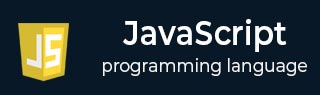
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Object Accessors
The object accessor properties in JavaScript are methods that get or set the value of an object. They are defined using the get and set keywords. Accessor properties are a powerful way to control how your objects are accessed and modified.
The JavaScript object can have two kinds of properties.
Data properties
Accessor properties
The below property is called the data properties.
const obj = { key: "value", // Data property }
Object Accessor Properties
In JavaScript, you can use the getters to get the object properties and setters to set the object properties.
There are two keywords to define accessor properties.
get − The get keyword is used to define a method to get the object property value.
set − The set keyword is used to define a method to update the object property value.
JavaScript Getters
The getters are used to access the object properties. To define a method as getter, we use get keyword followed by method name. Follow the syntax below to define the getter.
get methodName() { // Method body } obj.methodName;
In the above syntax, we have defined the getters using the 'get' keyword followed by the method name.
You can use the method name as an object property to get its returned value.
You don't need to write the pair of parenthesis followed by the method name to execute the getters. You can access it like the object property.
Example
In the example below, in the wall object, we have defined the getColor() getters. The getColor() returns the value of the color property.
After that, we use the getColor() method to access the color property value of the object.
<html> <body> <p id = "output">The color of the wall is : </p> <script> const wall = { color: "brown", get getColor() { return this.color; } } document.getElementById("output").innerHTML += wall.getColor; </script> </body> </html>
Output
The color of the wall is : brown
JavaScript Setters
The setters are used to update the JavaScript object properties. To define a method as setter, we use set keyword followed by method name You can follow the syntax below to define setters in the JavaScript object.
set methodName(param) { // Setter method return this.color = color; } wall.methodName = "Red";
In the above syntax, the 'set' keyword is used to define the setter method.
The method_name can be any valid identifier.
The setter method always takes a single parameter. If you don't pass a parameter or multiple parameters, it will give you an error.
You can assign value to the setter method as you assign value to the property.
Example
In the example below, we have defined the setter method to set the value of the color property of the wall object. We set the 'red' value to the color property of the object using the 'setColor' setter method.
<html> <body> <p id = "output"> </p> <script> const wall = { color: "brown", set setColor(color) { return this.color = color; } } document.getElementById("output").innerHTML += "The color of the wall before update is : " + wall.color + "<br>"; //updating the color of wall wall.setColor = "Red"; document.getElementById("output").innerHTML += "The color of the wall after update is : " + wall.color; </script> </body> </html>
Output
The color of the wall before update is : brown The color of the wall after update is : Red
JavaScript Object Methods vs. Getters/Setters
In JavaScript, whatever you can do using the getters and setters, you can also do by defining the specific object method. The difference is that getters and setters provide simpler syntax.
Let's understand it with the help of some examples.
Example
In the example below, we have defined the getColor() getters method and colorMethod() object method in the wall object. Both method returns the color value.
You can observe that defining and accessing getters is more straightforward than defining and accessing the object method.
<html> <body> <p id = "output"> </p> <script> const wall = { color: "brown", get getColor() { return this.color; }, colorMethod: function () { return this.color; } } document.getElementById("output").innerHTML += "Getting the color using the getters : " + wall.getColor + "<br>"; document.getElementById("output").innerHTML += "Getting the color using the object method : " + wall.colorMethod(); </script> </body> </html>
Output
Getting the color using the getters : brown Getting the color using the object method : brown
Data Quality and Security
The getter and setter methods can provide better data quality. Also, they are used for encapsulation to secure the object data.
Let's understand how getter and setter improve data quality and provide security via the example below.
Example
In the example below, the getColor() is a getter method, returning the value of the color property after converting it to the uppercase. Also, it hides the color property from users, as you can access its value using the getColor() method.
<html> <body> <p id = "output"> </p> <script> const wall = { color: "Brown", get getColor() { return this.color.toUpperCase(); } } document.getElementById("output").innerHTML += "Getting the color using the getters : " + wall.getColor; </script> </body> </html>
Output
Getting the color using the getters : BROWN
Defining getters/setters using the Object.defineProperty()
You can also use the Object.defineProperty() method to add getters or setters into the object.
Object.defineProperty(object, "methodName", { keyword: function () { // Method body }, })
Using the above syntax, you can define the getter or setter same as methodName.
Parameters
object − It is an object where you need to add getters or setters.
methodName − It is the name of the method for getters or setters.
keyword − It can be 'get' or 'set' according to you want to define the getter or setter method.
Example
In the example below, we have added the getSize and setSize getter and setter to the object using the object.defineProperty() method.
After that, we use the getSize and setSize to get and set the size, respectively.
<html> <body> <p id = "demo"> </p> <script> const output = document.getElementById("demo"); const door = { size: 20, } Object.defineProperty(door, "getSize", { get: function () { return this.size; } }); Object.defineProperty(door, "setSize", { set: function (value) { this.size = value; }, }) output.innerHTML += "Door size is : " + door.getSize + "<br>"; door.setSize = 30; output.innerHTML += "Door size after update is : " + door.getSize; </script> </body> </html>
Output
Door size is : 20 Door size after update is : 30
Reasons to use getters and setters
Here are the benefits of using the getters and setters in JavaScript.
It has a simple syntax than object methods.
To improve the data quality by validating the data.
For the encapsulation or securing the data.
It also allows abstraction or data hiding.