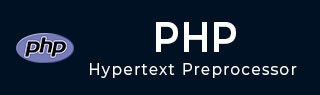
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP var_dump() Function
One of the built-in functions in PHP is the var_dump() function. This function displays structured information such as type and the value of one or more expressions given as arguments to this function.
var_dump(mixed $value, mixed ...$values): void
This function returns all the public, private and protected properties of the objects in the output. The dump information about arrays and objects is properly indented to show the recursive structure.
For the built-in integer, float and Boolean varibles, the var_dump() function shows the type and value of the argument variable.
Example 1
For example, here is an integer variable −
<?php $x = 10; var_dump ($x); ?>
The dump information is as follows −
int(10)
Example 2
Let's see how it behaves for a float variable −
<?php $x = 10.25; var_dump ($x); ?>
The var_dump() function returns the following output −
float(10.25)
Example 3
If the expression is a Boolean value −
<?php $x = true; var_dump ($x); ?>
It will produce the following output −
bool(true)
Example 4
For a string variable, the var_dump() function returns the length of the string also.
<?php $x = "Hello World"; var_dump ($x); ?>
It will produce the following output −
string(11) "Hello World"
Here we can use the <pre> HTML tag that dislays preformatted text. Text in a <pre> element is displayed in a fixed-width font, and the text preserves both the spaces and the line breaks.
<?php echo "<pre>"; $x = "Hello World"; var_dump ($x); echo "</pre>" ?>
It will produce the following output −
string(11) "Hello World"
Example 5 - Studying the Array Structure Using var_dump()
The var_dump() function is useful to study the array structure. In the following example, we have an array with one of the elements of the array being another array. In other words, we have a nested array situation.
<?php $x = array("Hello", false, 99.99, array(10, 20,30)); var_dump ($x); ?>
It will produce the following output −
array(4) { [0]=> string(5) "Hello" [1]=> bool(false) [2]=> float(99.99) [3]=> array(3) { [0]=> int(10) [1]=> int(20) [2]=> int(30) } }
Example 6
Since "$x" is an indexed array in the previous example, the index starting with "0" along with its value is dumped. In case the array is an associate array, the key-value pair information is dumped.
<?php $x = array( "Hello", false, 99.99, array(1=>10, 2=>20,3=>30) ); var_dump($x); ?>
Here, you will get the following output −
array(4) { [0]=> string(5) "Hello" [1]=> bool(false) [2]=> float(99.99) [3]=> array(3) { [1]=> int(10) [2]=> int(20) [3]=> int(30) } }
When you use var_dump() to show array value, there is no need of using the end tag " </pre> ".
Example 7
The var_dump() function can als reveal the properties of an object representing a class. In the following example, we have declared a Point class with two private properties "x" and "y". The class constructor initializes the object "p" with the parameters passed to it.
The var_dump() function provides the information about the object properties and their corrcponding values.
<?php class Point { private int $x; private int $y; public function __construct(int $x, int $y = 0) { $this->x = $x; $this->y = $y; } } $p = new Point(4, 5); var_dump($p) ?>
It will produce the following output −
object(Point)#1 (2) { ["x":"Point":private]=> int(4) ["y":"Point":private]=> int(5) }
There is a similar built-in function for producing dump in PHP, named as get_defined_vars().
var_dump(get_defined_vars());
It will dump all the defined variables to the browser.