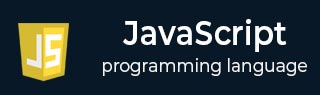
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Function Hoisting
Function Hoisting
The function hoisting in JavaScript is a default behavior in which function declarations are moved at the top of their local scope before execution of the code. So, you can call the function in its scope before it is declared. It's important to notice that only declaration is hoisted not the initialization. So the variables or functions should have been initialized before they are used.
Similar to the function hoisting, the variable hoisting is also a default behavior in which the variable declarations are moved at the top of their local scope. We can use the function before it has been declared.
Let's consider the following JavaScript code.
add(5,10); // 15 function add(x, y){ return x + y; }
In the above JavaScript code, the function add is called before it's declaration. This is possible because JavaScript interpreter hoists the function declaration to the top of the scope. So the above code is equivalent to –
function add(x, y){ return x + y; } add(5,10); // 15
The function hoisting only works with function declaration not with function expression. So, if the function is defined using the function expression,., it is not hoisted at the top.
add(5,10); // ReferenceError: Cannot access 'add' before initialization const add = function (x, y){ return x + y; }
Let's write some complete JavaScript examples of function hoisting.
Example: Function Hoisting
In the example below, we have defined the printMessage() function, printing the position from where it is called.
Also, we call the printMessage() function before and after the function definition. It prints the output without any error as the function is hoisted at the top of its scope.
<html> <body> <p id = "output"> </p> <script> printMessage("Top"); function printMessage(pos) { document.getElementById("output").innerHTML += "The function is called from the " + pos + "<br/>"; } printMessage("Bottom"); </script> </body> </html>
Output
The function is called from the Top The function is called from the Bottom
Example
In the example below, we have defined the function inside the 'if' block. So, the function is hoisted at the top of the 'if' block, and you can execute the function only inside the 'if' block before its initialization.
You can't access the function outside the 'if' block.
<html> <body> <p id = "output"> </p> <script> // test("Top"); // Out of scope if (1) { test("Top"); // In the same scope function test(pos) { document.getElementById("output").innerHTML += "The function is called from the " + pos + "<br/>"; } test("Bottom"); // In the same scope } </script> </body> </html>
Output
The function is called from the Top The function is called from the Bottom
The hoisting is very important behavior in JavaScript. But it is always advisable to declare the function or variable at the beginning of the code. Since JavaScript always interprets the code in sequence of declaration, then initialization and then usage.
JavaScript Variable Hoisting
The hoisting behaviors of JavaScript move the variables declaration to the top of the variable's scope by default. In JavaScript, variables declared with the 'var' keyword are hoisted at the top of its scope, but variables declared with the 'let' and 'const' keyword is not hoisted at the top.
For example,
var x = y; var y;
The above code is similar to the below code, as the variables declaration is hoisted at the top.
var y; var x = y;
Let's understand the variable hoisting by following examples.
Example: Variable Hoisting
In the example below, we have initialized the variable y, printed its value in the output, and declared it at the end. The below code prints a value without any error, as variable y is hoisted at the top in the global scope.
<html> <head> <title> JavaScript - Variable Hoisting </title> </head> <body> <p id = "output"> </p> <script> y = 10; document.getElementById("output").innerHTML = "The value of the y is : " + y; var y; </script> </body> </html>
Output
The value of the y is : 10
Example: Variable Hoisting with Function
In the example below, we have defined the printNum() function. In the printNum() function, we have initialized the variable y, printed its value, and declared it afterward.
The variable y is hoisted at the top of the function so you can access it before its declaration, but you can't access it outside the function.
<html> <head> <title> JavaScript - Variable Hoisting with function </title> </head> <body> <p id = "output"> </p> <script> const output = document.getElementById("output"); function printNum() { y = 20; output.innerHTML += "The value of the variable y is : " + y; var y; // Function scope } printNum(); // Variable Y can't be accessible here </script> </body> </html>
Output
The value of the variable y is : 20
However, variable initialization is not hoisted at the top of the block.
Example: variable initialization is not hoisted
The example below demonstrates that the variable is hoisted at the top, but the variable initialization is not. Here, we print the variable x without initialization of it. So, it prints the 'undefined' in the output.
<html> <body> <p id = "output"> </p> <script> var x; document.getElementById("output").innerHTML = "x is -> " + x; x = 10; </script> </body> </html>
Output
x is -> undefined
Example: Hoisting with let and const keywords
The below example demonstrates that the variable declared with the 'let' and 'const' keywords are not hoisted at the top. So, you always need to define it before using it.
We used the 'try…catch' block to handle the error. In the output, users can observe the error the below code gives when we access the variable declared with the 'let' keyword.
<html> <head> <title> Hoisting with let and const </title> </head> <body> <p id = "output"> </p> <script> const output = document.getElementById("output"); try { x = 10; output.innerHTML += "The value of x is -> " + x; let x; }catch (error) { output.innerHTML += error.message; } </script> </body> </html>
Output
Cannot access 'x' before initialization