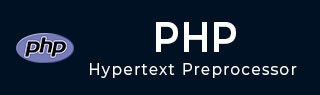
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Arrays
An array is a data structure that stores one or more data values having some relation among them, in a single variable. For example, if you want to store the marks of 10 students in a class, then instead of defining 10 different variables, it’s easy to define an array of 10 length.
Arrays in PHP behave a little differently than the arrays in C, as PHP is a dynamically typed language as against C which is a statically type language.
An array in PHP is an ordered map that associates values to keys.
A PHP array can be used to implement different data structures such as a stack, queue, list (vector), hash table, dictionary, etc.
The value part of an array element can be other arrays. This fact can be used to implement tree data structure and multidimensional arrays.
There are two ways to declare an array in PHP. One is to use the built-in array() function, and the other is to use a shorter syntax where the array elements are put inside square brackets.
The array() Function
The built-in array() function uses the parameters given to it and returns an object of array type. One or more comma-separated parameters are the elements in the array.
array(mixed ...$values): array
Each value in the parenthesis may be either a singular value (it may be a number, string, any object or even another array), or a key-value pair. The association between the key and its value is denoted by the "=>" symbol.
Examples
$arr1 = array(10, "asd", 1.55, true); $arr2 = array("one"=>1, "two"=>2, "three"=>3); $arr3 = array( array(10, 20, 30), array("Ten", "Twenty", "Thirty"), array("physics"=>70, "chemistry"=>80, "maths"=>90) );
Using Square Brackets [ ]
Instead of the array() function, the comma-separated array elements may also be put inside the square brackets to declare an array object. In this case too, the elements may be singular values or a string or another array.
$arr1 = [10, "asd", 1.55, true]; $arr2 = ["one"=>1, "two"=>2, "three"=>3]; $arr3 = [ [10, 20, 30], ["Ten", "Twenty", "Thirty"], ["physics"=>70, "chemistry"=>80, "maths"=>90] ];
Types of Arrays in PHP
There are three different kind of arrays and each array value is accessed using an ID which is called the array index.
Indexed Array − An array which is a collection of values only is called an indexed array. Each value is identified by a positional index staring from "0". Values are stored and accessed in linear fashion.
Associative Array − If the array is a collection of key-value pairs, it is called as an associative array. The key component of the pair can be a number or a string, whereas the value part can be of any type. Associative arrays store the element values in association with key values rather than in a strict linear index order.
Multi Dimensional Array − If each value in either an indexed array or an associative array is an array itself, it is called a multi dimensional array. Values are accessed using multiple indices
NOTE − Built-in array functions is given in function reference PHP Array Functions
It may be noted that PHP internally considers any of the above types as an associative array itself. In case of an indexed array, where each value has index, the index itself is its key. The var_dump() function reveals this fact.
Example
In this example, arr1 is an indexed array. However, var_dump()which displays the structured information of any object, shows that each value is having its index as its key.
<?php $arr1 = [10, "asd", 1.55, true]; var_dump($arr1); ?>
It will produce the following output −
array(4) { [0]=> int(10) [1]=> string(3) "asd" [2]=> float(1.55) [3]=> bool(true) }
Example
The same principle applies to a multi-dimensional index array, where each value in an array is another array.
<?php $arr1 = [ [10, 20, 30], ["Ten", "Twenty", "Thirty"], [1.1, 2.2, 3.3] ]; var_dump($arr1); ?>
It will produce the following output −
array(3) { [0]=> array(3) { [0]=> int(10) [1]=> int(20) [2]=> int(30) } [1]=> array(3) { [0]=> string(3) "Ten" [1]=> string(6) "Twenty" [2]=> string(6) "Thirty" } [2]=> array(3) { [0]=> float(1.1) [1]=> float(2.2) [2]=> float(3.3) } }
Accessing the Array Elements
To access any element from a given array, you can use the array[key] syntax.
Example
For an indexed array, put the index inside the square bracket, as the index itself is anyway the key.
<?php $arr1 = [10, 20, 30]; $arr2 = array("one"=>1, "two"=>2, "three"=>3); var_dump($arr1[1]); var_dump($arr2["two"]); ?>
It will produce the following output −
int(20) int(2)
We shall explore the types of PHP arrays in more details in the subsequent chapters.