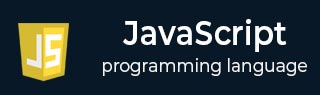
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - The Reflect Object
JavaScript Reflect
In JavaScript, Reflect is a global object and was introduced in ECMAScript 6 (ES6). It contains static methods, providing a better way to interact with the object at runtime.
Unlike most global objects, Reflect is not a constructor. You cannot use it with the new operator or invoke the Reflect object as a function. All methods of Reflect are static just like the Math object.
It contains various methods to access, update, delete, etc. properties from the object at run time.
Key Features of the Reflect Object
Object construction − The Reflect API allows to create objects at run time using the Reflect.construct() method.
Function invocation − The apply() method of the Reflect API is used to invoke a function or object method by passing the context and argument.
Object property manipulation − A Reflect API has different methods to set, update, or delete the object properties. Also, it can handle the extension of the object by preventing it.
Syntax
Following is the syntax to use the method of the Reflect API −
Reflect.method();
In the above syntax, the 'method' is the generalization. It can be any method of the Reflect API, such as get, set, apply, construct, has, etc.
Reflect Methods
In the following table, we have listed all the methods of Reflect −
Sr.No. | Name & Description |
---|---|
1 | apply() To invoke a function. |
2 | construct() Allows to specify the different prototypes. |
3 | defineProperty() Allows to insert or edit object property. |
4 | deleteProperty() Allows to delete the object property. |
5 | get() To get a value of the property. |
6 | getOwnPropertyDescriptor() Allows to get the descriptor of the object. |
7 | getPrototypeOf() To get the prototype of the object. |
8 | has() To check whether the property exists in the object. |
9 | isExtensible() Allows to check whether the object is extensible. |
10 | ownKeys() Returns the own keys of the targeted object and ignores the inherited keys. |
11 | preventExtensions() To prevent the extension of the object. |
12 | set() To set the value to the object property. |
13 | setPrototypeOf() Allows to set the prototype of the object as null or another object. |
Examples
Example 1
In the example below, we have defined a car object. The 'prop' variable contains the property name in the string format.
We use the get() method of the Reflect object to access the 'model' property from the car object. We passed the object name as a first parameter of the get() method and the property name as a second parameter.
In the output, you can see the value of the 'model' property.
<html> <body> <div id="output">The model of the car is: </div> <script> const car = { name: "Audi", model: "Q6", price: 7000000, } let prop = "model"; let model = Reflect.get(car, prop); document.getElementById("output").innerHTML += model; </script> </body> </html>
Output
The model of the car is: Q6
Example 2
In the example below, we used the set() method to set the property into the object. The set() method takes an object, property name, and value as a parameter.
The output shows the 'doors' property value. In this way, you can set the property into the object at run time using the set() method of the Reflect API.
<html> <body> <div id="output">The total doors in the Audi Q6 is: </div> <script> const car = { name: "Audi", model: "Q6", price: 7000000, } const model = Reflect.set(car, "doors", 4); document.getElementById("output").innerHTML += car.doors; </script> </body> </html>
Output
The total doors in the Audi Q6 is: 4
On executing the above script, the output window will pop up, displaying the text on the webpage.
Example 3
In the example below, we used the has() method to check whether the particular property exists in the object dynamically.
Both symbols look similar but are different, which you can see in the output.
<html> <body> <p id="output"></p> <script> const car = { name: "Audi", model: "Q6", price: 7000000, } const isName = Reflect.has(car, "name"); if (isName) { document.getElementById("output").innerHTML = "The Car object contains the name."; } </script> </body> </html>
Output
The Car object contains the name.