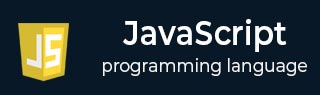
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Object Constructors
Object Constructors
An object constructor in JavaScript is a function that creates an instance of a class, which is typically called an object. A constructor is called when you declare an object using the new keyword. The purpose of a constructor is to create an object and set values if there are any object properties present.
There are two ways to create a template for the object in JavaScript - using a class and using an object constructor.
Whenever you need to create multiple objects with the same syntax, you require a template for the object. For example, you are managing the car inventory. So, it is not a good idea to create a new object every time using the object literal. In such cases, you need to use the object constructors.
The main benefit of the object constructors is that you can reuse the code.
Syntax
You can follow the syntax below to use the object constructor to create an object.
function Funcname(p1, p2, ... , pN) { this.prop1 = p1; } const obj = new Funcname(args);
In the above syntax, Funcname() is a constructor function, and you can replace any valid identifier with the Funcname.
A p1, p2, …, and pN are parameters you can use inside the function body. You need to pass arguments to the constructor while creating the object.
The 'this' keyword represents the function context you are using. Here, the 'this' keyword refers to the current instance of the object.
To create an object, you can use the function constructor with a 'new' keyword.
Example: Creating an object using a constructor function
In the example below, we have created a Tree() function. In the function body, we initialize the name and age properties.
After that, we use the function name with a 'new' keyword to create an object of the Tree() constructor.
<html> <body> <p id = "output"> </p> <script> function Tree() { this.name = "palm"; this.age = 5; } const tree1 = new Tree(); document.getElementById("output").innerHTML = "name = " + tree1.name + ", age = " + tree1.age; </script> </body> </html>
Output
name = palm, age = 5
Example: Constructor function with parameters
In the example below, the Bike() function takes three parameters. In the function body, we initialize the properties using the parameters.
After that, we created bike1 and bike2 objects with different values using the Bike() constructor. In the output, you can observe the object property values.
<html> <body> <p id = "output1">The bike1 object is : </p> <p id = "output2">The bike2 object is : </p> <script> function Bike(name, speed, gear) { this.name = name; this.speed = speed; this.gear = gear; } const bike1 = new Bike("Honda", 100, 4); const bike2 = new Bike("Yamaha", 200, 6); document.getElementById("output1").innerHTML += JSON.stringify(bike1); document.getElementById("output2").innerHTML += JSON.stringify(bike2); </script> </body> </html>
Output
The bike1 object is : {"name":"Honda","speed":100,"gear":4} The bike2 object is : {"name":"Yamaha","speed":200,"gear":6}
In this way, you can use the object constructor to reuse the object syntax code and create multiple objects of the same type.
Adding a Property or Method to a Constructor
You learned to add a property of method using the dot or square bracket notation into the object in the Objects chapter. But what if you want to add a property or method to the object constructor?
The object constructor doesn't allow you to add the property or method after defining it. So, you always need to add the required properties and methods while defining it. Let's understand it via the example below.
Example
The below example demonstrates adding methods and properties into the object constructor. We added the three properties and the getFullAddress() method in the houseAddress() constructor function.
Also, we have executed the method by taking the object as a reference.
<html> <body> <p id = "output1">The house object is </p> <p id = "output2">The full address of the house is </p> <script> function HouseAddress(no, street, city) { // Adding properties this.houseNo = no, this.street = street, this.city = city; // Adding a method this.getFullAddress = function () { return this.houseNo + ", " + this.street + ", " + this.city; }; } const house = new HouseAddress(20, "Cooper Square", "New York"); document.getElementById("output1").innerHTML += JSON.stringify(house); document.getElementById("output2").innerHTML += house.getFullAddress(); </script> </body> </html>
Output
The house object is {"houseNo":20,"street":"Cooper Square","city":"New York"} The full address of the house is 20, Cooper Square, New York
If you add the method or property as shown below. It will be added to the particular object but not to the constructor function.
Obj.prop = 20;
Other objects created using the object constructor don't contain the prop property as it is added to the obj object only.
JavaScript Object Prototype
In JavaScript, each object contains the prototype property by default, and the object constructor is also one kind of object. So, you can add properties or methods to the object prototype.
Syntax
You can follow the syntax below to add properties or methods to the object constructor prototype.
obj.prototype.name = value;
In the above syntax, 'obj' is an object constructor in which you need to add a property or method.
The 'name' is a property or method name.
For the property, you can replace a 'value' with an actual value; for the method, you can replace a 'value' with a function expression.
Example
In the example below, we have added the BikeDetails() method to the prototype of the Bike() constructor.
The BikDetails() method can be executed using any object of the Bike() constructor. However, when you print the bike1 and bike2 objects, it won't show you BikeDetails() method as it is added in the prototype.
<html> <body> <p id = "output1">The bike1 details is: </p> <p id = "output2">The bike2 details is: </p> <script> function Bike(name, speed, gear) { this.name = name; this.speed = speed; this.gear = gear; } Bike.prototype.BikeDetails = function () { return this.name + ", " + this.speed + ", " + this.gear + "<br>"; }; const bike1 = new Bike("Honda", 100, 4); const bike2 = new Bike("Yamaha", 200, 6); document.getElementById("output1").innerHTML += bike1.BikeDetails(); document.getElementById("output2").innerHTML += bike2.BikeDetails(); </script> </body> </html>
Output
The bike1 details is: Honda, 100, 4 The bike2 details is: Yamaha, 200, 6
Built-in Object Constructors in JavaScript
JavaScript contains a built-in constructor, which we have listed in the below table.
Sr. No. | Constructor | Description | Example |
---|---|---|---|
1 | Array | To create an array. | new Array() |
2 | String | To create a string. | new String("Hello") |
3 | Number | To create a number. | new Number(92) |
4 | Boolean | To create a boolean. | new Boolean(true) |
5 | Set | To create a new set. | new Set() |
6 | Map | To create a new map. | new Map() |
7 | Object | To create a new object. | new Object() |
8 | Function | To create a new function. | new Function("x", "return x * x;") |
9 | Date | To create an instance of Date object. | new Date() |
10 | RegExp | To create a new regular expression. | new RegExp("\\d+") |
11 | Error | To create a new error. | new Error("new error.") |
12 | Symbol | To create a symbol. | Symbol("description") |
However, you can also use literal expressions to define the number, strings, boolean, etc., variables containing primitive values.