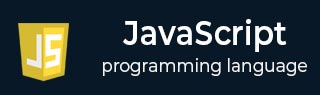
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Performance
JavaScript is very important in every website today. If JavaScript fails, our website wouldn't work properly. It is important to focus on the performance on JavaScript as it has impacts on delivering a positive user experience, retaining engagement, and ensuring business success. An optimized JavaScript would ensure the pages load faster and responsive interactions contribute to users satisfaction and hence higher conversion rates as well as higher search engine rankings (as the SEO would be better).
In a competitive digital landscape, optimized JavaScript code not only attracts and retains users but also enhances resource efficiency, reducing server costs and providing a competitive advantage. With the increasing prevalence of mobile devices and the emphasis on Progressive Web Apps, performance optimization is not only a user expectation but a strategic necessity for businesses aiming to stand out and thrive in the online space.
Optimizing DOM Manipulation
DOM manipulation is where each element is represented as a node in the tree and accessed or modified via their ids, class names etc. These DOM manipulation operations should be minimized as much as possible and would have a significant impact on the speed of our application. Let us consider the below example where we are dynamically creating a list of 1000 items.
Example
Naive Code
<!DOCTYPE html> <html> <body> <ul id="itemList"></ul> <script> const itemList = document.getElementById('itemList'); // Inefficient DOM manipulation for (let i = 0; i < 1000; i++) { itemList.innerHTML += `<li>Item ${i}</li>`; } </script> </body> </html>
Optimized Code
<!DOCTYPE html> <html> <body> <ul id="itemList"></ul> <script> // Efficient DOM manipulation using document fragment const itemList = document.getElementById('itemList'); const fragment = document.createDocumentFragment(); for (let i = 0; i < 1000; i++) { const listItem = document.createElement('li'); listItem.textContent = `Item ${i}`; fragment.appendChild(listItem); } itemList.appendChild(fragment); </script> </body> </html>
Asynchronous Operations with Promises
To prevent the main thread from blocking, we must prioritize asynchronous programming. Consider this example: data retrieval from an API using Promises; in such instances, a Promise proves indispensable for managing asynchronous data fetching. Preventing the UI from freezing during operation; enhancing the overall user experience: these are the benefits.
Example
<!DOCTYPE html> <html> <body> <h2>Asynchronous Operations with Promises</h1> <div id="dataContainer"></div> <script> // Fetch data asynchronously function fetchData() { return new Promise((resolve, reject) => { // Simulating an API call with a delay setTimeout(() => { const data = { message: 'Hello, world!' }; resolve(data); }, 1000); }); } // Usage of the fetchData function fetchData() .then(data => { document.getElementById('dataContainer').textContent = data.message; }) .catch(error => { alert('Error fetching data:', error); }); </script> </body> </html>
Deferred JavaScript Loading
JavaScript is normally loaded when the page is opened, however by delaying the loading of JavaScript we can optimize page load performance especially when dealing with non-essential scripts. When browsers come across the <script> tag, they normally block the rendering until the script has finished downloading and executing. However, by using the defer attribute in the script tag, we can instruct the browser to continue downloading the script in the background while it continues to parse & render the HTML. The script is then executed after the HTML is completely parsed.
Example
<!DOCTYPE html> <html> <body> <p>Deferred JavaScript Loading</p> <script defer src="https://code.jquery.com/jquery-3.6.4.min.js"></script> <div id="loadingMessage">JavaScript is loading...</div> <script> // Add an event listener to indicate when the JavaScript is fully loaded document.addEventListener('DOMContentLoaded', function() { document.getElementById('loadingMessage').textContent = 'JavaScript has finished loading!'; }); </script> </body> </html>
Another option to the above code is to use the async attribute. While similar to defer, async loads the script asynchronously, but it doesn't guarantee the order of execution. The script that finishes loading first will be executed first.
Avoiding Global Variables
Global variables are those which are visible throughout the code, or have a big scope. It is import to understand if our code actually requires the scope of variables to be global or can it be managed efficiently by code encapsulation. The below examples demonstrates the usage of encapsulation to avoid global variables hence leading to better performance of the script.
Example
<!DOCTYPE html> <html> <body> <h2>Avoiding Global Variables</h2> <div id="result"></div> <script> var resultElement = document.getElementById('result'); // Inefficient use of global variables var globalVar = 10; function addNumbers(x, y) { return globalVar + x + y; } resultElement.innerHTML += "Using global variable, the sum is " + addNumbers(51, 7) + "<br>"; // Improved encapsulation (function () { var localVar = 10; function addNumbers(x, y) { return localVar + x + y; } resultElement.innerHTML += "Using encapsulation, the sum is " + addNumbers(51, 7); })(); </script> </body> </html>
While we have discussed performance optimization in this tutorial from a JavaScript point of view, it is important to note that certain optimizations remain constant irrespective of the language. This includes scenarios like using switch case instead of length if else if, memory management, concurrency, etc.
The performance optimization of JavaScript has impacts across industries & applications. In ecommerce, a high speed page load has proven to enhance user engagement and boost conversation rates, while in social media JavaScript has helped in providing seamless interactions. News and media related websites benefit as the content gets updated quickly and only learning platforms (edtech industry) requires JavaScript for interactive educational components.
On the other hand, financial applications, collaboration tools, gaming websites, and healthcare apps all demand optimized JavaScript to ensure their interfaces are responsive and process data in a timely manner.