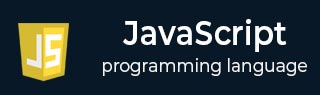
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Browser Object Model
The Browser Object Model (BOM) in JavaScript refers to the objects provided by the browsers to interact with them. By using these objects, you can manipulate the browser's functionality. For example, you can get the browser history and window size, navigate to different URLs, etc.
The Browser object model is not standardized. It depends on which browser you are using.
Here, we have listed all objects of the Browser Object Model with descriptions −
Window − The 'window' object represents the current browser window. You can use it to manipulate the browser window.
Document − The 'document' object represents the currently opened web page in the browser window. You can use it to customize the property of the document.
Screen − It provides information about the user's device's screen.
History − It provides the browser's session history.
Navigator − It is used to get the browser's information like default language, etc.
Location − The Location object is used to get the URL information, such as the hostname of the current web page.
Console − The console object allows developers to access the browser's console.
JavaScript Window Object
The JavaScript window object represents the browser's window. We can use different methods and properties of the window object to manipulate current browser window. For example, showing an alert, opening a new window, closing the current window, etc.
All the JavaScript global variables are properties of window object. All global functions are methods of the window object.
The other objects listed above such as document, screen, history etc., are the properties of the window object. We can access these objects as properties of the window object. We can also access them with referencing the window object. Look at the below example snippet to access the document object –
window.document.write("Welcome to Tutorials Point");
or without window object −
document.write("Welcome to Tutorials Point");
The innerHeight and innerWidth properties of the window object are used to access the height and width of the browser window. We will learn JavaScript window object in detail in next chapters.
JavaScript Document Object
The document object is a property of the JavaScript window object. The whole HTML document is represented as a document object. The document object forms HTML DOM. It is root node of the HTML document.
The document object can be accessed as window.document or just document.
The document object provides us with many properties and methods to access the HTML elements and manipulate them. One such method is document.getElementById() to access the HTML element using its id.
We can access an element with id as "text" using getElementById() method and manipulate it –
Example
<html> <body> <div id ="text">This text will be changed. </div> <script> // Access the element with id as text const textDiv = document.getElementById("text"); // Change the content of this element textDiv.innerHTML = "The text of this DIV is changed."; </script> </body> </html>
Output
The text of this DIV is changed.
JavaScript Screen Object
In JavaScript, the screen object is a property of window object. It provides us with properties that can be used to get the information about the device window screen. We can access the screen object as window.screen or just screen.
To get the width and height of the device screen in pixels we can use the screen.width and screen.height properties –
Example
<html> <body> <div id ="width">Width of the device screen is </div> <div id ="height">Height of the device screen is </div> <script> document.getElementById("width").innerHTML += screen.width + " px."; document.getElementById("height").innerHTML += screen.height + " px."; </script> <p> The above result may be different for different device.</p> </body> </html>
Output
Width of the device screen is 1536 px. Height of the device screen is 864 px. The above result may be different for different device.
JavaScript History Object
In JavaScript, the history object is also a property of the window object. It contains a list of the visited URLs in the current session. The history object provides an interface to manipulate the browser's session history.
The JavaScript history object can be accessed using window.history or just history. We can use the methods of history object to navigate the URLs in the history list. For example to go the previous page/URL in the history list, we can use history.back() method.
JavaScript Navigator Object
The JavaScript navigator object is also a property of the window object. Using the 'navigator' object, you can get the browser version and name and check whether the cookie is enabled in the browser. We can access the navigator object using window.navigator. We can also access it without using the window prefix.
JavaScript Location Object
The JavaScript 'location' object contains various properties and methods to get and manipulate the information of the browser's location, i.e. URL. It's also a property of JavaScript window object.
We can use the properties and methods of the location object to manipulate the URL information. For example, to get the host from the current URL, we can use the window.location.host or just location.host. The host is property of the location object.
Example
<html> <body> <div id = "output"> </div> <script> document.getElementById("output").innerHTML = "The host of the current location is: " + location.host; </script> </body> </html>
Output
The host of the current location is: www.tutorialspoint.com
JavaScript Console Object
The JavaScript console object allows us to access the debugging console of the browser. It is a property of the JavaScript window object. It can be accessed using window.console or just console.
The console object provides us with different methods such as console.log(). The console.log() method is used to display the message in debugging console.
What's Next?
We have provided detailed information about each of the above objects in separate chapters.