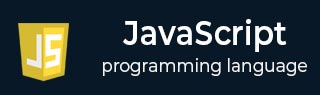
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
Javascript - Features
JavaScript Features
JavaScript is a highly popular and widely-used programming language for web development. It has a variety of features that make it powerful and flexible. Some of these features include being dynamic, lightweight, interpreted, functional, and object-oriented.
Many open-source JavaScript libraries are available, facilitating the utilization of JavaScript in both frontend as well as backend development. Let's highlight some of the key features of JavaScript.
Easy Setup
We don’t need a particular editor to start writing the JavaScript code. Even anyone can write JavaScript code in NotePad. Also, JavaScript can be executed in the browser without any interpreter or compiler setup.
You can use the <script > tag to add JavaScript in the HTML file. However, it also allows you to add JavaScript to the web page from the external JavaScript file, having '.js' extension.
Browser Support
All browsers support JavaScript, as all modern browser comes with the built-in JavaScript execution environment.
However, you can also use the ‘window’ object to check whether the browser supports JavaScript or its particular feature.
Dom Manipulation
JavaScript allows developers to manipulate the webpage elements. Also, you can control the browser.
It contains the various methods to access the DOM elements using different attributes and allows to customize the HTML elements.
Event Handling
JavaScript allows you to handle the events used to interact with the web page.
For example, you can detect the mouse click on a particular HTML element using JavaScript and interact with the HTML element.
Some other events also exist, like detecting the scrolling behavior of web page, etc. We will explore all events in the ‘JavaScript events’ chapter.
Dynamic Typing
JavaScript decides the type of variables at runtime. So, we don’t need to care about variable data type while writing the code, providing more flexibility to write code.
Also, you can assign the values of the different data types to a single variable. For example, if you have stored the number value of a particular variable, you can update the variable’s value with the string.
Functional Programming
JavaScript supports the functional programming. In JavaScript, you can define the first-class function, pure functions, closures, higher-order functions, arrow funcitons, function expresions, etc.
It mostly uses the functions as a primary building blocks to solve the problem.
Cross-platform Support
Each operating system and browser support JavaScript. So, it is widely used for developing websites, mobile applications, games, desktop applications, etc.
Object-oriented Programming
JavaScript contains the classes, and we can implement all object-oriented programming concepts using its functionality.
It also supports inheritance, abstraction, polymorphism, encapsulation, etc, concepts of Object-oriented programming.
Built-in Objects
JavaScript contains built-in objects like Math and Date. We can use a Math object to perform mathematical operations and a Date object to manipulate the date easily.
However, you can also manipulate the functionality of the built-in object.
Object Prototypes
In JavaScript, everything is an object. For example, array, function, number, string, boolean, set, map, etc. are objects.
Each object contains the prototype property, which is hidden. You can use the prototype property to achive inheritance or extend the functionality of class or object, by other object’s functionality.
Global Object
JavaScript contains the global object to access the variables which are available everywhere.
To access global variables in the browser, you can use the window object, and in Node.js, you can use the 'global' keyword to access global variables.
Recently, globalThis keyword is introduced to access the global variables, and which is supported by the most runtime environments.
Built-in Methods
JavaScript also contains the built-in methods for each object. Developers can use the built-in methods to write efficient and shorter codes.
For example, the Array object contains the filter() method to filter array elements and the sort() method to sort the array. The String object contains the replace() method to replace text in the string, the trim() method to remove whitespaces from the string, etc.
Modular Programming
JavaScript allows you to write the code in different modules and connect them with the parent module. So developers can write maintainable code.
By writing the code in a separate module, you can reduce the complexity of the code and reuse each module whenever you require.
JSON
JSON stands for JavaScript object notation. It is a widely used data format to exchange data between two networks. For example, server and client.
JavaScript also supports the JSON format to store the data.
Asynchronous Programming
JavaScript is a single-threaded programming language. To execute your code faster, you can use asynchronous programming.
You can use promises in JavaScript to write asynchronous code, allowing us to handle multiple tasks asynchronously.
Even-driven Architecture
The event-driven architecture of JavaScript allows developers to create interactive and responsive web applications by handling a large user base concurrently.
Due to the vast features and applications of JavaScript, the front end of Facebook is built on JavaScript. Netflix is built using the ReactJS framework of JavaScript. Similarly, Amazon, PayPal, Airbnb, LinkedIn, Twitter, etc., are also built using JavaScript.
Server-side Support
The Node.js runtime environment of JavaScript is widely used to create the backend of the application, as javaScript can also be used to create servers. It allows you to create a scalable backend for the application.