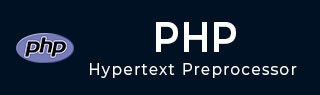
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Type Hints
PHP supports using "type hints" at the time of declaring variables in the function definition and properties or instance variables in a class. PHP is widely regarded as a weakly typed language. In PHP, you need not declare the type of a variable before assigning it any value.
The PHP parser tries to cast the variables into compatible type as far as possible. Hence, if one of values passed is a string representation of a number, and the second is a numeric variable, PHP casts the string variable to numeric in order to perform the addition operation.
Example
Take a look at the following example −
<?php function addition($x, $y) { echo "First number: $x Second number: $y Addition: " . $x+$y; } $x="10"; $y=20; addition($x, $y); ?>
It will produce the following output −
First number: 10 Second number: 20 Addition: 30
However, if $x in the above example is a string that doesn’t hold a valid numeric representation, then you would encounter an error.
<?php function addition($x, $y) { echo "First number: $x Second number: $y Addition: " . $x+$y; } $x="Hello"; $y=20; addition($x, $y); ?>
It will produce the following output −
PHP Fatal error: Uncaught TypeError: Unsupported operand types: string + int in hello.php:5
Type-hinting is supported from PHP 5.6 version onwards. It means you can explicitly state the expected type of a variable declared in your code. PHP allows you to type-hint function arguments, return values, and class properties. With this, it is possible to write more robust code.
Let us incorporate type-hinting in the addition function in the above program −
function addition($x, $y) { echo "First number: $x Second number: $y Addition: " . $x+$y; }
The type-hinting feature is mostly used by IDEs (Integrated Development Environment) to prompt the user about the expected types of the parameters used in function declaration.
The following figure shows the VS Code editor popping up the function prototype as you type −
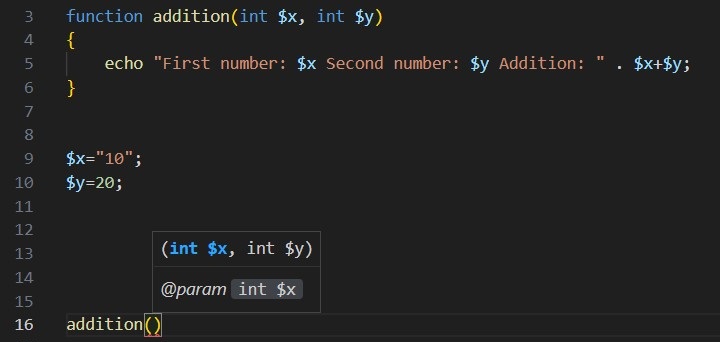
If the cursor hovers on the name of the function, the type declarations for the parameters and the return value are displayed −
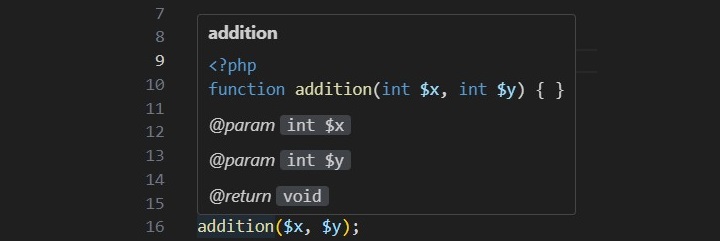
Note that by merely using the data types in the variable declarations doesn’t prevent the unmatched type exception raised, as PHP is a dynamically typed language. In other words, $x="10" and $y=20 will still result in the addition as 30, where as $x="Hello" makes the parser raise the error.
strict_types
PHP can be made to impose stricter rules for type conversion, so that "10" is not implicitly converted to 10. This can be enforced by setting strict_types directive to 1 in a declare() statement. The declare() statement must be the first statement in the PHP code, just after the "<?php" tag.
Example
<?php declare (strict_types=1); function addition(int $x, int $y) { echo "First number: $x Second number: $y Addition: " . $x+$y; } $x=10; $y=20; addition($x, $y); ?>
It will produce the following output −
First number: 10 Second number: 20 Addition: 30
Now, if $x is set to "10", the implicit conversion wont take place, resulting in the following error −
PHP Fatal error: Uncaught TypeError: addition(): Argument #1 ($x) must be of type int, string given
The VS Code IDE too indicates the error of the same effect −
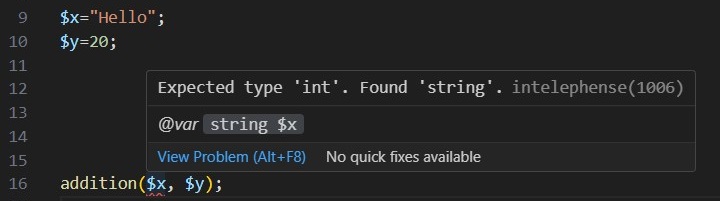
From PHP 7 onwards with type-hinting support has been extended for function returns to prevent unexpected return values. You can type-hint return values by adding the intended type after the parameter list prefixed with a colon (:) symbol.
Example
Let us add a type hint to the return value of the addition function above −
<?php declare (strict_types=1); function addition(int $x, int $y) : int { return $x+$y; } $x=10; $y=20; $result = addition($x, $y); echo "First number: $x Second number: $y Addition: " . $result; ?>
Here too, if the function is found to return anything other than an integer, the IDE indicates the reason even before you run.

Union Types
The PHP introduced union types with its version 8.0. You can now specify more than one type for a single declaration. The data types are separated by the "|" symbol.
Example
In the definition of addition() function below, the $x and $y arguments can be of int or float type.
<?php declare (strict_types=1); function addition(int|float $x, int|float $y) : float { return $x+$y; } $x=10.55; $y=20; $result = addition($x, $y); echo "First number: $x Second number: $y Addition: " . $result; ?>
Type-hinting in Class
In PHP, from version 7.4 onwards, you can use the type hints in declaration of class properties and methods.
Example
In the following example, the class constructor uses type hints −
<?php declare (strict_types=1); class Student { public $name; public $age; public function __construct(string $name, int $age) { $this->name = $name; $this->age = $age; } public function dispStudent() { echo "Name: $this->name Age: $this->age"; } } $s1 = new Student("Amar", 21); $s1->dispStudent(); ?>
It is also possible to use type hints in the declaration of class properties.
class Student { public string $name; public int $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function dispStudent() { echo "Name: $this->name Age: $this->age"; } }
The most commonly encountered errors during program development are type errors. The type-hinting feature helps in reducing them.