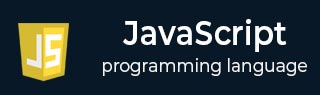
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - ECMAScript 2016
The ECMAScript 2016 version of JavaScript was released in 2016. Previously the old versions of JavaScript are named by numbers for example ES5 and ES6. Since 2016 the versions are named by the year they are released for example ECMAScript 2016, ECMAScript 17, etc. Lets discuss the featues added in ECMAScript 2016.
New Features Added in ECMAScript 2016
Here are the new methods, features, etc., added to the ECMAScript 2016 version of JavaScript.
Array includes() method
Exponentiation Operator (**)
Exponentiation Assignment Operator (**=)
Here, we have explained each feature in detail.
JavaScript Array includes() Method
The JavaScript array includes() methods is used to check whether the array contains a particular element.
Syntax
The syntax of Array includes() method in JavaScript is as follows −arr.include(searchElement, fromIndex);
Here arr is the original array, the searchElement is to be search from. The fromIndex is an optional argument if passed, the searching will start from the fromIndex index.
Example
In the below code, we use the array includes() method to check whether the watches array contains the 'Noise' brand.
<html> <body> <div id = "output">Does watches array include Noise?</div> <script> const watches = ["Titan", "Rolex", "Noise", "Fastrack", "Casio"]; document.getElementById("output").innerHTML += watches.includes("Noise"); </script> </body> </html>
Output
Does watches array include Noise? true
Example
In the below code, we use the array includes() method to check whether the subjects array contains the 'Python' subject searching from index 1.
<html> <body> <div id = "output">Does subjects array include Python fromIndex 1? </div> <script> const subjects = ["Java", "JavaScript", "Python", "C", "C++"]; document.getElementById("output").innerHTML += subjects.includes("Python", 1); </script> </body> </html>
Output
Does subjects array include Python fromIndex 1? true
JavaScript Exponentiation Operator
The JavaScript exponentiation operator is used to find the power of the first operand raised to the second operand.
Syntax
The syntax of expponentiation operator is as follow −
x ** y;
It returns the result of raising the first operand (x) to the power of the second operand (y).
Example
In the below code, we find the 22 using the exponentiation operator and store the resultant value in the 'res' variable.
<html> <body> <div id = "output">The resultant value for 2 ** 2 is: </div> <script> document.getElementById("output").innerHTML += 2 ** 2; </script> </body> </html>
Output
The resultant value for 2 ** 2 is: 4
Exponentiation Assignment Operator
The JavaScript exponentiation assignment operator raises the power of the first operand by the second operand and assigns it to the first operand.
Syntax
The syntax of exponentiation assignment operator is as follows −
x **= y;
It assigns the result of raising the first operand (x) to the power of the second operand (y) to x.
Example
In the below code, we find the 102 and assign the resultant value to the 'num' variable using the exponentiation assignment operator.
<html> <body> <div id = "output">The resultant value for 10 ** 2 is: </div> <script> let num = 10; num **= 2; // exponentiation assignment operation document.getElementById("output").innerHTML += num; </script> </body> </html>
Output
The resultant value for 10 ** 2 is: 100
ECMAScript 2016 Browser Support
Most modern browsers support the ECMAScript 2016 version of JavaScript
Chrome | Firefox | Microsoft Edge | Opera | Safari | Firefox Android |
---|---|---|---|---|---|
Yes | Yes | Yes | Yes | Yes | Yes |