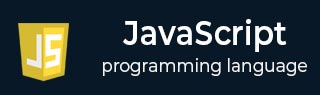
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Objects Overview
JavaScript Objects
The JavaScript object is a non-primitive data type that is used to store data as key-value pairs. The key-value pairs are often referred as properties. A key in a key-value pair, also called a "property name", is a string and value can be anything. If a property's value is a function, the property is known as a method.
Objects are created using curly braces and each property is separated by a comma. Each property is written as property name followed by colon (:) followed by property value. The key: value pairs are not stored in the specific order in the object. So an object is an unordered collection of properties written as key: value pairs.
JavaScript is an Object Oriented Programming (OOP) language. A programming language can be called object-oriented if it provides four basic capabilities to developers.
Encapsulation − the capability to store related information, whether data or methods, together in an object.
Abstraction − the capability to hide object's implementation details from users.
Inheritance − the capability of a class to rely upon another class (or number of classes) for some of its properties and methods.
Polymorphism − the capability to write one function or method that works in a variety of different ways.
Let's understand in details about the JavaScript objects.
Object Properties
Object properties can be any of the primitive data types, objects or functions. Object properties are usually variables that are used internally in the object's methods, but can also be globally visible variables that are used throughout the page.
The syntax for adding a property to an object is −
objectName.objectProperty = propertyValue;
For example − The following code gets the document title using the "title" property of the document object.
var str = document.title;
Object Methods
Methods are the functions that let the object do something or let something be done to it. There is a small difference between a function and a method – at a function is a standalone unit of statements and a method is attached to an object and can be referenced by the this keyword.
Methods are useful for everything from displaying the contents of the object to the screen to performing complex mathematical operations on a group of local properties and parameters.
For example − Following is a simple example to show how to use the write() method of document object to write any content on the document.
document.write("This is test");
Creating New Objects
All user-defined objects and built-in objects are descendants of an object called Object.
We can use object literals to create a new user-defined object. Alternatively, we can create a constructor function and then invoke this function using new keyword to instantiate an object.
There are different ways to create an object in JavaScript. Here, we will learn all ways given below.
Using the Object Literal
Using the Object Constructor
Using the Object.create() Method
Using JavaScript ES6 Classes
The JavaScript Object Literal
In JavaScript, ‘{}’ is represented by the object literal. You can add pair of key-value pairs between curly braces to define an object.
You can follow the syntax below to use the object literal to define objects.
const obj = { key: val, }
You can add key-value pairs between curly braces. Each key-value pair is comma separated, and you need to add a colon (:) between the key and value.
Example
In the example below, we have defined a wall object containing the 4 properties. Each property contains the different values of different data types.
In the output, you can observe the object properties and its value.
<html> <body> <p id = "output"> </p> <script> const myBook = { title: "Perl", author: "Mohtashim", pages: 355, } document.getElementById("output").innerHTML = "Book name is : " + myBook.title + "<br>" +"Book author is : " + myBook.author + "<br>" +"Total pages : " + myBook.pages; </script> </body> </html>
Output
Book name is : Perl Book author is : Mohtashim Total pages : 355
The JavaScript new Operator
The new operator is used to create an instance of an object. To create an object, the new operator is followed by the constructor method.
In the following example, the constructor methods are Object(), Array(), and Date(). These constructors are built-in JavaScript functions.
var employee = new Object(); var books = new Array("C++", "Perl", "Java"); var day = new Date("August 15, 1947");
The JavaScript Object() Constructor
A constructor is a function that creates and initializes an object. JavaScript provides a special constructor function called Object() to build the object. The return value of the Object() constructor is assigned to a variable.
The variable contains a reference to the new object. The properties assigned to the object are not variables and are not defined with the var keyword.
Example
Try the following example; it demonstrates how to create an Object.
<html> <body> <p id = "output"> </p> <script> var book = new Object(); // Create the object book.subject = "Perl"; // Assign properties to the object book.author = "Mohtashim"; document.getElementById("output").innerHTML = "Book name is : " + book.subject + "<br>" + "Book author is : " + book.author; </script> </body> </html>
Output
Book name is : Perl Book author is : Mohtashim
The JavaScript Constructor Function
In JavaScript, you can define a custom function and use it as a constructor function to define a new object. Here, the custom function works as a template.
The benefit of the custom user-defined constructor function over the Object() constructor is that you can add arguments to the custom function according to your requirements.
Below is simple syntax to use the custom user-defined constructor function to create an object.
// Object template function ConstructorFunc(para) { this.para = para; } const obj = new ConstructorFunc(arg);
The ConstructorFunc() function works as an object template. It uses the 'this' keyword to access the context of the function and define the key in the function context. Also, the key is initialized with the 'para' value.
Next, you can use the function as a constructor with a 'new' keyword to define the object and pass the required arguments to the constructor.
Example
This example demonstrates how to create an object with a user-defined constructor Function. Here this keyword is used to refer to the object that has been passed to a function.
<html> <body> <div id = "output"> </div> <script> function Book(title, author) { this.title = title; this.author = author; } const myBook = new Book("Perl", "Mohtashim"); document.getElementById("output").innerHTML = "Book title is : " + myBook.title + "<br>" + "Book author is : " + myBook.author + "<br>"; </script> </body> </html>
Output
Book title is : Perl Book author is : Mohtashim
The JavaScript Object.create() Method
The Object.create() method creates a new object from the already defined object. Also, you can add some new properties to the object prototype while cloning one object from another object using the Object.create() method.
Follow the syntax below to use the Object.create() method to define a new object.
const obj = Object.create({}, { key: { value: val }, })
{} − It is an empty object. The Object.create() method creates a copy of it.
{ key: { value: val }, } − It is an object containing the properties to add to the prototype of the cloned object.
Example
In the example below, we added multiple properties to the prototype of the empty object. However, if you print the object, you won't be able to see any properties as they are added to the prototype.
You can access the object properties with a dot notation.
<html> <body> <p id = "output"> </p> <script> const myBook = Object.create({}, { title: { value: "Perl" }, author: { value: "Mohtashim" }, }) document.getElementById("output").innerHTML = "Book title is : " + myBook.title + "<br>" + "Book author is : " + myBook.author + "<br>"; </script> </body> </html>
Output
Book title is : Perl Book author is : Mohtashim
The JavaScript ES6 Classes
The JavaScript classes are introduced in ES6. The JavaScript classes are template to create objects. A class is defined using the "class" keyword. It is similar to the function while defining a class. The class keyword is followed by the class name and then class body.
class MyClass{ //class body }
You can use the new operator to create an object using a class −
const myObj = new MyClass();
Here ClassName is the name of class and myObject is the name of object creating using this class.
We have discussed the JavaScript classes in details in the next chapter.
Defining Methods for an Object
The previous examples demonstrate how the constructor creates the object and assigns properties. But we need to complete the definition of an object by assigning methods to it.
Example
Try the following example; it shows how to add a function along with an object.
<html> <head> <title>User-defined objects</title> <script> // Define a function which will work as a method function addPrice(amount) { this.price = amount; } function Book(title, author) { this.title = title; this.author = author; this.addPrice = addPrice; // Assign that method as property. } </script> </head> <body> <div id = "output"> </div> <script> var myBook = new Book("Perl", "Mohtashim"); myBook.addPrice(100); document.getElementById("output").innerHTML = "Book title is : " + myBook.title + "<br>" +"Book author is : " + myBook.author + "<br>" +"Book price is : " + myBook.price + "<br>"; </script> </body> </html>
Output
Book title is : Perl Book author is : Mohtashim Book price is : 100
The 'with' Keyword
The ‘with’ keyword is used as a kind of shorthand for referencing an object's properties or methods.
The object specified as an argument to with becomes the default object for the duration of the block that follows. The properties and methods for the object can be used without naming the object.
Syntax
The syntax for with object is as follows −
with (object) { properties used without the object name and dot }
Example
Try the following example.
<html> <head> <script> // Define a function which will work as a method function addPrice(amount) { with(this) { price = amount; } } function Book(title, author) { this.title = title; this.author = author; this.price = 0; this.addPrice = addPrice; // Assign that method as property. } </script> </head> <body> <div id = "output"></div> <script> var myBook = new Book("Perl", "Mohtashim"); myBook.addPrice(100); document.getElementById("output").innerHTML = "Book title is : " + myBook.title + "<br>" +"Book author is : " + myBook.author + "<br>" +"Book price is : " + myBook.price + "<br>"; </script> </body> </html>
Output
Book title is : Perl Book author is : Mohtashim Book price is : 100
JavaScript Native Objects
JavaScript has several built-in or native objects. These objects are accessible anywhere in your program and will work the same way in any browser running in any operating system.
Here is the list of all important JavaScript Native Objects −
JavaScript Object Methods
Here, we have listed the methods of JavaScript object.
Static methods
These methods are invoked using the Object class itself.
Sr.No. | Method | Description |
---|---|---|
1 | assign() | To copy properties and their values from one object to another object. |
2 | create() | To create a new object using an existing object as prototype. |
3 | defineProperty() | To make a clone of the object and add new properties to its prototype. |
4 | defineProperties() | To define a property into a particular object and get the updated object. |
5 | entries() | It returns an array containing the [key, value] pairs. |
6 | freeze() | To prevent adding or updating object properties by freezing the object. |
7 | fromEntries() | To create a new object from the array of the [key, value] pairs. |
8 | getOwnPropertyDescriptor() | To get the property descriptor for the properties of the object. |
9 | getOwnPropertyNames() | To get object properties. |
10 | getOwnPropertySymbols() | To get all symbols in the array form which are present in the object. |
11 | getPrototypeOf() | To get the prototype of the object. |
12 | hasOwn() | To check whether the particular property exists in the object. |
13 | Object.is() | To check whether the two objects contain a similar value. |
14 | isExtensible() | To check if an object is extensible. |
15 | isFrozen() | To check if the object is frozen. |
16 | isSealed() | To check if the object is sealed. |
17 | keys() | To get all keys of the object in the array format. |
18 | preventExtensions() | To prevent the prototype updation of the object. |
19 | seal() | To seal the object. |
20 | setPrototypeOf() | To set a prototype of the object. |
21 | toLocaleString() | To get the object in the string format. |
22 | values() | To get all values of the object in the array format. |
Instance methods
These methods are invoked using the instance of the object.
Sr.No. | Method | Description |
---|---|---|
1 | defineGetter() | To define getters to get the particular property value. |
2 | hasOwnProperty() | To check if the object has a particular property as its own property. |
3 | isPrototypeOf() | To check whether the particular object exists as a prototype of the other object. |
4 | propertyIsEnumerable() | To check whether the property of the object is enumerable. |
Object Properties
Sr.No. | Property | Description |
---|---|---|
1 | constructor | To get the reference to the constructor function of the object. |