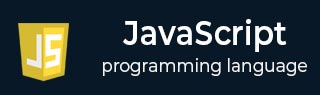
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Grouping Operator
JavaScript Grouping Operator
The grouping operator in JavaScript controls the precedence of the evaluation in expressions. It is denoted by parenthesis (), and you can put the expression inside that to change the expression evaluation order. It helps to evaluate an expression with lower precedence before an expression with higher precedence.
Syntax
You can follow the syntax below to use the grouping operator –
( exp )
In the above syntax, the 'exp' is an expression to change the precedence of evaluation.
let res1 = 4 + 5 * 6; // 4 + 30 let res2 = (4 + 5) * 6; // 9 + 6
The multiplication (*) operator in JavaScript has higher precedence than the addition (+) operator. So, when the above code evaluates the first expression, it will multiply 5 and 6 and add the resultant value to 4.
In the second expression, we grouped the (4 + 5) expression using the grouping operator to provide it with higher precedence than the normal operator. So, it adds 4 and 5 first and multiplies the resultant value with 6.
Example
<html> <body> <div id="output"></div> <script> let res1 = 4 + 5 * 6; let res2 = (4 + 5) * 6; document.getElementById("output").innerHTML = "Result 1: " + res1 + "<br>" + "Result 2: " + res2; </script> </body> </html>
It will produce the following result −
Result 1: 34 Result 2: 54
Immediately Invoked Function Expressions (IIFEs)
To define an immediately invoked function in JavaScript, we use the grouping operator. The anonymous function definition is put inside the grouping operator. These functions are also called self-executing functions.
(function () { return 5; })();
In the above example, the function definition "function () { return 5;}" in put inside the grouping operator.
Example
In the example below, we have defined the self-executing function statement using the grouping operator.
The first expression divides 10 by 5 (returned value from the function) and adds the resultant value, which is 2 to 5.
The second expression adds 5 and 10 first and divides the resultant value with the value returned from the function.
<html> <body> <div id="output"></div> <script> let ans1 = 5 + 10 / (function () { return 5; })(); let ans2 = (5 + 10) / (function () { return 5; })(); document.getElementById("output").innerHTML = "Result 1: " + ans1 + "<br>" + "Result 2: " + ans2; </script> </body> </html>
It will produce the following result −
Result 1: 7 Result 2: 3
In simple terms, the grouping operator is used to group the sub-expressions to change its evaluation precedence over the normal precedence.
Grouping Operator with Logical Operators
The grouping operator in JavaScript can also be used to group expressions with logical operators. For example, in the following expression, the && operator has a higher precedence than the ||operator. So, the expression will be evaluated as follows:
false && false || true; // true
However, if we add parentheses around the ||operator, the expression will be evaluated as follows:
false && (false || true); //false
This is because the grouping operator overrides the normal operator precedence, so that the ||operator is evaluated first.
Example
This example demonstrates that how a grouping operator changes the precedence of OR operator to be evaluated before AND operator. The expression uses the logical operators.
<html> <body> <div id="output"></div> <script> let res1 = false && false || true // true let res2 = false && (false || true) //false document.getElementById("output").innerHTML = "Result without grouping operator: " + res1 + "<br>" + "Result with grouping operator: " + res2; </script> </body> </html>
It will produce the following result −
Result without grouping operator: true Result with grouping operator: false