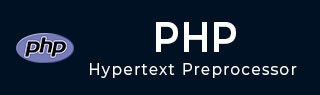
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
PHP - Read File
There are a number of options in PHP for reading data from a file that has been opened with the fopen() function. The following built-in functions in PHP’s library can help us perform the read operation −
fgets() − gets a line from the file pointer.
fgetc() − returns a string with a single character from the file pointer.
fread() − reads a specified number of bytes from the file pointer.
fscanf() − reads data from the file and parses it as per the specified format.
The fgets() Function
The fgets() function can return a line from an open file. This function stops returning on a new line at a specified length or EOF, whichever comes first and returns false on failure.
fgets(resource $stream, ?int $length = null): string|false
Here, the $stream parameter is a file pointer or handle to the file opened with the fopen() function with read or read/write mode, and $length is an optional parameter specifying the number of bytes to be read.
The read operation ends when "length-1" bytes are read or a newline is encountered, whichever is first.
Example
The following code reads the first available line from the "hello.txt" file −
<?php $file = fopen("hello.txt", "r"); $str = fgets($file); echo $str; fclose($file); ?>
It will produce the following output −
Hello World
Example
You can put the fgets() function in a loop to read the file until the end of file is reached.
<?php $file = fopen("hello.txt", "r"); while(! feof($file)) { echo fgets($file). "<br>"; } fclose($file); ?>
It will produce the following output −
Hello World TutorialsPoint PHP Tutorials
Here, we have used the feof() function which returns true if the file pointer is at EOF; otherwise returns false.
The fgetc() Function
The fgetc() function returns a single character read from the current position of the file handle. It returns false when EOF is encountered.
fgetc(resource $stream): string|false
Here, the $stream parameter is a file pointer or handle to the file opened with the fopen() function with read or read/write mode.
Example
The following code displays the first character read from the "hello.txt" file −
<?php $file = fopen("hello.txt", "r"); $str = fgets($file); echo $str; fclose($file); ?>
It will produce the following output −
H
Example
You can also put the fgetc() function inside a loop to read the file character by character until it reaches EOF.
<?php $file = fopen("hello.txt", "r"); while(! feof($file)) { $char = fgetc($file); if ($char == "\n") echo "<br>"; echo $char; } fclose($file); ?>
It will produce the following output −
Hello World TutorialsPoint PHP Tutorials
The fread() Function
The fread() function in PHP is a binary-safe function for reading data from a file. While the fgets() function reads only from a text file, the fread() function can read a file in binary mode.
fread(resource $stream, int $length): string|false
Here, the $stream parameter is a file pointer or handle to the file opened with the fopen() function with binary read or read/write mode (rb or rb+). The $length parameter specifies number of bytes to be read.
If the $length parameter is not given, PHP tries to read the entire file until EOF is reached, subject to the chunk size specified.
Example
The following code reads a text file −
<?php $name = "hello.txt"; $file = fopen($name, "r"); $data = fread($file, filesize($name)); echo $data; fclose($file); ?>
It will produce the following output −
Hello World TutorialsPoint PHP Tutorials
Example
You can also read a non-ASCII file such as an image file opened in rb mode.
<?php $name = "welcome.png"; $file = fopen($name, "rb"); $data = fread($file, filesize($name)); var_dump($data); fclose($file); ?>
The browser displays the "var_dump" information as the following −

The fscanf() Function
The fscanf() function in PHP reads the input from a file stream and parses it according to the specified format, thereby converts it into the variables of respective types. Each call to the function reads one line from the file.
fscanf(resource $stream, string $format, mixed &...$vars): array|int|false|null
Here, the $stream parameter is the handle to the file opened with the fopen() function and in read mode. And, $format is a string containing one or more of the following formatting specifiers −
%% − Returns a percent
%b − Binary number
%c − The character according to the ASCII value
%f − Floating-point number
%F − Floating-point number
%o − Octal number
%s − String
%d − Signed decimal number
%e − Scientific notation
%u − Unsigned decimal number
%x − Hexadecimal number for lowercase letters
%X − Hexadecimal number for uppercase letters
$vars is an optional parameter that specifies variables by reference which will contain the parsed values.
Assuming that the "employees.txt" file is available in the same directory in which the PHP script given below is present. Each line in the text file has name, email, post and salary of each employee, separated by tab character.
Example
The following PHP script reads the file using the format specifiers in fscanf() function −
<?php $fp = fopen("employees.txt", "r"); while ($employee_info = fscanf($fp, "%s\t%s\t%s\t%d\n")) { list ($name, $email, $post, $salary) = $employee_info; echo "<b>Name</b>: $name <b>Email</b>: $email <b>Salary</b>: Rs. $salary <br>"; } fclose($fp); ?>
It will produce the following output −
Name: Ravishankar Email: ravi@gmail.com Salary: Rs. 40000 Name: Kavita Email: kavita@hotmail.com Salary: Rs. 25000 Name: Nandkumar Email: nandu@example.com Salary: Rs. 30000