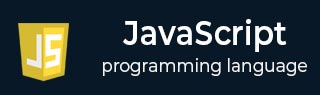
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - addEventListener()
The JavaScript addEventListener() method is used to attach an event handler function to an HTML element. This allows you to specify a function that will be executed when a particular event occurs on the specified element.
Events are a specific occurrence or action like user clicks, keypress or page loads. The browser detects these events and triggers associated JavaScript functions known as event handlers to respond accordingly.
Developers employ the 'addEventListener()' method for linking particular HTML elements with specific function behaviours when those events occur. Examples of events include clicks, mouse movements, keyboard inputs, and document loading.
Syntax
The basic syntax for addEventListener() is as follows −
element.addEventListener(event, function, options);
Here element is an HTML element, such as a button, input or div - can be selected using methods like getElementById, getElementsByClassName, getElementsByTagName and querySelector; these are just a few examples. The event listener attaches to this particular element.
Parameters
The addEventListener() method accepts the following parameters −
event − a string that embodies the type of action − for instance, "click", "mouseover", or "keydown" among others; will serve as our trigger to execute the given function.
function − a named, anonymous or reference to an existing function is called when the specified event occurs; it's essentially the operation that facilitates execution at predetermined instances.
options (optional) − it allows for the specification of additional settings particularly capturing or once behaviours related to the event listener.
Examples
Example: Alert on Clicking Button
In this example, we will have a simple button being displayed which upon clicking shows an alert on the screen. The addeventlistener will be responsible for handling the event “click” which means it will call a function handleClick which throws an alert to the screen when the button is clicked. We make use of the getElementById to fetch the button we want to bind the event listener to.
This is a commonly used event when it comes to submitting on forms, login, signup etc.
<html> <head> <title>Click Event Example</title> </head> <body> <p> Click the button below to perform an event </p> <button id="myButton">Click Me</button> <script> // Get the button element const button = document.getElementById("myButton"); // Define the event handler function function handleClick() { alert("Button clicked!"); } // Attach the event listener to the button button.addEventListener("click", handleClick); </script> </body> </html>
Example: Colour Change on Mouse Over
In this example we have a dig tag which will initially be of light blue colour. Upon hovering the mouse on this div tag, it will change to red and back to blue if we hover out.
There are two events in this case, mouseover and mouseout. The mouseover means the mouse moves onto an element mouseout means the mouse movies out of an element.
There are two functions here, one for mouseover and one for mouseout. Upon mouseover, the background colour property is set to light coral (a shade of red) and upon mouseout, the background colour is set to light blue.
These types of mouse hover events are commonly seen when hovering over the navbar of a lot of websites.
<html> <head> <title>Mouseover Event Example</title> <style> #myDiv { width: 600px; height: 200px; background-color: lightblue; } </style> </head> <body> <div id="myDiv">Hover over me</div> <script> // Get the div element const myDiv = document.getElementById("myDiv"); // Define the event handler function function handleMouseover() { myDiv.style.backgroundColor = "lightcoral"; } // Attach the event listener to the div myDiv.addEventListener("mouseover", handleMouseover); // Additional example: Change color back on mouseout function handleMouseout() { myDiv.style.backgroundColor = "lightblue"; } myDiv.addEventListener("mouseout", handleMouseout); </script> </body> </html>
There can be multiple event listeners for the same elements like in the case of 2nd example which has two event listeners (for mouseover and mouseout). Event listeners can be removed using the removeEventListener function. By passing a parameter in the options as once:true, we can ensure that the event listener is removed after being invoked once and this is important in certain case scenarios like payments.
It is important to note that one should never use the "on" prefix for specifying events, this simply means for a click event, we should specify it as "click" and not "onclick".