); function findLocation() { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(getCords, errorCallback); // } else { output.innerHTML += "Geo Location is not supported by this browser!"; } } // Callback function function getCords(coords) { output.innerHTML += "The current position is: <br>"; output.innerHTML += "Latitude: " + coords.coords.latitude + "<br>"; output.innerHTML += "Longitude: " + coords.coords.longitude + "<br>"; } // Function to handle error function errorCallback(err) { switch (err.code) { case err.PERMISSION_DENIED: output.innerHTML += "You have denied to access your device's location"; break; case err.POSITION_UNAVAILABLE: output.innerHTML += "Your position is not available."; break; case err.TIMEOUT: output.innerHTML += "Request timed out while fetching your location."; break; case err.UNKNOWN_ERROR: output.innerHTML += "Unknown error occurred while fetching your location."; break; } } </script> </body> </html>
Geolocation Options
The getCurrentPosition() method takes the object containing the options as a third parameter.
Here is the list of options you can pass as a key to the option object.
Property | Type | Description |
---|---|---|
enableHighAccuracy | Boolean | It represents whether you want to get the most accurate location. |
timeout | Number | It takes a number of milliseconds as a value for that much time you want to wait to fetch the location data. |
maximumAge | Number | It takes the milliseconds as a value, specifying the maximum age of the cached location. |
Example
The below code finds the most accurate location. Also, we have set milliseconds to the maximumAge, and timeout properties of the options object.
First, we convert the data into the JSON format. After that, we convert the data into the string and print it on the web page.
<html> <body> <div id = "output"> </div> <button onclick = "findLocation()"> Find Location </button> <script> const output = document.getElementById("output"); function findLocation() { if (navigator.geolocation) { // Options for geolocation const options = { enableHighAccuracy: true, timeout: 5000, maximumAge: 0 }; navigator.geolocation.getCurrentPosition(getCords, errorfunc, options); } else { output.innerHTML += "Geo Location is not supported by this browser!"; } } // Callback function function getCords(coords) { output.innerHTML += "The current position is: <br>"; output.innerHTML += "Latitude: " + coords.coords.latitude + "<br>"; output.innerHTML += "Longitude: " + coords.coords.longitude + "<br>"; } function errorfunc(err) { output.innerHTML += "The error message is - " + err.message + "<br>"; } </script> </body> </html>
Watching the Current Location of the User
The watchPosition() method allows you to track the live location of users. It returns the ID, which we can use with the clearWatch() method when you want to stop tracking the user.
Syntax
Follow the syntax below to use the watchPosition() method to track the live location of users.
var id = navigator.geolocation.watchPosition(successCallback, errorCallback, options)
The errorCallback and options are optional arguments.
If you want to stop tracking the user, you can follow the syntax below.
navigator.geolocation.clearWatch(id);
The clearWatch() method takes the id returned by the watchPosition() method as an argument.
Example
In the below code, we used the geolocation object's watchPosition () method to get the user's continuous position.
We used the getCords() function as a callback of the watchPosition() method, where we print the latitude and longitude of the user's position.
In the findLocation() method, we used the setTimeOut() method to stop tracking after 30 seconds.
In the output, you can observe that the code prints the user's position multiple times.
First, we convert the data into the JSON format. After that, we convert the data into the string and print it on the web page.
<html> <body> <button onclick = "findLocation()"> Find Location </button> <div id = "output"> </div> <script> let output = document.getElementById("output"); function findLocation() { if (navigator.geolocation) { let id = navigator.geolocation.watchPosition(getCoords); setTimeout(function () { navigator.geolocation.clearWatch(id); output.innerHTML += "<br>Tracking stopped!"; }, 30000); // Stop tracking after 30 seconds. } else { output.innerHTML += "<br>Geo Location is not supported by this browser!"; } } // Callback function function getCoords(location) { let latitude = location.coords.latitude; let longitude = location.coords.longitude; output.innerHTML += `<br> Latitude: ${latitude}, Longitude: ${longitude}`; } </script> </body> </html>