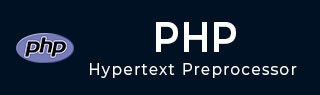
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Flash Messages
Message flashing in a PHP web application refers to the technique that makes certain messages popup on the browser window for the user to receive application’s feedback. To be able to give the user a meaningful feedback to his interactions is an important design principle, that gives a better user experience.
In a PHP web application, we can use the session data to flash messages regarding success or failure of a certain action, notifications or warnings, etc., from time to time to keep the user informed.
A flash message allows you to create a message on one page and display it once on another page. To transfer a message from one page to another, you use the $_SESSION superglobal variable.
To start with, you add a variable to the $_SESSION array as follows −
<?php session_start(); $_SESSION['flash_message'] = "Hello World"; ?>
Later, navigate to another page, and retrieve the flashed message from the $_SESSION variable and assign it to a variable. Then, you can display the message and then delete the message from the $_SESSION −
<?php session_start(); if(isset($_SESSION['flash_message'])) { $message = $_SESSION['flash_message']; unset($_SESSION['flash_message']); echo $message; } ?>
To generalize the basic idea of handling the flashed messages, we shall write a function that adds a message to the $_SESSION −
session_start(); function create_flash_message(string $name, string $message): void { // remove existing message with the name if (isset($_SESSION[FLASH][$name])) { unset($_SESSION[FLASH][$name]); } // add the message to the session $_SESSION[FLASH][$name] = ['message' => $message]; }
Let us also have another function that reads back a message, flashes it on the browser, and removes it from the $_SESSION.
function display_flash_message(string $name): void { if (!isset($_SESSION[FLASH][$name])) { return; } // get message from the session $flash_message = $_SESSION[FLASH][$name]; // delete the flash message unset($_SESSION[FLASH][$name]); // display the flash message echo format_flash_message($flash_message); }
The format_flash_message() function applies desired formatting to the obtained string with appropriate CSS rules.
If there are more than messages that have been flashed by the application, all of them can be retrieved and flashed with the following example −
function display_all_flash_messages(): void { if (!isset($_SESSION[FLASH])) { return; } // get flash messages $flash_messages = $_SESSION[FLASH]; // remove all the flash messages unset($_SESSION[FLASH]); // show all flash messages foreach ($flash_messages as $flash_message) { echo format_flash_message($flash_message); } }
Use the following flash() function to create, format and flash the messages
function flash(string $name = '', string $message = ''): void { if ($name !== '' && $message !== '') { create_flash_message($name, $message); } elseif ($name !== '' && $message === '') { display_flash_message($name); // display a flash message } elseif ($name === '' && $message === '' ) { display_all_flash_messages(); // display all flash message } }
To implement the above method, call the flash() function on the first page.
flash('first', 'Hello World');
Navigate to another page and call the flash() function to retrieve and display the message −
flash('first');
Mechanism of using the flash messages is usually employed on a signup page to redirect users to the login page with a welcome message after they sign up.