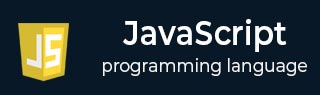
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Object Properties
JavaScript Object Properties
An object property in JavaScript is a key: value pair, where key is a string and value can be anything. The key in key: value pair is also called property name. So the properties are association between key (or name) and value.
An object is in other terms a collection of properties (key: value pairs). However, key: value pairs are not stored in the specific order in the object. To write an object syntax, the curly braces are used. Each key: value pair is written within curly braces separated by a comma.
You can manipulate the object properties in JavaScript. For example, you can add, delete, or update the object's properties.
Syntax
You can follow the syntax below to define properties in the object.
const fruit = { name: "Apple", price: 100, }
In the above syntax, fruit is an object. The fruit object contains the name and price properties. The value of the name property is 'Apple’, and the price is 100.
In an object, the key can either be a string or a symbol only. If you use another data type as a key, the object implicitly converts it into the string.
The property value can be anything like an object, set, array, string, set, function, etc.
Accessing Object Properties
There are 3 ways to access object properties in JavaScript.
Using the dot notation
Using the square bracket notation
Using the expression
The Dot Notation
You can access the object property using the dot notation/ syntax.
obj.prop;
In the above syntax, 'obj' is an object, and 'prop' is its property whose value you need to access.
Example
The 'fruit' object in the example below contains the name and price property. We access the object properties using the dot notation, and you can see property values in the output.
<!DOCTYPE html> <html> <body> <p id = "output"> </p> <script> const fruit = { name: "Banana", price: 20, } document.getElementById("output").innerHTML = "The price of the " + fruit.name + " is " + fruit.price; </script> </body> </html>
Output
The price of the Banana is 20
The Square Bracket Notation
You can use the square bracket pair containing the property as a string followed by the object name to access a particular property.
obj["prop"]
In the above syntax, we access the 'prop' property from the object.
You can't access the property using the dot notation when you use invalid identifiers as an object key. So, you need to use the square bracket notation. The identifier is invalid if it starts from a number, contains a space, or a hyphen.
Example
In the example below, we access the fruit object's name and price property values.
<!DOCTYPE html> <html> <body> <p id = "output"> </p> <script> const fruit = { name: "Banana", price: 20, } document.getElementById("output").innerHTML = "The price of the " + fruit["name"] + " is " + fruit["price"]; </script> </body> </html>
Output
The price of the Banana is 20
Using the expression inside the bracket
Sometimes, you require to access the object properties dynamically using the variable or expression. So, you can write the expression inside the square bracket notation. The expression can be a variable, a mathematical expression, etc.
obj[expression]
The above syntax evaluates the expression first and accesses the property same as a resultant value from the object. You don't need to write the expression in quotes.
Example
In the example below, the num object contains the number as a key in the string format and its word representation as a value.
We use the variable x to access the property value from the object. Also, we used the "x + 10" mathematical expression to access the object property dynamically.
<!DOCTYPE html> <html> <body> <p id = "output"> </p> <script> const num = { 10: "ten", 20: "Twenty", } const x = 10; document.getElementById("output").innerHTML = num[x + 10]; </script> </body> </html>
Output
Twenty
Accessing the Nested Object Properties
Accessing the nested object properties is very similar to accessing the object properties. You can either use the dot or square bracket notation.
Syntax
Obj.prop.nestedProp // OR Obj["prop"]["nestedProp"];
In the above syntax, the prop is a property of the obj object, and nestedProp is a property of the 'prop' object.
Example
In the below code, the 'cars' object contains the nested objects named OD and BMW. We access the nested object properties using the dot and square bracket notation.
<!DOCTYPE html> <html> <body> <p id = "output1"> </p> <p id = "output2"> </p> <script> const cars = { totalBrands: 50, audi: { model: "Q7", price: 10000000, }, bmw: { model: "S20D", price: 8000000, } } document.getElementById("output1").innerHTML = "The model of Audi is " + cars.audi.model + " and its price is " + cars.audi.price; document.getElementById("output2").innerHTML = "The model of BMW is " + cars["bmw"]["model"] + " and its price is " + cars["bmw"]["price"]; </script> </body> </html>
Output
The model of Audi is Q7 and its price is 10000000 The model of BMW is S20D and its price is 8000000
Adding or Updating the Object Properties
You can update or add new properties to the object using the dot or square bracket notation. You can access the object property and assign a new value to it. If the property already exists, it updates the property value. Otherwise, it adds the property to the object.
Syntax
Obj.prop = new_value; OR Obj["prop"] = new_value;
In the above syntax, we update the value of the 'prop' property of the obj object.
Example
In the example below, we update the name and price property of the fruit object. Also, we add the expiry property to the fruit object.
<!DOCTYPE html> <html> <body> <p id = "output"> </p> <script> const fruit = { name: "Watermealon", price: 150, } fruit.name = "Apple"; // Updating using the dot notation fruit["price"] = 200; // Updating using the bracket notation fruit.expiry = "5 days"; // Adding new property to the object. document.getElementById("output").innerHTML += "The price of " + fruit.name + " is " + fruit.price + " and it expires in " + fruit.expiry; </script> </body> </html>
Output
The price of Apple is 200 and it expires in 5 days
Deleting the Object Properties
You can use the 'delete' operator to delete the specific object properties.
Syntax
delete obj.prop;
In the above syntax, obj.prop is an operator for the delete operator.
Example
In the example below, we delete the name property from the fruit object using the delete operator. The output shows that the fruit object contains only the price property after deleting the name property.
<!DOCTYPE html> <html> <body> <p> The object after deleting the "name" property: </p> <p id = "output"> </p> <script> const fruit = { name: "Watermealon", price: 150, } delete fruit.name; document.getElementById("output").innerHTML = JSON.stringify(fruit); </script> </body> </html>
Output
The object after deleting the "name" property: {"price":150}
Enumerating the Object Properties
There are various approaches to enumerating the object properties. The Object.keys() method returns the object's keys in the array. However, we will use the for…in loop to traverse through each property of the object.
Syntax
You can follow the syntax below to iterate through the object properties.
for (let key in table) { // Use the key to access its value }
In the above syntax, 'key' is an object property, which can be used to access its value.
Example
In the example below, we have created the table object containing 3 properties. After that, we used the for…in loop to traverse through each property of the object and access its value.
<!DOCTYPE html> <html> <body> <p> Iterating the obejct properties</p> <p id = "output"> </p> <script> const table = { color: "brown", shape: "round", price: 10000, } for (let key in table) { document.getElementById("output").innerHTML += key + ": " + table[key] + "<br>"; } </script> </body> </html>
Output
Iterating the obejct properties color: brown shape: round price: 10000
Property Attributes
The object property contains four attributes.
value − A value of the object.
enumerable − Contains boolean value representing whether the object is iterable.
configurable − Contains the boolean value representing whether the object is configurable.
writable − It also contains the boolean value, representing whether the object is writable.
By default, you can't edit other attributes except the value attribute of the object property. You need to use the defineProperty() or defineProperties() methods to update other attributes.