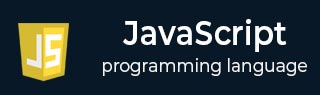
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - ECMAScript 2018
The ECMAScript 2018 version of JavaScript was released in 2018. ECMAScript 2017 introduced singnificant enhancement to the language. Two important features introduced in this version are asynchronous iteration for improved handling of asynchronous operations, and promise finally() to execute code regardless of promise resolution. This chapter will discuss all the new added features in ECMAScript 2018.
New Added Features in ECMAScript 2018
Here are the new methods, features, etc., added to the ECMAScript 2018 version of JavaScript.
- Asynchronous iteration
- New features of the RegExp() Object
- Promise.finally()
- Rest object properties
Here, we have explained each feature in detail.
JavaScript Asynchronous Iteration
You can also use the 'await' keyword with the for loop to make asynchronous iterations.
For example, you are iterating multiple promises, and in each iteration, you need to stop the code execution until the current promise gets resolved or rejected.
Example
In the below code, we have defined the async generator function named gen_function. The gen_func() function makes 5 iterations using the loop. In each iteration, it awaits to resolve the promise and returns p.
In the test() function, we used the 'await' keyword with the for loop to make asynchronous iterations. It updates the output after every 0.5 seconds.
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); // Generator function async function* gen_function() { for (let p = 0; p < 5; p++) { await new Promise(res => setTimeout(res, 500)); yield p; } } async function test() { for await (const ele of gen_function()) { output.innerHTML += "Returned element is: " + ele + "<br>"; } } test(); </script> </body> </html>
Output
Returned element is: 0 Returned element is: 1 Returned element is: 2 Returned element is: 3 Returned element is: 4
New features of the RegExp() object
In ECMAScript 2018, the following four new regular expression features were introduced −
- Unicode Property Escapes (\p{...})
- Lookbehind Assertions (?<= ) and (?<! )
- Named Capture Groups
- s (dotAll) Flag
Unicode Property Escapes (\p{...})
The Unicode property escape allows you to escape the Unicode character. You need to represent the Unicode in the curly braces followed by the '\p'.
Example
In the below code, we use the regular expression to check whether the tet contains the letter using the Unicode property access.
<html> <body> <div id = "output1">regex.test('Y'): </div> <div id = "output2">regex.test('6'): </div> <script> const regex = /\p{Letter}/u; // To Match letters only document.getElementById("output1").innerHTML += regex.test('Y'); // true document.getElementById("output2").innerHTML += regex.test('6'); // false </script> </body> </html>
Output
regex.test('Y'): true regex.test('6'): false
Lookbehind Assertions (?<= ) and (?<! )
The Lookbehind assertion allows you to find a particular subpattern followed by a particular sub patter. The positive look-behind assertion is equal to ?<=, and the negative look-behind assertion is ?<!.
Example
In the below code, we are looking for a word after the '@' using the look behind the assertion. It finds words after the '@' pattern.
<html> <body> <div id = "output">lookBeind.exec('abcd@domain.com')[0]: </div> <script> const lookBeind = /(?<=@)\w+/; document.getElementById("output").innerHTML += lookBeind.exec('abcd@tutorialspoint.com')[0]; // Prints domain </script> </body> </html>
Output
lookBeind.exec('abcd@domain.com')[0]: tutorialspoint
Named Capture Groups
You can give a unique name to each group of the regular expression. The group names make it easy to extract the patterns from the string.
Example
In the code below, we have defined the regular expression to match the date pattern. Also, we have named the groups' year, month, and day.
After that, we extracted the year from the date using the group name.
<html> <body> <div id = "output">The year of the date is: </div> <script> const datePattern = /(?<year>\d{4})-(?<month>\d{2})-(?<day>\d{2})/; const match = datePattern.exec('2023-08-22'); document.getElementById("output").innerHTML += match.groups.year; </script> </body </html>
Output
The year of the date is: 2023
s (dotAll) Flag
The '.' (dot) character matches any character except the new line in a regular expression. If you also want to match the new line using the '.' Character, you need to use the '/s' flag, as shown in the example below.
Example
In the below code, we have added the '.' Character in the regular expression pattern to match any character, and also added \s flag.
In the output, you can see that '.' character also matches with the '\n'.
<html> <body> <div id = "output">strRegex.test('Hello\nprogrammers'): </div> <script> const strRegex = /Hello.programmers/s; document.getElementById("output").innerHTML += strRegex.test('Hello\nprogrammers'); </script> </body> </html>
Output
strRegex.test('Hello\nprogrammers'): true
JavaScript Promise finally()
You can use the finally() block with promises to execute the particular code after the promise gets resolved or rejected. It is similar to the try...catch...finally block.
Example
In the example below, we created the promise and stored it in the getData variable. The promise gets resolved after 1000 milliseconds.
After that, we use the 'then...finally', block to execute the promise. In the output, you can observe that code of the 'finally' block always gets executed.
<html> <body> <div id = "output"> </div> <script> const getData = new Promise((res, rej) => { setTimeout(() => { res("Promise resolved!"); }, 1000); }); getData .then(result => { document.getElementById("output").innerHTML += result + "<br>"; }) .finally(() => { document.getElementById("output").innerHTML += "In the finally block!"; }); </script> </body> </html>
Output
Promise resolved! In the finally block!
JavaScript Rest Object Properties
You can use the spread operator while destructuring the objects. The spread operator allows you to collect the remaining properties in a single variable in the object format.
Example
In the example below, the numbers object contains 4 properties. While destructuring, we get the value of the num1 property and store other properties in the nums variable using the spread operator.
<html> <body> <div id = "output"> </div> <script> const numbers = { num1: 40, num2: 50, num3: 80, num4: 90, } const { num1, ...nums } = numbers; document.getElementById("output").innerHTML = "num1 = " + num1 + "<br>" + "nums = " + JSON.stringify(nums); </script> </body> </html>
Output
num1 = 40 nums = {"num2":50,"num3":80,"num4":90}