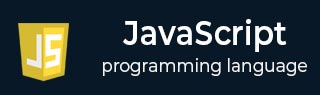
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - ES5
The ES6 version of JavaScript was released in 2015, marking the second major revision of JavaScript. The ES6 is also known as ECMAScript 2015. Some of the important features introduced in ES6 are arrow functions, let and const keywords, Classes, res parameters, etc. This chapter will discuss all the newly added features in ES6 version.
New Added Features in ES6
Here are the new methods, features, etc., added to the ES6 version of JavaScript.
- Arrow Functions
- Array find()
- Array findIndex()
- Array from()
- Array keys()
- Classes
- const keyword
- Default Parameters
- For/of
- Function Rest Parameter
- JavaScript Modules
- let keyword
- Map Objects
- New Global Methods
- New Math Methods
- New Number Methods
- New Number Properties
- Promises
- Set Objects
- String.endsWith()
- String.includes()
- String.startsWith()
- Symbol
- The spread Operator
Here, we have explained each feature in detail with examples.
JavaScript Arrow Functions
The arrow function is a way to write the shorter function code. The concept of the arrow functions allows you to define the function without using the function keyword, curly braces, and return keyword.
Example
In the below code, func() is a regular function, and the variable subtracts stores the arrow function.
<html> <body> <div id = "output">The subtraction of 20 and 10 is: </div> <script> /* Normal function function func(a, b) { return a - b; } */ // Arrow function const subtract = (a, b) => a - b; document.getElementById("output").innerHTML += subtract(20, 10); </script> </body> </html>
Output
The subtraction of 20 and 10 is: 10
JavaScript Array find() Method
The JavaScript array.find() method returns the first element that follows the particular condition.
Example
In the below code, we find the first array element whose length is less than 4 using the array.find() method.
<html> <body> <div id = "output">The first array element whose length is less than 4 is: </div> <script> const strs = ["Hello", "World", "How", "are", "You"]; function func_len(value, index, array) { return value.length < 4; } document.getElementById("output").innerHTML += strs.find(func_len); </script> </body> </html>
Output
The first array element whose length is less than 4 is: How
JavaScript Array findIndex()
The JavaScript array.findIndex() method is similar to the array.find() method, but it returns the index of the first element that matches the particular condition. It returns the 0-based index.
Example
In the below code, we find the index of the first element whose length is less than 4 using the array.findIndex() method.
<html> <body> <div id = "output">The first array element whose length is less than 4 is: </div> <script> const strs = ["Hello", "World", "How", "are", "You"]; function func_len(value, index, array) { return value.length < 4; } document.getElementById("output").innerHTML += strs.findIndex(func_len); </script> </body> </html>
Output
The first array element whose length is less than 4 is: 2
JavaScriipt Array from()
The JavaScript Array.from() method creates an array from the iterable passed as an argument.
Example
In the below code, we create an array from the string using the Array.from() method. However, you can also pass iterable as an argument of the Array.from() method.
<html> <body> <div id = "output">The array from the Hello string is: </div> <script> document.getElementById("output").innerHTML += Array.from("Hello"); </script> </body> </html>
Output
The array from the Hello string is: H,e,l,l,o
JavaScript Array keys()
The JavaScript array.keys() method returns an iterator to iterate the keys. The keys of the array element are indexes of the array elements.
Example
In the below code, we use the keys() method to get the iterators of keys of the nums[] array. After that, we use the for/of loop to traverse the keys of the array.
<html> <body> <div id = "demo">The keys of the nums array is: <br></div> <script> const output = document.getElementById("demo"); const nums = [45, 67, 89, 342, 123, 12]; const iteratorNums = nums.keys(); for (let key of iteratorNums) { output.innerHTML += key + "<br>"; } </script> </body> </html>
Output
The keys of the nums array is: 0 1 2 3 4 5
JavaScript Classes
Classes are essential in the object-oriented programming language. It is a blueprint for the object.
You can use the class keyword to define the class. You can add constructors, properties, and methods to the class body. To access class properties and methods, you can use the class instance.
Example
In the below code, we have defined the animal class.
The constructor initializes the value of the name and isVegetarian property. The getInfo() method returns the animal information.
We created the object of the animal class and used it to invoke the getInfo() method of the class.
<html> <body> <div id = "output">The animal information is: </div> <script> class animal { constructor(name, isVegetarian) { this.name = name; this.isVegetarian = isVegetarian; } getInfo() { return "Name : " + this.name + ", " + "isVegetarian? : " + this.isVegetarian; } } const lion = new animal("Lion", false); document.getElementById("output").innerHTML += lion.getInfo(); </script> </body> </html>
Output
The animal information is: Name : Lion, isVegetarian? : false
JavaScript const keyword
The JavaScript const keyword is used to declare the constant variables. You need to initialize the constant variables while declaring them.
Example
In the below code, the 'fruit' is a constant variable. You can't reinitialize its value.
<html> <body> <div id = "output">The value of the fruit variable is: </div> <script> const fruit = "Apple"; // fruit = "Banana"; This is Invalid document.getElementById("output").innerHTML += fruit; </script> </body> </html>
Output
The value of the fruit variable is: Apple
JavaScript let keyword
The JavaScript let keyword is used to define the blocked scope variables.
Example
In the below code, we have defined the variable 'a' using the let keyword inside the 'if' block. It can't be accessible outside the 'if' block due to its scoping behavior.
<html> <body> <div id = "output"> </div> <script> if (true) { let a = 20; document.getElementById("output").innerHTML += "The value of a is: " + a; } // You can't access it here. </script> </body> </html>
Output
The value of a is: 20
JavaScript Default Parameters
The default parameters mean function parameters can have default values. When you don't pass enough arguments to the function, it uses the default parameter values.
Example
In the below code, the division () function takes two parameters. The default value of a is 10, and b is 2.
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); function division(a = 10, b = 2) { return a / b; } output.innerHTML += "division(40, 5) => " + division(40, 5) + "<br>"; output.innerHTML += "division(40) => " + division(40) + "<br>"; output.innerHTML += "division() => " + division(); </script> </body> </html>
Output
division(40, 5) => 8 division(40) => 20 division() => 5
JavaScript for…of Loop
The JavaScript for…of loop traverses the iterable like an array, string, set, map, etc.
Example
In the below code, we traverse the array of numbers and print each element of the array in the output.
<html> <body> <div id = "output">The array elements are: </div> <script> const array = [10, 67, 82, 75, 80]; for (let number of array) { document.getElementById("output").innerHTML += number + ", "; } </script> </body> </html>
Output
The array elements are: 10, 67, 82, 75, 80,
JavaScript Function Rest Parameter
When you are unsure about a number of the function argument, you can use the rest parameters. The rest parameter allows you to collect multiple arguments in a single array.
Example
We have passed the below code using the rest parameter with the sum() function. The name of the rest parameter can be a valid identifier, and it is used with the spread (…) operator.
The sum() function adds the multiple numeric values and returns them.
<html> <body> <div id = "output">sum(10, 67, 82, 75, 80) = </div> <script> function sum(...restParam) { let res = 0; for (let ele of restParam) { res += ele; } return res; } document.getElementById("output").innerHTML += sum(10, 67, 82, 75, 80); </script> </body> </html>
Output
sum(10, 67, 82, 75, 80) = 314
JavaScript Modules
In JavaScript, you can create different modules to write reusable code. After that, you can import the modules into the different JavaScript files.
Default Export/Import modules
const moduleMath = "This is the default value."; export default moduleMath; // Exporting the module
In other JavaScript files,
// Importing the default module import moduleMath from './filename.js'; console.log(moduleMath);
Named Export/Import modules
You can also export particular functions or properties from the modules and import them into other JavaScript files.
// Exporting variables export const size = 90; // Exporting function export function add(a, b) { return a + b; }
In other JavaScript files,
// Importing specific properties and functions import { size, add} from './filename.js'; console.log(myVariable); // 90 console.log(add(15, 25)); // 40
JavaScript Map Objects
The map is used to store the key-value pairs. You can use the Map() constructor to define a map.
Example
In the example below, we use the map to store the fruit's name and price. The set() method is used to insert the key-value pair into the fruit map, and the get() method is used to get the value of a particular key from the map.
<html> <body> <div id = "output1">The price of the Apple is: </div> <div id = "output2">The price of the Banana is: </div> <script> const fruit = new Map(); fruit.set("Apple", 50); fruit.set("Banana", 60); document.getElementById("output1").innerHTML += fruit.get("Apple") + "<br>"; document.getElementById("output2").innerHTML += fruit.get("Banana"); </script> </body> </html>
Output
The price of the Apple is: 50 The price of the Banana is: 60
New Global Methods
In the ES6, below two global methods are added.
- isFinite()
- isNaN()
isFinite()
The isFinite() method checks whether the value passed as an argument is finite.
Example
In the below code, the num1 variable contains the infinity value, and num2 contains the valid numeric value.
We used the isFinite() method to check whether the num1 and num2 variable's value is finite.
<html> <body> <div id = "output"> </div> <script> const num1 = 6453 / 0; const num2 = 90; document.getElementById("output").innerHTML = "isFinite(6453 / 0): " + isFinite(num1) + "<br>" + "isFinite(90): " + isFinite(num2); </script> </body> </html>
Output
isFinite(6453 / 0): false isFinite(90): true
isNaN()
The isNaN() method checks whether the argument is a valid number. It returns false for the number value.
Example
In the below code, the isNaN() method returns true for the num1 variable, as it contains the string, and the string is not a number. For the num2 variable, the isNaN() method returns false, as it contains the numeric value.
<html> <body> <div id = "output"> </div> <script> const num1 = "Hello"; const num2 = 867; document.getElementById("output").innerHTML = "isNaN(num1): " + isNaN(num1) + "<br>" + "isNaN(num2): " + isNaN(num2); </script> </body> </html>
Output
isNaN(num1): true isNaN(num2): false
New JavaScript Math Methods
In ES6, 5 new methods were added to the Math object.
- Math.cbrt() − It is used to find the cube root of the given number.
- Math.log2() – It is used to find the logarithm of a number and uses the base 2.
- Math.log10() – It finds the base 10 logarithm of numeric value.
- Math.trunc() – It removes the decimal part from the floating point number and converts it into the whole number.
- Math.sign() – It returns 1, 0, and -1 based on the sign of the number passed as an argument.
Example: Math.cbrt()
The below code finds the cube root of the 64.
<html> <body> <div id = "output">The cube root of the 64 is: </div> <script> document.getElementById("output").innerHTML += Math.cbrt(64); </script> </body> </html>
Example: Math.log2()
The below code finds the logarithm of 30 base 2.
<html> <body> <div id = "output">The value of logarithm of 30 base 2 is: </div> <script> document.getElementById("output").innerHTML += Math.log2(30); </script> </body> </html>
Example: Math.log10()
The below code finds the logarithm of 10 base 10.
<html> <body> <div id = "output">The value of the logarithm of 10 base 10 is: </div> <script> document.getElementById("output").innerHTML += Math.log10(10); </script> </body> </html>
Example: Math.trunc()
The below code truncates the floating point numbers using the Math.trunc() method.
<html> <body> <div id = "output">After converting 23.2 to integer is: </div> <script> document.getElementById("output").innerHTML += Math.trunc(23.2); </script> </body> </html>
Example: Math.sign()
<html> <body> <div id="output1">Math.sign(23): </div> <div id="output2">Math.sign(-23): </div> <script> document.getElementById("output1").innerHTML += Math.sign(23); document.getElementById("output2").innerHTML += Math.sign(-23); </script> </body> </html>
New Number Methods
In ES6, two new number methods were added.
Number.isInteger() − It checks whether the number passed as an argument is a whole number or an integer.
Number.isSafeInteger() − It checks whether the number can be represented as a 64-bit double number.
Example
The below code checks whether 10 and 10.5 is an integer value. Also, it uses the isSafeInteger() method of the number class to check whether the number is safe integer.
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); output.innerHTML += "Is 10 integer? " + Number.isInteger(10) + "<br>"; output.innerHTML += "Is 10.5 integer? " + Number.isInteger(10.5) + "<br>"; output.innerHTML += "Is 10000 safe integer? " + Number.isSafeInteger(10000) + "<br>"; output.innerHTML += "Is 10000000000000000000000 safe integer? " + Number.isSafeInteger(10000000000000000000000); </script> </body> </html>
Output
Is 10 integer? true Is 10.5 integer? false Is 10000 safe integer? - true Is 10000000000000000000000 safe integer? - false
New Number Properties
In ES6, three new number properties were added.
EPSILON − It returns the value of the Epsilon.
MIN_SAFE_INTEGER − It returns the minimum integer value that a 64-bit number can represent.
MAX_SAFE_INTEGER − returns the maximum number, which can be represented by 64-bit.
Example
The below code shows the value of the Epsilon constant, minimum value of the safe integer, maximum value of the safe integer in JavaScript.
<html> <body> <div id = "output1">The value of Epsilon is: </div> <div id = "output2">The minimum safe integer is: </div> <div id = "output3">The maximum safe integer is: </div> <script> document.getElementById("output1").innerHTML += Number.EPSILON; document.getElementById("output2").innerHTML += Number.MIN_SAFE_INTEGER; document.getElementById("output3").innerHTML += Number.MAX_SAFE_INTEGER </script> </body> </html>
Output
The value of Epsilon is: 2.220446049250313e-16 The minimum safe integer is: -9007199254740991 The maximum safe integer is: 9007199254740991
JavaScript Promises
In JavaScript, promises are used to handle the code asynchronously.
It produces and consumes the code.
Example
In the below code, we used the Promise() constructor to create a promise. We resolve and reject the promise based on the random value generated using the random() method of the Math block.
After that, we handle the promise using the then() and catch() block.
<html> <body> <div id = "output"> </div> <script> // Creating a Promise const newPromise = new Promise((res, rej) => { setTimeout(() => { const rand_value = Math.random(); if (rand_value < 0.5) { res("Value is less than 0.5"); // Resolving the promise } else { rej("Value is greater than 0.5"); // Rejecting the promise } }, 1000); // Adding 1 second delay }); // Consuming the Promise newPromise .then((res) => { document.getElementById("output").innerHTML += res; }) .catch((rej) => { document.getElementById("output").innerHTML += rej; }); </script> </body> </html>
Output
Value is greater than 0.5
JavaScript Set Objects
The Set() constructor is used to create a set. The set stores only unique elements of different types.
Example
In the below code, we created a new set and passed the array containing the number as an argument of the Set() constructor. The set contains only unique elements, which you can see in the output.
<html> <body> <div id = "output">The set elements are: </div> <script> const num_set = new Set([10, 20, 20, 42, 12]); for (let num of num_set) { document.getElementById("output").innerHTML += ", " + num; } </script> </body> </html>
Output
The set elements are: , 10, 20, 42, 12
JavaScript New String Methods
In ES6, three new string methodswere added.
- endsWith() − checks whether the string ends with a particular substring.
- includes() − checks whether the string contains the substring at any position.
- startsWith() − checks whether the string starts with a particular substring.
Example
The example below demonstrates how to use String endsWith(), includes(), and startsWith() methods with the help of an staing – "How are you? I'm fine!".
<html> <body> <div id = "output1">Does string end with 'fine'? </div> <div id = "output2">Does string include 'are'? </div> <div id = "output3">Does string start with 'How'? </div> <script> let str = "How are you? I'm fine!"; document.getElementById("output1").innerHTML += str.endsWith("fine!"); document.getElementById("output2").innerHTML += str.includes("are"); document.getElementById("output3").innerHTML += str.startsWith("How"); </script> </body> </html>
Output
Does string end with 'fine'? true Does string include 'are'? true Does string start with 'How'? true
JavaScript Symbol
The JavaScript Symbol is a primate data type in JavaScript. In JavaScript, each Symbol is unique. You may use it to create unique ids.
Example
In the code below, we have defined two symbols and passed the same value as an argument. Still, both symbols are unique, which you can see in the output.
<html> <body> <div id = "output"> </div> <script> const sym1 = Symbol("a"); const sym2 = Symbol("a"); if (sym1 == sym2) { document.getElementById("output").innerHTML += "sym1 and sym2 are equal. <br>"; } else { document.getElementById("output").innerHTML += "sym1 and sym2 are not equal."; } </script> </body> </html>
Output
sym1 and sym2 are not equal.
JabaScript Spread Operator
The JavaScript spread operator allows you a create a copy of the iterable, like array, string, etc.
Example
The below code demonstrates using the spread operator to create an array of characters from the string.
<html> <body> <div id = "output">The char array is: </div> <script> let str = "Hello World!"; const char = [...str]; document.getElementById("output").innerHTML += char; </script> </body> </html>
Output
The char array is: H,e,l,l,o, ,W,o,r,l,d,!