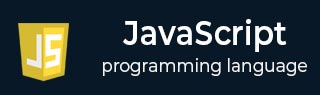
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Tempate Literals
JavaScript Tempate Literals
In JavaScript, the template literals are introduced in ES6 to customize the string dynamically. The template literals allow you to add variables or expressions into the string, and the string changes according to the variables and expression value changes.
There are multiple synonyms words used for the template literal words. For example, template string, string templates, back-tics syntax, etc.
Syntax
Follow the syntax below to use the template literals in JavaScript.
let str = `Hi ${name}`;
You need to write a string between back-tics (``). For using the dynamic variable or expression with a string, you need to place it between the ${}.
Furthermore, using the template literals, you don’t need escape characters to add single or double quotes in the string.
Examples
Example: Creating Strings with Template Literals
In the example below, we used the template literals to create a string with special characters.
The str1 string is the same as the regular string we create using single or double quotes. The str2 string contains the single quotes with the string. Here, you can see that we haven’t used the escape characters to add a single quote in the string.
<html> <body> <div id = "output1"> </div> <div id = "output2"> </div> <script> let str1 = `Hello Users!`; let str2 = `'Tutorialspoint' is a good website`; document.getElementById("output1").innerHTML = str1; document.getElementById("output2").innerHTML = str2; </script> </body> </html>
Output
Hello Users! 'Tutorialspoint' is a good website
Example: Variables with Template Literals
The below code demonstrates to use the dynamic values in the string by passing the variables into the template literal string.
Here, we have defined the variables related to cars. After that, we create a string using the template literals and add the variables.
In the output, you can see that variables are replaced with their value in the string.
<html> <body> <p id = "output"> </p> <script> let car = "BMW"; let model = "X5"; const price = 5000000; const carStr = `The price of the ${car} ${model} is ${price}.`; document.getElementById("output").innerHTML = carStr; </script> </body> </html>
Output
The price of the BMW X5 is 5000000.
Example: Expressions with Template Literals
You can also add the expressions to the string using the template literals.
In the str1 string, we added the expression to perform the summation of two numbers in the template literals string.
In str2, we invoke the function invocation as an expression. It replaces the expression with a returned value from the function.
<html> <body> <div id = "output1"> </div> <div id = "output2"> </div> <script> function func() { return 10; } const str1 = `The sum of 2 and 3 is ${2 + 3}.`; const str2 = `The return value from the function is ${func()}`; document.getElementById("output1").innerHTML = str1; document.getElementById("output2").innerHTML = str2; </script> </body> </html>
Output
The sum of 2 and 3 is 5. The return value from the function is 10
JavaScript Nested Template Literals
JavaScript allows you to use the template literals inside other template literals, and it is called the nested template literals.
Example
In the example below, we added the expression in the outer template literal. The expression contains the ternary operator. It checks whether the 2 is less than 3. Based on the returned boolean value, it executes the first or second nested expression and prints the result.
<html> <head> <title>Nested Template Literals</title> </head> <body> <p id = "output"> </p> <script> const nested = `The subtraction result is: ${2 < 3 ? `${3 - 2}` : `${2 - 3}`}`; document.getElementById("output").innerHTML = nested; </script> </body> </html>
Output
The subtraction result is: 1
Example: Multiline String Using Template Literal
You can also use the template literals to define the multiline string.
<html> <body> <div id = "output"> </div> <script> function func() { return 10; } const str1 = `The sum of 2 and 3 is ${2 + 3}. <br> The return value from the function is ${func()}`; document.getElementById("output").innerHTML = str1; </script> </body> </html>
Output
The sum of 2 and 3 is 5. The return value from the function is 10