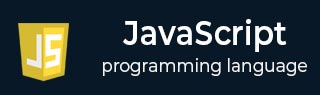
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript Date setUTCSeconds() Method
The JavaScript Date.setUTCSeconds() method is used to set the "seconds" of a Date object according to Coordinated Universal Time (UTC). The return value of this method will be an updated timestamp of the Date object, which reflects the changes made by modifying the seconds component. Additionally, we can modify the "milliseconds" of the date object.
UTC, also known as Universal Time Coordinated, is the time established by the World Time Standard. UTC is equivalent to GMT (Greenwich Mean Time), ensuring the uniformity in time measurement across the world.
Syntax
Following is the syntax of JavaScript Date setUTCSeconds() method −
setUTCSeconds(secondsValue, millisecondsValue);
Parameters
This method accepts two parameters. The same is described below −
- secondsValue An integer representing the seconds (0 to 59).
- If -1 is provided, it will result in the last second of the previous minute.
- If 60 is provided, it will result in the first second of the next minute.
- millisecondsValue (optional) An integer representing the milliseconds (0 to 999).
- If -1 is provided, it will result in the last millisecond of the previous second.
- If 1000 is provided, it will result in the first millisecond of the next second.
Return Value
This method returns the number of milliseconds between the generated date and midnight January 1, 1970, Universal Coordinated Time (UTC).
Example 1
In the following example, we are using the JavaScript Date setUTCSeconds() method to set the "seconds" to 30, according to UTC time −
<html> <body> <script> const currentDate = new Date(); currentDate.setUTCSeconds(30); document.write(currentDate)); </script> </body> </html>
Output
If we execute the above program, the seconds will be set to 30.
Example 2
If we provide "-1" for secondsValue, this method will gives the last second of the previous minute −
<html> <body> <script> const currentDate = new Date("2023-12-25 18:30:10"); currentDate.setUTCSeconds(-1); document.write(currentDate.getUTCSeconds()); </script> </body> </html>
Output
It will return "59" as the last second of previous minute (29).
Example 3
If we provide "60" for secondsValue, this method will gives the first second of the next minute −
<html> <body> <script> const currentDate = new Date("2023-12-25 18:30:10"); currentDate.setSeconds(60); document.write(currentDate.getUTCSeconds()); </script> </body> </html>
Output
It will return "0" as the last second of previous minute (31).
Example 4
In the following example, we are setting the "milliseconds" of a date object along with the "seconds" −
<html> <body> <script> const currentDate = new Date("2023-12-25 18:30:10"); currentDate.setSeconds(35, 697); document.write("UTCSeconds: ", currentDate.getUTCSeconds(), "
","UTCMilliseconds: ", currentDate.getMilliseconds()); </script> </body> </html>
Output
If we execute the above program, the seconds will be set to "35" and milliseconds to "697".
Example 5
If we pass a NaN value as a parameter to this function, the date will be set to "Invalid date" and "NaN" is returned as result −
<html> <body> <script> const currentDate = new Date(); currentDate.setUTCSeconds("Hle"); document.write(currentDate); </script> </body> </html>
Output
As we can see the output, "NaN" is returned as output.