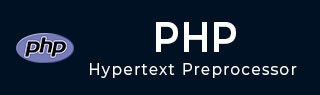
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Variables
A variable in PHP is a named memory location that holds data belonging to one of the data types.
PHP uses the convention of prefixing a dollar sign ($) to the name of a variable.
Variable names in PHP are case-sensitive.
Variable names follow the same rules as other labels in PHP. A valid variable name starts with a letter or underscore, followed by any number of letters, numbers, or underscores.
As per the naming convention, "$name", "$rate_of_int", "$Age", "$mark1" are examples of valid variable names in PHP.
Invalid variable names: "name" (not having $ prefix), "$rate of int" (whitespace not allowed), "$Age#1" (invalid character #), "$11" (name not starting with alphabet).
Variables are assigned with the "=" operator, with the variable on the left hand side and the expression to be evaluated on the right.
No Need to Specify the Type of a Variable
PHP is a dynamically typed language. There is no need to specify the type of a variable. On the contrary, the type of a variable is decided by the value assigned to it. The value of a variable is the value of its most recent assignment.
Take a look at this following example −
<?php $x = 10; echo "Data type of x: " . gettype($x) . "\n"; $x = 10.55; echo "Data type of x now: " . gettype($x) . ""; ?>
It will produce the following output −
Data type of x: integer Data type of x now: double
Automatic Type Conversion of Variables
PHP does a good job of automatically converting types from one to another when necessary. In the following code, PHP converts a string variable "y" to "int" to perform addition with another integer variable and print 30 as the result.
Take a look at this following example −
<?php $x = 10; $y = "20"; echo "x + y is: ", $x+$y; ?>
It will produce the following output −
x + y is: 30
Variables are Assigned by Value
In PHP, variables are always assigned by value. If an expression is assigned to a variable, the value of the original expression is copied into it. If the value of any of the variables in the expression changes after the assignment, it doesn’t have any effect on the assigned value.
<?php $x = 10; $y = 20; $z = $x+$y; echo "(before) z = ". $z . "\n"; $y=5; echo "(after) z = ". $z . ""; ?>
It will produce the following output −
(before) z = 30 (after) z = 30
Assigning Values to PHP Variables by Reference
You can also use the way to assign values to PHP variables by reference. In this case, the new variable simply references or becomes an alias for or points to the original variable. Changes to the new variable affect the original and vice versa.
To assign by reference, simply prepend an ampersand (&) to the beginning of the variable which is being assigned (the source variable).
Take a look at this following example −
<?php $x = 10; $y = &$x; $z = $x+$y; echo "x=". $x . " y=" . $y . " z = ". $z . "\n"; $y=20; $z = $x+$y; echo "x=". $x . " y=" . $y . " z = ". $z . ""; ?>
It will produce the following output −
x=10 y=10 z = 20 x=20 y=20 z = 40
Variable Scope
Scope can be defined as the range of availability a variable has to the program in which it is declared. PHP variables can be one of four scope types −
Variable Naming
Rules for naming a variable is −
Variable names must begin with a letter or underscore character.
A variable name can consist of numbers, letters, underscores but you cannot use characters like + , - , % , ( , ) . & , etc
There is no size limit for variables.