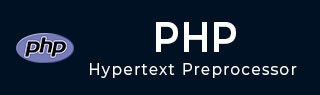
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP For C Developers
If you have a prior knowledge of C programming, learning PHP becomes a lot easier, especially the basics. Although PHP is a lot like C, it is bundled with a whole lot of Web-specific libraries, with everything hooked up directly to your favorite Web server.
The simplest way to think of PHP is as interpreted C that you can embed in HTML documents. PHP script can also be executed from the command line, much like a C program.
The syntax of statements and function definitions should be familiar, except that variables are always preceded by $, and functions do not require separate prototypes.
Let us take a look at some of the similarities and differences in PHP and C −
Similarities Between C and PHP
Syntax − Broadly speaking, PHP syntax is the same as in C, which is what makes learning PHP easier, if you are already conversant with C.Similar to C, PHP Code is blank insensitive, statements are terminated with semicolons.
function calls have the same structure
my_function(expression1, expression2) { Statements; }
Curly brackets are used to put multiple statements into blocks.
PHP supports C and C++-style comments (/* */ as well as //), and also Perl and shell-script style (#).
Operators − The assignment operators (=, +=, *=, and so on), the Boolean operators (&&, ||, !), the comparison operators (<,>, <=, >=, ==, !=), and the basic arithmetic operators (+, -, *, /, %) all behave in PHP as they do in C.
Control Structures − The basic control structures (if, switch, while, for) behave as they do in C, including supporting break and continue. One notable difference is that switch in PHP can accept strings as case identifiers.
PHP also has foreach looping construct that traverses the collections such as arrays.
Function Names − As you peruse the documentation, you.ll see many function names that seem identical to C functions.
Differences Between C and PHP
Dollar Sign − All variable names are prefixed with a leading $. Variables do not need to be declared in advance of assignment, and they have no intrinsic type. PHP is a dynamically typed language, as against C being a statically typed language.
Types − PHP has only two numerical types: integer (corresponding to a long in C) and double (corresponding to a double in C). In PHP, float is synonymous to double. Strings are of arbitrary length. There is no separate char type in PHP, as is the case in C.
Type Conversion − C is a strongly typed language, as type of a variable must be declared before using, and the types are checked at compile time. PHP on the other hand, is a weakly typed language, types are not checked at compile time, and type errors do not typically occur at runtime either. Instead, variables and values are automatically converted across types as needed.
Arrays − Arrays have a syntax superficially similar to C's array syntax, but they are implemented completely differently. In C, an array is a collection of similar data types. In a PHP array, the items may be of different types. PHP arrays are actually associative arrays or hashes, and the index can be either a number or a string. They do not need to be declared or allocated in advance.
No Struct Type − The struct keyword in C is used to define a new data type. There is no struct keyword or its equivalent in PHP, partly because the array and object types together make it unnecessary. The elements of a PHP array need not be of a consistent type.
No Pointers − Pointers are an important concept in C. There are no pointers available in PHP, although the tapeless variables play a similar role. Unlike C, PHP does support variable references. You can also emulate function pointers to some extent, in that function names can be stored in variables and called by using the variable rather than a literal name.
No Prototypes − Functions do not need to be declared before their implementation is defined, as long as the definition can be found somewhere in the current code file or included files. On the contrary, a C function must defined before it is used.
No main() − In a C program, the main() function is the entry point, irrespective of where it is present in the code. A PHP program on the other hand starts execution from the first statement in the script
Memory Management − The PHP engine is effectively a garbage-collected environment (reference-counted), and in small scripts there is no need to do any deallocation. You should freely allocate new structures - such as new strings and object instances. IN PHP5, it is possible to define destructor for objects, but there is are no free or delete keywords as in C/C++. Destructor are called when the last reference to an object goes away, before the memory is reclaimed.
Compilation and Linking − PHP is an interpreted language. Hence, the compiled version of PHP script is not created. A C program is first compiled to obtain the object code, which is then linked to the required libraries to build an executable. There is no separate compilation step for PHP scripts. A PHP script cannot be turned into a self executable.
Permissiveness − As a general matter, PHP is more forgiving than C (especially in its type system) and so will let you get away with new kinds of mistakes. Unexpected results are more common than errors.