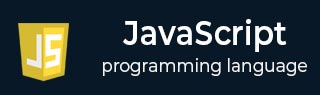
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Web API
A Web API is an application programming interface (API) for web. The concept of the Web API is not limited to JavaScript only. It can be used with any programming language. Let’s learn what web API is first.
What is Web API?
The API is an acronym for the Application Programming Interface. It is a standard protocol or set of rules to communicate between two software components or systems.
A web API is an application programming interface for web.
The API provides an easy syntax to use the complex code. For example, you can use the GeoLocation API to get the coordinates of the users with two lines of code. You don’t need to worry about how it works in the backend.
Another real-time example you can take is of a power system at your home. When you plug the cable into the socket, you get electricity. You don’t need to worry about how electricity comes into the socket.
There are different types of web APIs, some are as follow −
Browser API (Client-Side JavaScript API)
Server API
Third Party APIs
Let’s discuss each of the above type of web APIs in detail −
Browser API (Client-side JavaScript API)
The browser APIs are set of Web APIs that are provided by the browsers.
The browser API is developed on top of the core JavaScript, which you can use to manipulate the web page's functionality.
There are multiple browser APIs available which can be used to interact with the web page.
Following is a list of common browser APIs −
Storage API − It allows you to store the data in the browser's local storage.
DOM API − It allows you to access DOM elements and manipulate them.
History API − It allows you to get the browser’s history.
Fetch API − It allows you to fetch data from web servers.
Forms API − It allows you to validate the form data.
Server API
A server API provides different functionalities to the web server. Server APIs allow developers to interact with server and access data and resources.
For example, REST API is a server API that allows us to create and consume the resources on the server. A JSON API is popular API for accessing data in JSON format. The XML API is a popular API for accessing data in XML format.
Third-party APIs
The third-party API allows you to get the data from their web servers. For example, YouTube API allows you to get the data from YouTube’s web server.
Here is the list of common third-party APIs.
YouTube API − It allows you to get YouTube videos and display them on the website.
Facebook API − It allows you to get Facebook posts and display them on the website.
Telegram API − It allows you to fetch and send messages to the telegram.
Twitter API − It allows you to get tweets from Twitter.
Pinterest API − It allows you to get Pinterest posts.
You can also create and expose API endpoints for your own software. So, other applications can use your API endpoints to get the data from your web server.
Fetch API: An Example of Web API
Here is an example of how to use the fetch API. In the below example, we Fetch API to access data from a given URL ('https://jsonplaceholder.typicode.com/todos/5). The fetch() method returns a promise that we handle using the “then” block. First, we convert the data into the JSON format. After that, we convert the data into the string and print it on the web page.
<html> <body> <h3> Fetch API: An Example of Web API </h3> <div id = "output"> </div> <script> const URL = 'https://jsonplaceholder.typicode.com/todos/5'; fetch(URL) .then(res => res.json()) .then(data => { document.getElementById('output').innerHTML += "The data accessed from the API: " + "<br>" + JSON.stringify(data); }); </script> </body> </html>
JavaScript Web API List
Here, we have listed the most common web APIs.
API | Description |
---|---|
Console API | It is used to interact with the browser’s console. |
Fetch API | It is used to fetch data from web servers. |
FullScreen API | It contains various methods to handle HTML elements in full-screen mode. |
GeoLocation API | It contains the methods to get the user’s current location. |
History API | It is used to navigate in the browser based on the browser’s history. |
MediaQueryList API | It contains methods to query media. |
Storage API | It is used to access the local and session storage. |
Forms API | It is used to validate the form data. |
In upcoming chapters, we will cover the above APIs in details/>