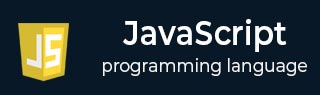
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - let Statement
What is JavaScript let statement?
The JavaScript let statement is used to declare a variable. With the let statement, we can declare a variable that is block-scoped. This mean a variable declared with let is only accessible within the block of code in which it is defined.
The let keyword was introduced in the ES6 (2015) version of JavaScript. It is an alternative to the var keyword.
The main reason behind introducing the let keyword is to improve the scoping behaviors of variables and the safety of the code.
Variable Declaration with let statement
Following is the syntax to declare a variable with let statement −
let var_name = value
Let's have a look at some examples for variable declaration with let.
let name = "John"; let age = 35; let x = true;
Using let statement we can declare a variable of any datatypes, e.g., numeric, string, boolean, etc.
JavaScript Block Scope vs. Function Scope
The scope of the variable declared with the let keyword is a block-scope. It means if you define the variable with the let keyword in the specific block, you can access the variable inside that particular block only, and if you try to access the variable outside the block, it raises an error like 'variable is not defined'.
{ let x = "John"; } //here x can't be accessed
The var keyword has a function scope, meaning if you define the variable using the var keyword in any function block, you can access it throughout the function.
function foo(){ if (true){ let x = 5 var y = 10 } // here x can't be accessed while y is accessible }
Sometimes, we require to define the variable with the same name in different blocks of one function. Conflicts may occur with the variable value if they use the var keyword.
Example
In the example below, we have defined the variable x using the let keyword and variable y using the var keyword. Also, we have assigned 10 and 20 values to both variables, respectively.
We defined the test() function, redeclared the x and y variables inside it, and initialized them with 50 and 100 values, respectively. We print variable values inside the function, and it prints the 50 and 100 as it gives first preference to the local variables over global variables.
<html> <head> <title> Variable declaration with let keyword </title> </head> <body> <script> let x = 10; var y = 20; function test() { let x = 50; var y = 100; document.write("x = " + x + ", y = " + y + "<br/>"); } test(); </script> </body> </html>
Example
In the example below, we initialized the bool variable with a 'true' value. After that, we declared the variables x and y using the let and var keywords in the 'if' block.
We print the value of the x and y variable inside the 'if' block. We can't access the 'x' variable outside the 'if' block as it has blocked scope, but we can access variable y outside the 'if' block and inside the function block as it has function scope.
<html> <head> <title> Variable declaration with let keyword </title> </head> <body> <script> function test() { let bool = true; if (bool) { let x = 30; var y = 40; document.write("x = " + x + ", y = " + y + "<br/>"); } // x can't be accessible here document.write("y = " + y + "<br/>"); } test(); </script> </body> </html>
In this way, the let keyword is used to improve the scoping behaviors of the code.
Redeclaring Variables in JavaScript
You can't redeclare the variables declared with the let keyword in the same block. However, you can declare the variables with the same name into the different blocks with the same function.
Example
In the example below, you can observe that variables declared with the let keyword can’t be redeclared in the same block, but variables declared with the var keyword can be redeclared in the same block.
The code prints the value of the newly declared variable in the output.
<html> <head> <title> Variable redeclaring </title> </head> <body> <script> function test() { if (1) { let m = 70; // let m = 80; // redeclaration with let keyword is not possible var n = 80; var n = 90; // redeclaration with var keyword is possible document.write("m = " + m + ", n = " + n); } } test(); </script> </body> </html>
Variable Hoisting
The hoisting behaviors of JavaScript move the declaration of the variables at the top of the code. The let keyword doesn't support hoisting, but the var keyword supports the hosting.
Example
In the example below, you can see that we can initialize and print the value of the variable n before its declaration as it is declared using the var keyword.
<html> <head> <title> Variable hoisting </title> </head> <body> <script> function test() { // Hoisiting is not supported by let keyword // m = 100; // document.write("m = " + m + "<br/>"); // let m; n = 50; document.write("n = " + n + "<br/>"); var n; } test(); </script> </body> </html>
You can uncomment the code using the let keyword and check the error in the web console, as it doesn't support hoisting.