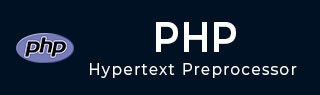
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Anonymous Functions
What are Anonymous Functions?
PHP allows defining anonymous functions. Normally, when we define a function in PHP, we usually provide it a name which is used to call the function whenever required. In contrast, an anonymous function is a function that doesn’t have any name specified at the time of definition. Such a function is also called closure or lambda function.
Sometimes, you may want a function for one time use only. The most common use of anonymous functions is to create an inline callback function.
Anonymous functions are implemented using the Closure class. Closure is an anonymous function that closes over the environment in which it is defined.
The syntax for defining an anonymous function is as follows −
$var=function ($arg1, $arg2) { return $val; };
Note that there is no function name between the function keyword and the opening parenthesis, and the fact that there is a semicolon after the function definition. This implies that anonymous function definitions are expressions. When assigned to a variable, the anonymous function can be called later using the variable’s name.
Example
Take a look at the following example −
<?php $add = function ($a, $b) { return "a:$a b:$b addition: " . $a+$b; }; echo $add(5,10); ?>
It will produce the following output −
a:5 b:10 addition: 15
Anonymous Function as a Callback
Anonymous functions are often used as callbacks. Callback functions are used as one of the arguments of another function. An anonymous function is executed on the fly and its return value becomes the argument of the parent function, which may be either a built-in or a user-defined function.
Example
In this example, we use an anonymous function inside the usort() function, a built in function that sorts an array by values using a user-defined comparison function.
<?php $arr = [10,3,70,21,54]; usort ($arr, function ($x , $y) { return $x > $y; }); foreach ($arr as $x){ echo $x . "\n"; } ?>
It will produce the following output −
3 10 21 54 70
Example
The following example uses an anonymous function to calculate the cumulative sum after successive numbers in an array. Here, we use the array_walk() function. This function applies a user defined function to each element in the array.
<?php $arr=array(1,2,3,4,5); array_walk($arr, function($n){ $s=0; for($i=1;$i<=$n;$i++){ $s+=$i; } echo "Number: $n Sum: $s". PHP_EOL; }); ?>
It will produce the following output −
Number: 1 Sum: 1 Number: 2 Sum: 3 Number: 3 Sum: 6 Number: 4 Sum: 10 Number: 5 Sum: 15
Anonymous Function as Closure
Closure is also an anonymous function that can access the variables outside its scope with the help of the "use" keyword.
Example
Take a look a the following example −
<?php $maxmarks=300; $percent=function ($marks) use ($maxmarks) { return $marks*100/$maxmarks; }; $m = 250; echo "Marks = $m Percentage = ". $percent($m); ?>
It will produce the following output −
Marks = 250 Percentage = 83.333333333333