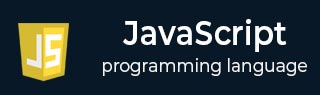
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Custom Events
Custom Events
The custom events in JavaScript define and handle custom interactions or signals within an application. They establish a communication mechanism between various sections of your code: one part can notify others about specific occurrences or changes; thus enhancing the functionality of your program.
Typically, users utilize custom events in conjunction with the Event and CustomEvent interfaces. The following provides a detailed breakdown of their functionality:
Concept | Description |
---|---|
Custom Event | The CustomEvent constructor in JavaScript facilitates communication between various parts of an application by defining a user-specific event. Such custom events manifest as instances of this constructor. |
CustomEvent Constructor | The built-in JavaScript constructor creates custom events, utilizing two parameters: the event type, a string, and a discretionary configuration object; for instance, an optional detail property can be used to provide supplementary data. |
dispatchEvent Method | A method available on DOM elements that dispatches a custom event. It triggers the execution of all listeners for that event type on the specified element. |
addEventListener Method | A method available on DOM elements to attach an event listener function to an event type. The listener function is executed when the specified event is dispatched on the element. |
Event Types | Strings that identify the type of event. Custom events can have any user-defined string as their type. |
Event Handling | Listening for and responding to events is an active process. It primarily involves the creation of listeners for specific event types in custom contexts, and subsequently defining precise actions that will occur when these events take place. |
Pub/Sub Pattern | In this design pattern, system components communicate with each other indirectly and without direct references. By utilizing custom events, one can implement a publish/subscribe pattern that enables various application sections to subscribe to specific events and react accordingly. |
detail Property | An optional property in the configuration object when creating a custom event. It allows you to pass additional data (as an object) along with the event. |
Example: Basic Custom Event
In this example, we initiate a custom event named 'myCustomEvent' and render an associated button. Using the addEventListener method, we track events triggered by this button. Upon clicking the button, our action dispatches the custom event; subsequently alerting a message "Custom event triggered!"
<!DOCTYPE html> <html> <body> <button id="triggerBtn">Trigger Event</button> <script> // Creates the new custom event. const customEvent = new Event('myCustomEvent'); // Adds an event listener to the button. document.getElementById('triggerBtn').addEventListener('click', function() { // Dispatches custom event on button click. document.dispatchEvent(customEvent); }); // Add listener for the custom event. document.addEventListener('myCustomEvent', function() { alert('Custom event triggered!'); }); </script> </body> </html>
Example: Custom Event with Data
In this example we will make use of the CustomEvent which is an interface and extends the primary Event. The detail property will be demonstrated here which allows us to set additional data. The custom event name will be 'myCustomEventWithData' and it will have a message associated to it. This custom event will be getting dispatched upon the click of a button. When this button is clicked, this event will be triggered and the message set will be alerted on screen.
<!DOCTYPE html> <html> <body> <button id="triggerBtn">Trigger Custom Event</button> <script> const eventData = { message: 'Hello from custom event!' }; const customEvent = new CustomEvent('myCustomEventWithData', { detail: eventData }); document.getElementById('triggerBtn').addEventListener('click', function() { document.dispatchEvent(customEvent); }); document.addEventListener('myCustomEventWithData', function(event) { alert('Custom event triggered with data: ' + event.detail.message); }); </script> </body> </html>
Example: Condition-based Event Dispatching
This example illuminates a scenario: event dispatching critically hinges on a variable (v), being conditionally based. It underscores your application's dynamic use of custom events, dependent upon specific conditions. The case at hand involves the dispatching either 'TutorialEvent' or 'TutorialEvent2' determined by the value of v; correspondingly, an event listener reacts accordingly to this choice.
<!DOCTYPE html> <html> <body> <script> var v='tutorialspoint'; const event = new Event("TutorialEvent"); const event2 = new Event("TutorialEvent2"); document.addEventListener('TutorialEvent', ()=>{ alert("Welcome to Tutorialspoint Event") }); document.addEventListener('TutorialEvent2', ()=>{ alert("Welcome to Event 2") }); if(v == 'tutorialspoint'){ document.dispatchEvent(event); } else{ document.dispatchEvent(event2); } </script> </body> </html>
To summarize the steps for creating custom events, we first create an event or Custom event, add the listener using the addEventListener (preferably) and then we trigger or dispatch the event using the. dispatchEvent method.