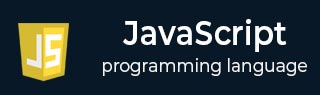
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Object Destructuring
Object Destructuring
The object destructuring assignments are JavaScript expressions that allow you to unpack and assign object properties into individual variables. The name of the individual variables can be the same as the object properties or different.
The object destructuring is a very useful feature when you have an object with a lot of properties and you only need a few of them.
Syntax
The syntax of Object Destructing assignment in JavaScript is as follows –
const { prop1, popr2 } = obj; OR const { prop1: p1, prop12: p2 } = obj; // Renaming variables OR const { prop1 = default_vaule } = obj; // With Default values OR const { prop1, ...prop2 } = obj; // With rest parameter
In the above syntax, 'obj' is an object. The prop1 and prop2 are object properties. It covers the different use cases of object destructuring.
Example: Basic Object Destructuring
In the example below, the watch object contains the brand and price properties.
We store the values of the object properties into the individual variables using object destructuring. You can see the brand's value and price variable in the output, which is the same as object property values.
<html> <body> <p id = "output"> </p> <script> const watch = { brand: "Titan", price: 6000, } const {brand, price} = watch; document.getElementById("output").innerHTML += "The brand of the watch is " + brand + " and the price is " + price; </script> </body> </html>
Output
The brand of the watch is Titan and the price is 6000
Example: Destructuring with less properties
The code below demonstrates that you can unpack only required object properties and keep others as it is. Here, the Object contains total 4 properties. But we have unpacked only brand and price properties.
<html> <body> <p id = "output"> </p> <script> const watch = { brand: "Titan", price: 6000, color: "Pink", dial: "Round", } const { brand, price } = watch; document.getElementById("output").innerHTML = "The brand of the watch is " + brand + " and the price is " + price; </script> </body> </html>
Output
The brand of the watch is Titan and the price is 6000
Object Destructuring and Renaming Variables
In JavaScript object destructuring, it is not necessary to store the object property values in the variables with the same name as object properties.
You can write a new variable name followed by a colon followed by an object property name. In this way, you can rename the object properties while destructuring the object.
Example
In the example below, we have stored the value of the brand property in the 'bd' variable, the color property in the 'cr' variable, and the dial property in the 'dl' variable.
The values of the new variables are the same as the object properties.
<html> <body> <p id = "output1">brand: </p> <p id = "output2">color: </p> <p id = "output3">dial: </p> <script> const watch = { brand: "Titan", color: "Pink", dial: "Round", } const { brand: bd, color: cr, dial: dl } = watch; document.getElementById("output1").innerHTML += bd; document.getElementById("output2").innerHTML += cr; document.getElementById("output3").innerHTML += dl; </script> </body> </html>
Output
brand: Titan color: Pink dial: Round
Object Destructuring and Default Values
In many situations, an object property may contain an undefined value, or particular property doesn't exist in the object. If the property is undefined, JavaScript destructuring assignment allows you to initialize the variables with default values.
Example
In the below code, the animal object contains the name and age properties.
We destructure the object and try to get the name and color property values from the object. Here, the color property doesn't exist in the object, but we have initialized it with the default value.
The output shows 'yellow' as the color variable's value, which is the default value.
<html> <body> <p id = "output1">Animal Name: </p> <p id = "output2">Animal Color: </p> <script> const animal = { name: "Lion", age: 10, } const { name = "Tiger", color = "Yellow" } = animal; document.getElementById("output1").innerHTML += name; document.getElementById("output2").innerHTML += color; </script> </body> </html>
Output
Animal Name: Lion Animal Color: Yellow
Example
In the below code, we have renamed the variables and assigned the default values to the variables. We used the colon to change the variable name and the assignment operator to assign the default values.
<html> <body> <p id = "output1">Animal Name: </p> <p id = "output2">Animal Color: </p> <script> const animal = { name: "Lion", age: 10, } const { name: animalName = "Tiger", color: animalColor = "Yellow" } = animal; document.getElementById("output1").innerHTML += animalName; document.getElementById("output2").innerHTML += animalColor; </script> </body> </html>
Output
Animal Name: Lion Animal Color: Yellow
Object Destructuring and Rest Operator
The syntax of the JavaScript Rest parameter is three dots (...). It allows you to collect the remaining object properties into a single variable in the object format. Let's understand it via the example below.
Example
In the below code, the nums object contains the 4 properties. While destructuring, the object value of the num1 property is stored in the num1 variable. Other remaining properties are stored in the 'numbers' variable using the rest operator.
In the output, you can see that 'numbers' contains the object containing the remaining properties of the nums object.
<html> <body> <p id = "output"> </p> <script> let output = document.getElementById("output"); const nums = { num1: 10, num2: 20, num3: 30, num4: 40, } const {num1, ...numbers} = nums; output.innerHTML += "num1: " + num1 + "<br>"; output.innerHTML += "numbers: " + JSON.stringify(numbers); </script> </body> </html>
Output
num1: 10 numbers: {"num2":20,"num3":30,"num4":40}
Object Destructuring and Function Parameter
You can pass the JavaScript object as a function argument. After that, you can destructure the object in the parameter of the function definition.
Example
In the below code, the nums object contains multiple properties, and we have passed it as an argument of the sum() function.
In the function parameter, we destructure the object and use that variable inside the function body. The function body returns the sum of object properties.
<html> <body> <p id = "output"> </p> <script> function sum({ num1, num2, num3, num4 }) { return num1 + num2 + num3 + num4; } const nums = { num1: 5, num2: 7, num3: 10, num4: 12, } const res = sum(nums); document.getElementById("output").innerHTML += "The sum of numbers is: " + res; </script> </body> </html>
Output
The sum of numbers is: 34