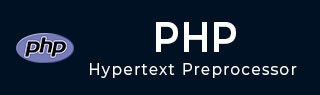
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Open File
PHP’s built-in function library provides fopen() function to open a file or any other stream and returns its "reference pointer", also called as "handle".
The fopen() function in PHP is similar to fopen() in C, except that in C, it cannot open a URL.
Syntax of fopen()
The fopen() function has the following signature −
fopen( string $filename, string $mode, bool $use_include_path = false, ?resource $context = null ): resource|false
The $filename and $mode parameters are mandatory. Here’s the explanation of the parameters −
$filename − This parameter is a string representing the resource to be opened. It may be a file in the local filesystem, or on a remote server with the scheme:// prefix.
$mode − A string that represents the type of access given to the file/resource.
$use_include_path − A Boolean optional parameter can be set to '1' or true if you want to search for the file in the include_path, too.
$context − A context stream resource.
Modes of Opening a File
PHP allows a file to be opened in the following modes −
Modes | Description |
---|---|
r | Open a file for read only. |
w | Open a file for write only. creates a new file even if it exists. |
a | Open a file in append mode |
x | Creates a new file for write only. |
r+ | Open a file for read/write. |
w+ | Open a file for read/write. creates a new file even if it exists. |
a+ | Open a file for read/write in append mode. |
x+ | Creates a new file for read/write. |
c | Open the file for writing, if it doesn’t exist. However, if it exists, it isn’t truncated (as in w mode). |
c++ | Open the file for read/write, if it doesn’t exist. However, if it exists, it isn’t truncated (as in w mode). |
e | Set close-on-exec flag on the opened file descriptor. Only available in PHP compiled on POSIX.1-2008 conform systems. |
If the fopen() function is successfully executed, it returns a "file pointer" or "handle" resource bound to the file stream. However, if it fails, it returns false with E_WARNING being emitted.
$handle = fopen('a.txt, 'r'); var_dump($handle);
If the file exists in the current directory, the success is shown by the output −
resource(5) of type (stream)
If not, you get the following error message −
Warning: fopen(a.txt): Failed to open stream: No such file or directory in a.php on line 2 bool(false)
Examples
The following examples show different usages of the fopen() function −
<?php $handle = fopen("hello.txt", "w"); $handle = fopen("c:/xampp/htdocs/welcome.png", "rb"); $handle = fopen("http://localhost/hello.txt", "r"); ?>
Note that this function may also succeed when the filename is a directory. In that case, you may need to use the is_dir() function to check whether it is a file before doing any read/write operations.
Once a file is opened, you can write data in it with the help of functions such as fwrite() or fputs(), and read data from it with fread() and fgets() functions.
Closing a File
It is always recommended to close the open stream referenced by the handle −
fclose($handle);