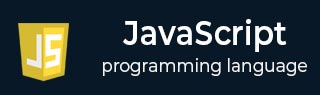
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - JSON.stringify() Method
The JavaScript JSON.stringify() method is used to convert a JavaScript value (such as an object or an array) into a JSON string. When using this method, you have the option to include an optional parameter called replacer, which can be specified as a function.
If you provide a replacer function, it allows you to modify the value being stringified. Additionally, by returning a different value from the replacer function, you can customize the output of the resulting JSON string.
The JSON.stringify() method is a static method in JavaScript, which means you can always use it as JSON.stringify() no need to create an object instance to invoke it.
Syntax
Following is the syntax of JavaScript JSON.stringify() method −
JSON.stringify(value, replacer, space)
Parameters
This method accepts three parameters named 'value', 'replacer', and 'space', which are described below −
value − The value you want to convert to a JSON string.
replacer (optional) − A function that modifies the stringification process or an array of strings and numbers specifying properties to include in the output. If the 'replacer' is an array, non-string and non-number elements (including symbols) are ignored. If 'replacer' is not a function or an array (e.g., null or not provided), all string-keyed properties of the object are included in the resulting JSON string.
space (optional) − A string or number is used to insert white space (indentation, line breaks, etc.) into the output JSON string for readability.
Return value
This method returns a JSON string representing the given value, or 'undefined'.
Examples
Example 1
If we pass only a value parameter to this method, it will convert this JavaScript value to a JSON string.
In the following example, we are using the JavaScript JSON.stringify() method to convert this JavaScript value {a: 10, b: 20, c: 30, d: 40, e: 50} to a JSON string.
<html> <head> <title>JavaScript JSON.stringify() Method</title> </head> <body> <script> const value = {a: 10, b: 20, c: 30, d: 40, e: 50}; document.write("The given value: ", value.a, value.b, value.c, value.d, value.e); document.write("<br>After converting to JSON srtring: ", JSON.stringify(value)); </script> </body> </html>
Output
The above program returns a JSON string after converting JavaScript value as −
The given value: 1020304050 After converting to JSON srtring: {"a":10,"b":20,"c":30,"d":40,"e":50}
Example 2
If we pass the value and space parameters to this method, it will convert the value to a JSON string and add a specified number of space of indentations before each character.
The following is another example of the JavaScript JSON.stringify() method. We use this method to convert the JavaScript value {"name": "Rahul", "age": 22, "city": "Lucknow"} to a formatted JSON string with 3, spaces of indentation for better readability.
const value = {"name": "Rahul", "age": 22, "city": "Lucknow"}; console.log("The given value: ", value.name, " ", value.age, " ", value.city); const space = 3; console.log("Number of spaces: ", space); //using the JSON.stringify() method const json_string = JSON.stringify(value, null, space); console.log(json_string);
Output
After executing the above program, it will return a JSON string in the console with 3, indentations (the changes only can be seen in the console).
The given value: Rahul 22 Lucknow Number of spaces: 3 { "name": "Rahul", "age": 22, "city": "Lucknow" }
Example 3
If we pass the replacer (as a function) as an argument to this method, it will modify the stringification process.
In the example below, we use the JSON.stringify() method to convert a JavaScript value to a JSON string. We define a replacer function named modifyAge(), which increases the age by 5 for each record. We pass this function as an argument to the method to modify the result before it is returned.
//custom replacer function function modifyAge(key, value){ if(key === "age"){ return value+5; } else { return value; } } const value = [{"name": "Rahul", "age": 22, "city": "Lucknow"}, {"name": "Vikash", "age": 20, "city": "Hyderabad"}]; console.log("The JavaScript value (before converting): ", JSON.stringify(value), " "); let space = 5; console.log("The number space: ", space); //using the JSON.stringify() method const jsonString = JSON.stringify(value, modifyAge, space); console.log("The JSON string (after converting): "); console.log(jsonString);
Output
Once the above program is executed, it will return a JSON string (with modified age).
The JavaScript value (before converting): [{"name":"Rahul","age":22,"city":"Lucknow"},{"name":"Vikash","age":20,"city":"Hyderabad"}] The number space: 5 The JSON string (after converting): [ { "name": "Rahul", "age": 27, "city": "Lucknow" }, { "name": "Vikash", "age": 25, "city": "Hyderabad" } ]
To Continue Learning Please Login