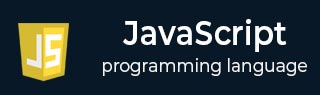
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - ECMAScript 2021
The ECMAScript 2021 standard was released in 2021. ECMAScript 2021 brought many notable features to JavaScript. The introduction of the String replaceAll() method simplified global string replacement. Logical Assignment Operators, (&&=, ||=, and ??=), enhanced code conciseness. This update focused on improving developer productivity and code readability.
This chapter will discuss all the newly added features in ECMAScript 2021.
New Features Added in ECMAScript 2021
Here are the new methods, features, etc., added to the ECMAScript 2021 version of JavaScript.
- Numeric Separators (_)
- Promise any() method
- String replaceAll() method
- Logical AND Assignment Operator (&&=)
- Logical OR Assignment (||=)
- Nullish Coalescing Assignment (??=)
Here, we have explained each feature in detail.
Numeric Separators (_)
ECMAScript 2021 introduced numeric separators. The numeric seprators are used to make the number more readable.
Example
We added a numeric separator in the code below to make the number more readable.
<html> <body> <div id = "output">The value of the num is: </div> <script> let num = 90_00_000; document.getElementById("output").innerHTML += num; </script> </body> </html>
Output
The value of the num is: 9000000
Promise any()Method
ECMAScript 2021 introduced Promise any() method. The promise.any() method fulfills any promise from the array of promises, which resolves at the earliest.
Example
In the below code, we have created multiple promises and passed them as an argument of the Promise.any() method.
We have resolved the promise1 and rejected the promise2. For the Promise.any() method, JavaScript control goes into the then() block as promise1 gets resolved.
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); const promise1 = new Promise((res, rej) => res()); const promise2 = new Promise((res, rej) => rej()); const promises = [promise1, promise2]; Promise.any(promises) .then(() => { output.innerHTML += 'One or more promises are resolved!'; }) .catch((err) => { output.innerHTML += 'All promises are rejected:' + err; }); </script> </body> </html>
Output
One or more promises are resolved!
String replaceAll() Method
The ECMAScript 2021 introduced String replaceAll() method to the Strings. The string replaceAll() method is used to replace the particular substring with another substring.
The replaceAll() method takes either string or regular expression as a parameter.
Example
In the below code, we have replaced the lowercase 'a' with the uppercase 'A' using the replaceAll() method.
<html> <body> <div id = "output1">Original string is: </div> <div id = "output2">Updated string is: </div> <script> let str = "abcd abcd abcd abcd"; document.getElementById("output1").innerHTML += str; let updatedStr = str.replaceAll("a", "A"); document.getElementById("output2").innerHTML += updatedStr; </script> </body> </html>
Output
Original string is: abcd abcd abcd abcd Updated string is: Abcd Abcd Abcd Abcd
Logical AND Assignment Operator (&&=) Operator
ECMAScript 2021 introduced the logical assignment operators (&&=, ||= and ??=) to the operators. The JavaScript logical AND Assignment operator updates the value of the first operand with the second operand if the first operand is true.
Example
In the code below, the str string's value is not falsy. So, it updates the value of an str variable with 'Hi'.
<html> <body> <div id = "output">The value of the str is: </div> <script> let str = "Hello"; str &&= "Hi"; document.getElementById("output").innerHTML += str; </script> </body> </html>
Output
The value of the str is: Hi
Logical OR Assignment (||=) Operator
ECMAScript 2021 introduced the logical OR Assignment operator to operators. It updates the value of the first operand with the second operand if the first operand is false.
Example
In the code below, the initial value of the str is false. So, the Logical OR assignment operator updates its value with the second operand, which is 10.
<html> <body> <div id = "output">The value of the str is: </div> <script> let str = false; str ||= 10; document.getElementById("output").innerHTML += str; </script> </body> </html>
Output
The value of the str is: 10
Nullish Coalescing Assignment (??=) Operator
The ECMAScript 2021 introduced the nullish coalescing assignment operator to operators. This operator updates the value of the left operand if it is undefined or null.
Example
In the below code, the value of the str variable is null. So, the nullish coalescing assignment operator assigns the 'default' value to the str variable.
<html> <body> <div id = "output">The value of the str is: </div> <script> let str = null; str ??= "default"; document.getElementById("output").innerHTML += str; </script> </body> </html>
Output
The value of the str is: default
Warning – Some of the above features are not supported by some browsers. So, you can use the polyfill to avoid errors.