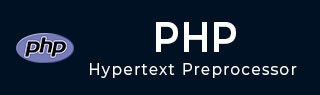
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - $_ENV
$_ENV is a superglobal variable in PHP. It is an associative array that stores all the environment variables available in the current script. $HTTP_ENV_VARS also contains the same information, but it is not a superglobal, and it has now been deprecated.
The environment variables are imported into the global namespace. Most of these variables are provided by the shell under which the PHP parser is running. Hence, the list of environment variables may be different on different platforms.
This array ($_ENV) also includes CGI variables in case PHP is running as a server module or a CGI processor.
We can use the foreach loop to display all the environment variables available −
<?php foreach ($_ENV as $k=>$v) echo $k . " => " . $v . "<br>"; ?>
On a Windows OS and with XAMPP server, you may get the list of environment variables as follows −
Variable |
Value |
---|---|
ALLUSERSPROFILE |
C:\ProgramData |
APPDATA |
C:\Users\user\AppData\Roaming |
CommonProgramFiles |
C:\Program Files\Common Files |
CommonProgramFiles(x86) |
C:\Program Files (x86)\Common Files |
CommonProgramW6432 |
C:\Program Files\Common Files |
COMPUTERNAME |
GNVBGL3 |
ComSpec |
C:\WINDOWS\system32\cmd.exe |
DriverData |
C:\Windows\System32\Drivers\DriverData |
HOMEDRIVE |
C − |
HOMEPATH |
\Users\user |
LOCALAPPDATA |
C:\Users\user\AppData\Local |
LOGONSERVER |
\\GNVBGL3 |
MOZ_PLUGIN_PATH |
C:\Program Files (x86)\ Foxit Software\ Foxit PDF Reader\plugins\ |
NUMBER_OF_PROCESSORS |
8 |
OneDrive |
C:\Users\user\OneDrive |
OneDriveConsumer |
C:\Users\user\OneDrive |
OS |
Windows_NT |
Path |
C:\Python311\Scripts\; C:\Python311\; C:\WINDOWS\system32; C:\WINDOWS; C:\WINDOWS\System32\Wbem; C:\WINDOWS\System32\WindowsPowerShell\ v1.0\; C:\WINDOWS\System32\OpenSSH\; C:\xampp\php; C:\Users\user\AppData\Local\Microsoft\ WindowsApps; C:\VSCode\Microsoft VS Code\bin |
PATHEXT |
.COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE; .WSF;.WSH;.MSC;.PY;.PYW |
PROCESSOR_ARCHITECTURE |
AMD64 |
PROCESSOR_IDENTIFIER |
Intel64 Family 6 Model 140 Stepping 1, GenuineIntel |
PROCESSOR_LEVEL |
6 |
PROCESSOR_REVISION |
8c01 |
ProgramData |
C:\ProgramData |
ProgramFiles |
C:\Program Files |
ProgramFiles(x86) |
C:\Program Files (x86) |
ProgramW6432 |
C:\Program Files |
PSModulePath |
C:\Program Files\WindowsPowerShell\Modules; C:\WINDOWS\system32\WindowsPowerShell\v1.0\ Modules |
PUBLIC |
C:\Users\Public |
SystemDrive |
C − |
SystemRoot |
C:\WINDOWS |
TEMP |
C:\Users\user\AppData\Local\Temp |
TMP |
C:\Users\user\AppData\Local\Temp |
USERDOMAIN |
GNVBGL3 |
USERDOMAIN_ROAMINGPROFILE |
GNVBGL3 |
USERNAME |
user |
USERPROFILE |
C:\Users\user |
windir |
C:\WINDOWS |
ZES_ENABLE_SYSMAN |
1 |
__COMPAT_LAYER |
RunAsAdmin Installer |
AP_PARENT_PID |
10608 |
You can access the value of individual environment variable too. This code fetches the PATH environment variable −
<?php echo "Path: " . $_ENV['Path']; ?>
It will produce the following output −
Path: C:\Python311\Scripts\;C:\Python311\;C:\WINDOWS\system32; C:\WINDOWS;C:\WINDOWS\System32\Wbem; C:\WINDOWS\System32\WindowsPowerShell\v1.0\; C:\WINDOWS\System32\OpenSSH\;C:\xampp\php; C:\Users\mlath\AppData\Local\Microsoft\WindowsApps; C:\VSCode\Microsoft VS Code\bin
Note − The $_ENV array may yield empty result, depending on "php.ini" setting "variables_order". You may have to edit the "php.ini" file and set variables_order="EGPCS" instead of variables_order="GPCS" value.
The getenv() Function
The PHP library provides the getenv() function to retrieve the list of all the environment variables or the value of a specific environment variable.
The following script displays the values of all the available environment variables −
<?php $arr=getenv(); foreach ($arr as $key=>$val) echo "$key=>$val"; ?>
To obtain the value of a specific variable, use its name as the argument for the getenv() function −
<?php echo "Path: " . getenv("PATH"); ?>
The putenv() Function
PHP also provides the putenv() function to create a new environment variable. The environment variable will only exist for the duration of the current request.
Changing the value of certain environment variables should be avoided. By default, users will only be able to set the environment variables that begin with "PHP_" (e.g. PHP_FOO=BAR).
The "safe_mode_protected_env_vars" directive in "php.ini" contains a comma-delimited list of environment variables that the end user won't be able to change using putenv().
<?php putenv("PHP_TEMPUSER=GUEST"); echo "Temp user: " . getenv("PHP_TEMPUSER"); ?>
The browser will display the following output −
Temp user: GUEST