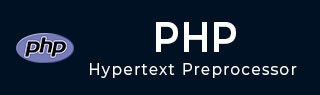
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - SAX Parser Example
PHP has the XML parser extension enabled by default in the php.ini settings file. This parser implements SAX API, which is an event-based parsing algorithm.
An event-based parser doesn’t load the entire XML document in the memory. instead, it reads in one node at a time. The parser allows you to interact with in real time. Once you move onto the next node, the old one is removed from the memory.
SAX based parsing mechanism is faster than the tree based parsers. PHP library includes functions to handle the XML events, as explained in this chapter.
The first step in parsing a XML document is to have a parser object, with xml_parse_create() function
xml_parser_create(?string $encoding = null): XMLParser
This function creates a new XML parser and returns an object of XMLParser to be used by the other XML functions.
The xml_parse() function starts parsing an XML document
xml_parse(XMLParser $parser, string $data, bool $is_final = false): int
xml_parse() parses an XML document. The handlers for the configured events are called as many times as necessary.
The XMLParser extension provides different event handler functions.
xml_set_element_handler()
This function sets the element handler functions for the XML parser. Element events are issued whenever the XML parser encounters start or end tags. There are separate handlers for start tags and end tags.
xml_set_element_handler(XMLParser $parser, callable $start_handler, callable $end_handler): true
The start_handler() function is called when a new XML element is opened. end_handler() function is called when an XML element is closed.
xml_set_character_data_handler()
This function sets the character data handler function for the XML parser parser. Character data is roughly all the non-markup contents of XML documents, including whitespace between tags.
xml_set_character_data_handler(XMLParser $parser, callable $handler): true
xml_set_processing_instruction_handler()
This function sets the processing instruction (PI) handler function for the XML parser parser. <?php ?> is a processing instruction, where php is called the "PI target". The handling of these are application-specific.
xml_set_processing_instruction_handler(XMLParser $parser, callable $handler): true
A processing instruction has the following format −
<?target data ?>
xml_set_default_handler()
This function sets the default handler function for the XML parser parser. What goes not to another handler goes to the default handler. You will get things like the XML and document type declarations in the default handler.
xml_set_default_handler(XMLParser $parser, callable $handler): true
Example
The following example demonstrates the use of SAX API for parsing the XML document. We shall use the SAX.xml as below −
<?xml version = "1.0" encoding = "utf-8"?> <tutors> <course> <name>Android</name> <country>India</country> <email>contact@tutorialspoint.com</email> <phone>123456789</phone> </course> <course> <name>Java</name> <country>India</country> <email>contact@tutorialspoint.com</email> <phone>123456789</phone> </course> <course> <name>HTML</name> <country>India</country> <email>contact@tutorialspoint.com</email> <phone>123456789</phone> </course> </tutors>
Example
The PHP code to parse the above document is given below. It opens the XML file and calls xml_parse() function till its end of file is reached. The event handlers store the data in tutors array. Then the array is echoed element wise.
<?php // Reading XML using the SAX(Simple API for XML) parser $tutors = array(); $elements = null; // Called to this function when tags are opened function startElements($parser, $name, $attrs) { global $tutors, $elements; if(!empty($name)) { if ($name == 'COURSE') { // creating an array to store information $tutors []= array(); } $elements = $name; } } // Called to this function when tags are closed function endElements($parser, $name) { global $elements; if(!empty($name)) { $elements = null; } } // Called on the text between the start and end of the tags function characterData($parser, $data) { global $tutors, $elements; if(!empty($data)) { if ($elements == 'NAME' || $elements == 'COUNTRY' || $elements == 'EMAIL' || $elements == 'PHONE') { $tutors[count($tutors)-1][$elements] = trim($data); } } } $parser = xml_parser_create(); xml_set_element_handler($parser, "startElements", "endElements"); xml_set_character_data_handler($parser, "characterData"); // open xml file if (!($handle = fopen('sax.xml', "r"))) { die("could not open XML input"); } while($data = fread($handle, 4096)) { xml_parse($parser, $data); } xml_parser_free($parser); $i = 1; foreach($tutors as $course) { echo "course No - ".$i. '<br/>'; echo "course Name - ".$course['NAME'].'<br/>'; echo "Country - ".$course['COUNTRY'].'<br/>'; echo "Email - ".$course['EMAIL'].'<br/>'; echo "Phone - ".$course['PHONE'].'<hr/>'; $i++; } ?>
The above code gives the following output −
course No - 1 course Name - Android Country - India Email - contact@tutorialspoint.com Phone - 123456789 ________________________________________ course No - 2 course Name - Java Country - India Email - contact@tutorialspoint.com Phone - 123456789 ________________________________________ course No - 3 course Name - HTML Country - India Email - contact@tutorialspoint.com Phone - 123456789 ________________________________________