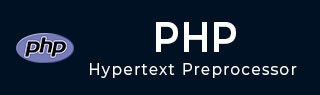
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP – Interfaces
Just as a class is a template for its objects, an interface in PHP can be called as a template for classes. We know that when a class is instantiated, the properties and methods defined in a class are available to it. Similarly, an interface in PHP declares the methods along with their arguments and return value. These methods do not have any body, i.e., no functionality is defined in the interface.
A concrete class has to implement the methods in the interface. In other words, when a class implements an interface, it must provide the functionality for all methods in the interface.
An interface is defined in the same way as a class is defined, except that the keyword "interface" is used in place of class.
interface myinterface { public function myfunction(int $arg1, int $arg2); public function mymethod(string $arg1, int $arg2); }
Note that the methods inside the interface have not been provided with any functionality. Definitions of these methods must be provided by the class that implements this interface.
When we define a child class, we use the keyword "extends". In this case, the class that must use the keyword "implements".
All the methods declared in the interface must be defined, with the same number and type of arguments and return value.
class myclass implements myinterface { public function myfunction(int $arg1, int $arg2) { ## implementation of myfunction; } public function mymethod(string $arg1, int $arg2) { # implementation of mymethod; } }
Note that all the methods declared in an interface must be public.
Example
Let us define an interface called shape. A shape has a certain area. You have shapes of different geometrical appearance, such as rectangle, circle etc., each having an area, calculated with different formula. Hence the shape interface declares a method area() that returns a float value.
interface shape { public function area(): float; }
Next, we shall define a circle class that implements shape interface, to implement, the class must provide a concrete implementation of the functions in the interface. Here, the area() function in circle class calculates the area of a circle of a given radius.
class circle implements shape { var $radius; public function __construct($arg1) { $this->radius = $arg1; } public function area(): float { return pow($this->radius,2)*pi(); } }
We can now declare an object of circle class, and call the area() method.
$cir = new circle(5); echo "Radius : " . $cir->radius . " Area of Circle: " . $cir->area(). PHP_EOL;
An interface can be implemented by any number of classes (which may be unrelated to each other) provided the implementing class provides functionality of each method in the interface.
Here is a Square class that implements shape. The area() method returns the square of the side property.
class square implements shape { var $side; public function __construct($arg1) { $this->side = $arg1; } public function area(): float { return pow($this->side, 2); } }
Similarly, create a Square object and call the area() method.
Example
Given below is the complete code for a shape interface, implemented by circle and Square classes −
<?php interface shape { public function area(): float; } class square implements shape { var $side; public function __construct($arg1) { $this->side = $arg1; } public function area(): float { return pow($this->side, 2); } } class circle implements shape { var $radius; public function __construct($arg1) { $this->radius = $arg1; } public function area(): float { return pow($this->radius,2)*pi(); } } $sq = new square(5); echo "Side: " . $sq->side . " Area of Square: ". $sq->area() . PHP_EOL; $cir = new circle(5); echo "Radius: " . $cir->radius . " Area of Circle: " . $cir->area(). PHP_EOL; ?>
It will produce the following output −
Side: 5 Area of Square: 25 Radius: 5 Area of Circle: 78.539816339745
Multiple Inheritance in PHP
PHP doesn’t have the provision to build a child class that extends two parent classes. In other words, the statement −
class child extends parent1, parent2
is not accepted. However, PHP does support having a child class that extends one parent class, and implementing one or more interfaces.
Let use look at the following example that shows a class that extends another and implements an interface.
First, the parent class marks. It has three instance variables or properties $m1, $m2, $m3 representing the marks in three subjects. A constructor is provided to initialize the object.
class marks { protected int $m1, $m2, $m3; public function __construct($x, $y, $z) { $this->m1 = $x; $this->m2 = $y; $this->m3 = $z; } }
We now provide an interface called percent that declares a method percent(), which should return a float but doesn’t have a function body.
interface percent { public function percent(): float; }
We now develop a class that extends marks class and provides implementation for percent() method in the interface.
class student extends marks implements percent { public function percent(): float { return ($this->m1+$this->m2+$this->m3)*100/300; } }
The student class inherits the parent constructor, but provides implementation of parent() method that returns the percentage of marks.
Example
The complete code is as follows −
<?php class marks { protected int $m1, $m2, $m3; public function __construct($x, $y, $z) { $this->m1 = $x; $this->m2 = $y; $this->m3 = $z; } } interface percent { public function percent(): float; } class student extends marks implements percent { public function percent(): float { return ($this->m1+$this->m2+$this->m3)*100/300; } } $s1 = new student(50, 60, 70); echo "Percentage of marks: ". $s1->percent() . PHP_EOL; ?>
It will produce the following output −
Percentage of marks: 60
The interface in PHP defines a framework of methods that classes use to provide a different but concrete implementation of their own.