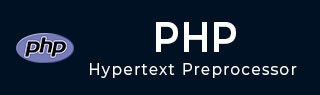
- PHP Tutorial
- PHP - Home
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Useful Resources
- PHP - Discussion
PHP - Append File
In PHP, the fopen() function returns the file pointer of a file used in different opening modes such as "w" for write mode, "r" read mode and "r+" or "r+" mode for simultaneous read/write operation, and "a" mode that stands for append mode.
When a file is opened with "w" mode parameter, it always opens a new file. It means that if the file already exists, its content will be lost. The subsequent fwrite() function will put the data at the starting position of the file.
Assuming that a file "new.txt" is present with the following contents −
Hello World TutorialsPoint PHP Tutorial
The following statement −
$fp = fopen("new.txt", "w");
Erases all the existing data before new contents are written.
Read/Write Mode
Obviously, it is not possible to add new data if the file is opened with "r" mode. However, "r+" or "w+" mod opens the file in "r/w" mode, but still a fwrite() statement immediately after opening a file will overwrite the contents.
Example
Take a look at the following code −
<?php $fp = fopen("new.txt", "r+"); fwrite($fp, "PHP-MySQL Tutorial\n"); fclose($fp); ?>
With this code, the contents of the "new.txt" file will now become −
PHP-MySQL Tutorial lsPoint PHP Tutorial
To ensure that the new content is added at the end of the existing file, we need to manually put the file pointer to the end, before write operation. (The initial file pointer position is at the 0th byte)
The fseek() Function
PHP’s fseek() function makes it possible to place the file pointer anywhere you want −
fseek(resource $stream, int $offset, int $whence = SEEK_SET): int
The $whence parameter is from where the offset is counted. Its values are −
SEEK_SET − Set position equal to offset bytes.
SEEK_CUR − Set position to current location plus offset.
SEEK_END − Set position to end-of-file plus offset.
Example
So, we need to move the pointer to the end with the fseek() function as in the following code which adds the new content to the end.
<?php $fp = fopen("new.txt", "r+"); fseek($fp, 0, SEEK_END); fwrite($fp, "\nPHP-MySQL Tutorial\n"); fclose($fp); ?>
Now check the contents of "new.txt". It will have the following text −
Hello World TutorialsPoint PHP Tutorial PHP-MySQL Tutorial
Append Mode
Instead of manually moving the pointer to the end, the "a" parameter in fopen() function opens the file in append mode. Each fwrite() statement adds the content at the end of the existing contents, by automatically moving the pointer to SEEK_END position.
<?php $fp = fopen("new.txt", "a"); fwrite($fp, "\nPHP-MySQL Tutorial\n"); fclose($fp); ?>
One of the allowed modes for fopen() function is "r+" mode, with which the file performs read/append operation. To read data from any position, you can place the pointer to the desired byte by fseek(). But, every fwrite() operation writes new content at the end only.
Example
In the program below, the file is opened in "a+" mode. To read the first line, we shift the file position to 0the position from beginning. However, the fwrite() statement still adds new content to the end and doesn’t overwrite the following line as it would have if the opening mode "r+" mode.
<?php $fp = fopen("new.txt", "a+"); fseek($fp, 0, SEEK_SET); $data = fread($fp, 12); echo $data; fwrite($fp, "PHP-File Handling"); fclose ($fp); ?>
Thus, we can append data to an existing file if it is opened in "r+/w+" mode or "a/a+" mode