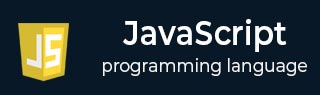
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Changing CSS
Changing CSS with JavaScript
JavaScript allows you to change the CSS of the HTML element dynamically.
When HTML gets loaded in the browser, it creates a DOM tree. The DOM tree contains each HTML element in the object format. Furthermore, each HTML element object contains the 'style' object as a property. Here, a 'style' object contains various properties like color, backgroundcolor, etc., to change or get the element's style.
So, you can use various properties of the 'style' object to change the style of the particular HTML element.
Syntax
Follow the syntax below to change the CSS of the HTML element.
element.style.property = value;
In the above syntax, 'element' is an HTML element, which you need to access from the DOM tree. The 'property' is a CSS property, and the 'value' can be static or dynamic.
Example: Changing the style of the HTML element
We have applied the initial style to the div element in the code below. In JavaScript, we change the div element's background color using the style object's 'backgroundColor' property.
<!DOCTYPE html> <html> <head> <style> div { background-color: blue; width: 700px; height: 100px; color: white; } </style> </head> <body> <div id = "square"> Changing the color of this Div. </div> <br> <button onclick="changeColor()"> Change Color </button> <script> function changeColor() { let square = document.getElementById('square'); square.style.backgroundColor = 'red'; } </script> </body> </html>
Example: Adding multiple styles to a single HTML element
In the below code, we change multiple CSS properties of the <div> element in the changeStyle() function.
We use the 'backgroundColor’, 'color’, 'fontSize’, and 'width' properties to change the CSS.
<!DOCTYPE html> <html> <body> <div id = "square"> Changing the style of this Div. </div> <br> <button onclick = "changeStyle()"> Change color </button> <script> function changeStyle() { document.getElementById('square').style.backgroundColor = 'red'; document.getElementById('square').style.color = "white"; document.getElementById('square').style.fontSize = "25px"; document.getElementById('square').style.width = "700px"; } </script> </body> </html>
Changing Style of the Element When Event Triggers
You can also change the style of the element when a particular event triggers.
Example
In the below code, we added the 'click' event to the <div> element. When users click the button, it changes the background color of the div element.
<!DOCTYPE html> <html> <head> <style> div { width: 700px; height: 100px; color: white; background-color: orange; } </style> </head> <body> <div id = "square"> Click Me </div> <br> <script> const square = document.getElementById('square'); square.addEventListener('click', () => { square.style.backgroundColor = 'green'; square.style.fontSize = "25px"; }); </script> </body> </html>
Example
In the below code, we added the 'hover' event on the div element. When the mouse cursor enters and leaves the div element, it changes the style of the div element.
<!DOCTYPE html> <html> <head> <style> div { width: 700px; height: 100px; color: white; background-color: orange; } </style> </head> <body> <div id = "square"> Hover Me </div> <br> <script> const square = document.getElementById('square'); square.addEventListener('mouseenter', () => { square.style.backgroundColor = 'green'; square.style.fontSize = "25px"; }); square.addEventListener('mouseleave', () => { square.style.backgroundColor = 'orange'; square.style.fontSize = "initial"; }); </script> </body> </html>
Changing CSS of HTML elements Dynamically
You can also change the CSS of the HTML element dynamically. You can assign values using the variables.
Example
We have added the default style to the <div> element in the code below.
Also, we have created multiple radio buttons. When users select any radio button, it changes the style of the div element accordingly.
<!DOCTYPE html> <html> <head> <style> p { width: 700px; height: 100px; color: white; background-color: blue; } </style> </head> <body> <div><p id = "square">Select any of the following colors to change the background color</p></div> <div>Yellow: <input type = "radio" id = "yellow" name = "color"></div> <div>Green: <input type = "radio" id = "green" name = "color"></div> <div>Red: <input type = "radio" id = "red" name = "color"></div> <script> let square = document.getElementById('square'); // Changing the style when radio button changes let colors = document.getElementsByName('color'); for (let i = 0; i < colors.length; i++) { colors[i].addEventListener('change', function () { square.style.backgroundColor = this.id; }); } </script> </body> </html>