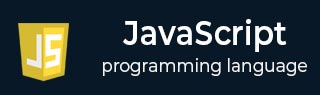
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - ECMAScript 2017
The ECMAScript 2017 version of JavaScript was released in 2017. ECMAScript 2017 introduced a number of new features to the language. One the most notable features is async/await syntax which allows us to write asynchronous operations in a more synchronous style. It provided shared memory and atomics that enhances support for concurrent programming.
In this chapter, we will discuss all the new added features in ECMAScript 2017.
New Features Added in ECMAScript 2017
Here are the new methods, features, etc., added to the ECMAScript 2017 version of JavaScript.
padStart() and padEnd() methods
Object.entries() method
Object.values() method
JavaScript async and await
Object getOwnPropertyDescriptors() Method
JavaScript Shared Memory
Here, we have explained each feature in detail.
String Padding: padStart() and padEnd() methods
The ECMAScript 2017 introduced two string methods, padStart() and padEnd() methods, that allow you to add padding to the string at the start and end by adding a particular character. These methods are used to extend the string and achieve the desired length.
Example
In the below code, we have passed the desired string length as the first parameter of the padStart() and padEnd() method and the character as a second parameter.
However, you can also pass the string as a second parameter.
<html> <body> <div id = "output1">After padding at the start: </div> <div id = "output2">After padding at the end: </div> <script> let base = "TurorialsPoint"; let start_updated = base.padStart(18, "@"); // Padding at the start let end_updated = base.padEnd(18, "*"); // Padding at the end document.getElementById("output1").innerHTML += start_updated; document.getElementById("output2").innerHTML += end_updated; </script> </body> </html>
Output
After padding at the start: @@@@TurorialsPoint After padding at the end: TurorialsPoint****
The Object.entries() Method
ECMAScript 2017 added Object.entries() method to objects. The Object.entries() method returns an iterator to traverse the key-value pair of the object.
Example
In the below code, the employee object contains three properties. We used the Object entries() method to get the iterator of the object.
After that, we used the for...of loop to traverse object properties using the iterator.
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); const employee = { Company: "TutorialsPoint", Ex_company: "TCS", Experience: 4, } const emp_iterator = Object.entries(employee); for (let pair of emp_iterator) { output.innerHTML += pair + "<br>"; } </script> </body> </html>
Output
Company,TutorialsPoint Ex_company,TCS Experience,4
The Object.values() Method
ECMAScript 2017 introduced Object.values() method to objects. The JavaScript Object.values() method is used to get the array of values of the object properties.
Example
In the below code, we used the Object.values() method to get all the values of the object in the array.
<html> <body> <div id = "output">Object values are: </div> <script> const wall = { color: "blue", size: "15x12", paintBrand: "Asiant paints" } document.getElementById("output").innerHTML += " " + Object.values(wall); </script> </body> </html>
Output
Object values are: blue,15x12,Asiant paints
JavaScript async and await
The async and await keywords are added to the language in ECMAScript 2017. The async/await keywords are used to create asynchronous functions. The async keyword is used to create asynchronous functions, and await keyword handles the operations.
Example
The printMessage() function is asynchronous in the below code. We have defined a new promise inside the function and stored it in the getData variable.
The promise takes the time of 0.5 seconds to resolve. The ‘await’ keyword handles the promise and blocks the function code execution until the promise gets resolved.
<html> <body> <div id = "output"> </div> <script> async function printMessage() { let getData = new Promise(function (res, rej) { setTimeout(() => { res("Promise resolved !!"); }, 500); }); document.getElementById("output").innerHTML = await getData; } printMessage(); </script> </body> </html>
Output
Promise resolved !!
The Object.getOwnPropertyDescriptors() Method
The Object.getOwnPropertyDescriptor() method returns the property descriptors for each property, such as writable, enumerable, configurable, value, etc. It is metadata for the object property.
Example
In the below code, we get the property descriptors for each property of the object using the getOwnPrpopertyDescriptors() method.
<html> <body> <div id = "output">The Property descriptors of the wall object is: <br></div> <script> const wall = { color: "blue", thickness: 10, } document.getElementById("output").innerHTML += JSON.stringify(Object.getOwnPropertyDescriptors(wall)); </script> </body> </html>
Output
The Property descriptors of the wall object is: {"color":{"value":"blue","writable":true,"enumerable":true,"configurable":true},"thickness":{"value":10,"writable":true,"enumerable":true,"configurable":true}}
JavaScript Shared Memory and Atomics
In JavaScript, share memory allows multiple threads to share a memory, enabling efficient communication between multiple threads.
JavaScript is a single-threaded programming language. However, you can use the web workers to run the JavaScript code in the different threads.
In 2018, SharedArrayBuffer was introduced to share memory and perform atomic operations by sharing the data.